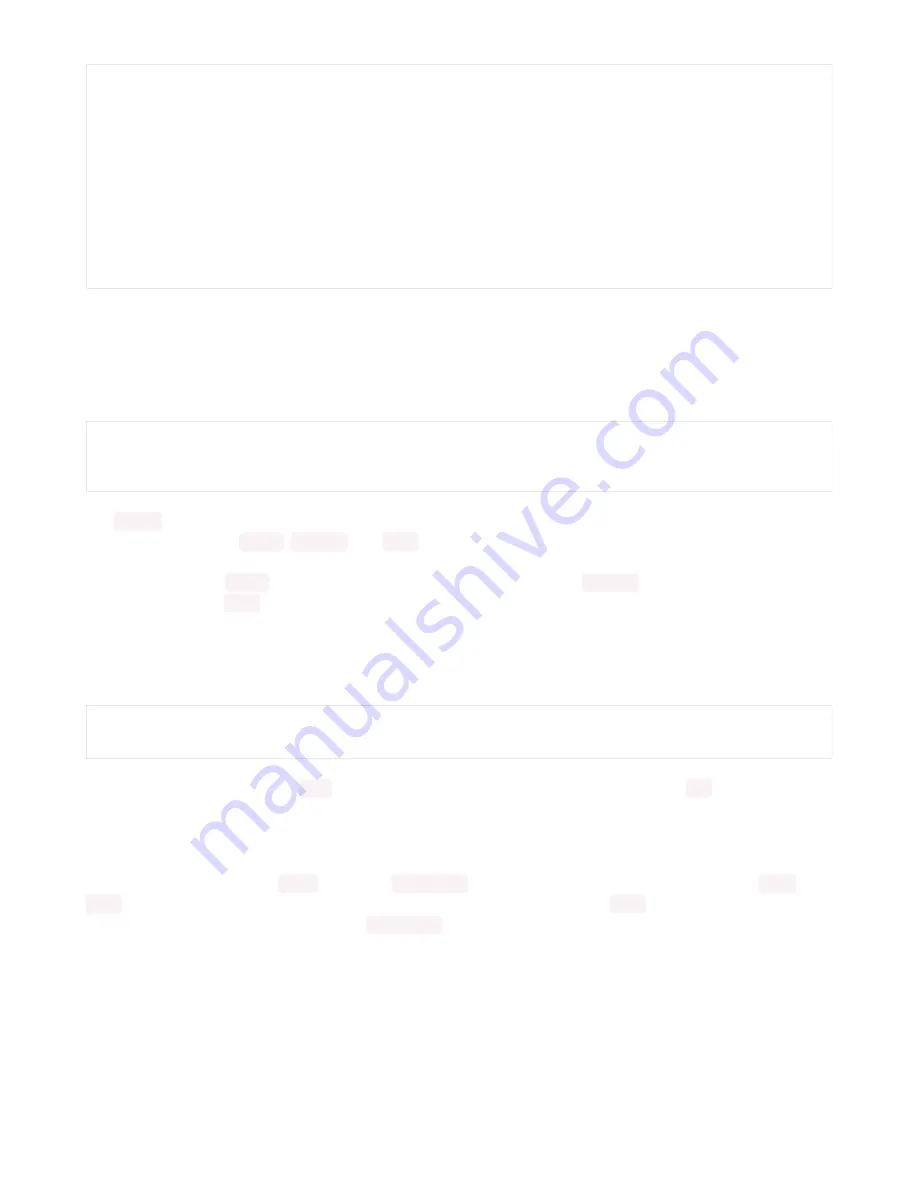
Imports & Libraries
Each CircuitPython program you run needs to have a lot of information to work. The reason CircuitPython is so simple
to use is that most of that information is stored in other files and works in the background. These files are called
libraries. Some of them are built into CircuitPython. Others are stored on your CIRCUITPY drive in a folder called lib.
The
import
statements tells the board that you're going to use a particular library in your code. In this example, we
imported three libraries:
board
,
digitalio
, and
time
. All three of these libraries are built into CircuitPython, so no
separate files are needed. That's one of the things that makes this an excellent first example. You don't need any thing
extra to make it work!
board
gives you access to the
hardware on your board
,
digitalio
lets you
access that hardware
as inputs/outputs
and
time
let's you pass time by 'sleeping'
Setting Up The LED
The next two lines setup the code to use the LED.
Your board knows the red LED as
D13
. So, we initialise that pin, and we set it to output. We set
led
to equal the rest
of that information so we don't have to type it all out again later in our code.
Loop-de-loops
The third section starts with a
while
statement.
while True:
essentially means, "forever do the following:".
while
True:
creates a loop. Code will loop "while" the condition is "true" (vs. false), and as
True
is never False, the code will
loop forever. All code that is indented under
while True:
is "inside" the loop.
Inside our loop, we have four items:
import board
import digitalio
import time
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
while True:
led.value = True
time.sleep(0.5)
led.value = False
time.sleep(0.5)
import board
import digitalio
import time
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 52 of 183
Содержание Feather M4 Express
Страница 1: ...Adafruit Feather M4 Express Created by lady ada Last updated on 2019 03 04 10 41 14 PM UTC...
Страница 5: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 10 of 183...
Страница 58: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 63 of 183...
Страница 164: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 169 of 183...
Страница 168: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 173 of 183...