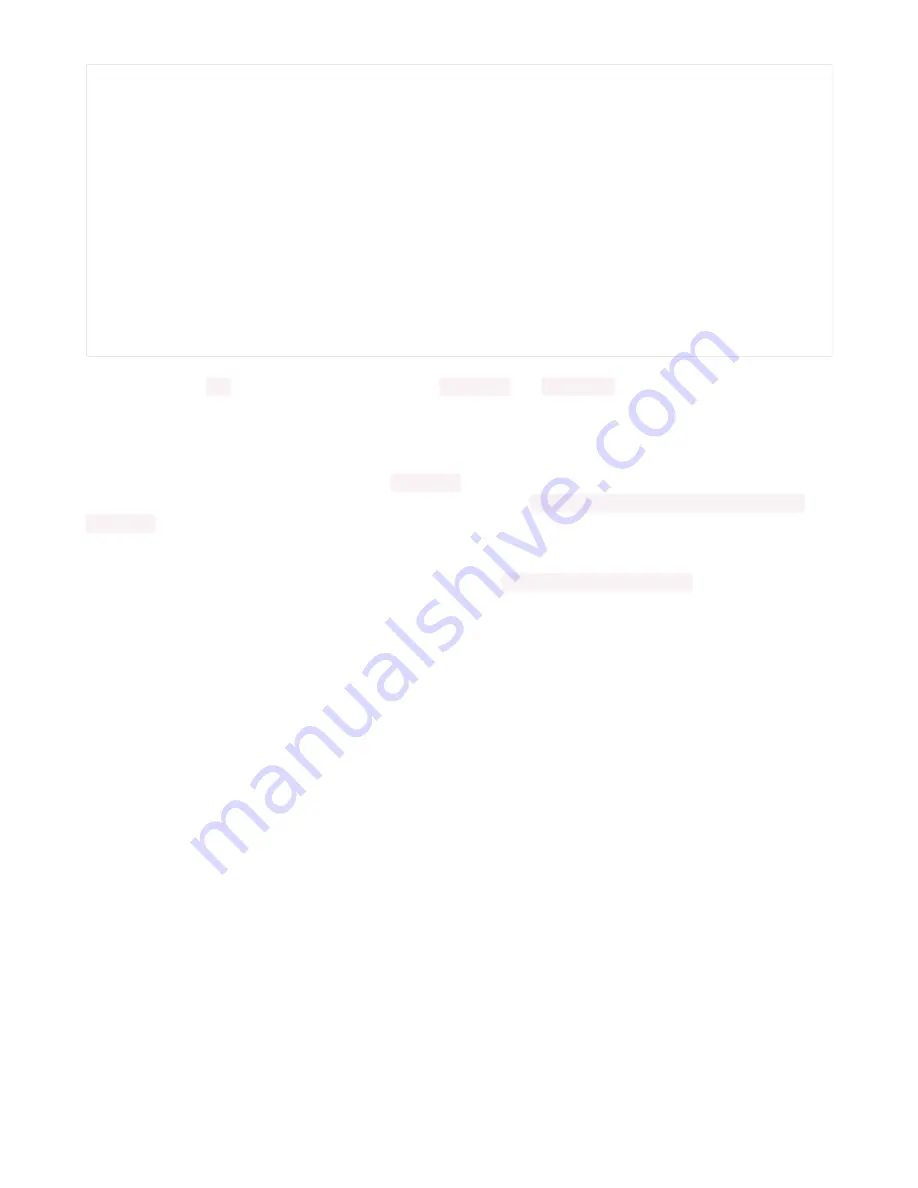
First we create the
i2c
object and provide the I2C pins,
board.SCL
and
board.SDA
.
To be able to scan it, we need to lock the I2C down so the only thing accessing it is the code. So next we include a
loop that waits until I2C is locked and then continues on to the scan function.
Last, we have the loop that runs the actual scan,
i2c_scan()
. Because I2C typically refers to addresses in hex form,
we've included this bit of code that formats the results into hex format:
[hex(device_address) for device_address in
i2c.scan()]
.
Open the serial console to see the results! The code prints out an array of addresses. We've connected the TSL2561
which has a 7-bit I2C address of 0x39. The result for this sensor is
I2C addresses found: ['0x39']
. If no addresses are
returned, refer back to the wiring diagrams to make sure you've wired up your sensor correctly.
I2C Sensor Data
Now we know for certain that our sensor is connected and ready to go. Let's find out how to get the data from our
sensor!
Copy and paste the code into code.py using your favorite editor, and save the file.
# CircuitPython demo - I2C scan
import time
import board
import busio
i2c = busio.I2C(board.SCL, board.SDA)
while not i2c.try_lock():
pass
while True:
print("I2C addresses found:", [hex(device_address)
for device_address in i2c.scan()])
time.sleep(2)
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 156 of 183
Содержание Feather M4 Express
Страница 1: ...Adafruit Feather M4 Express Created by lady ada Last updated on 2019 03 04 10 41 14 PM UTC...
Страница 5: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 10 of 183...
Страница 58: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 63 of 183...
Страница 164: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 169 of 183...
Страница 168: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 173 of 183...