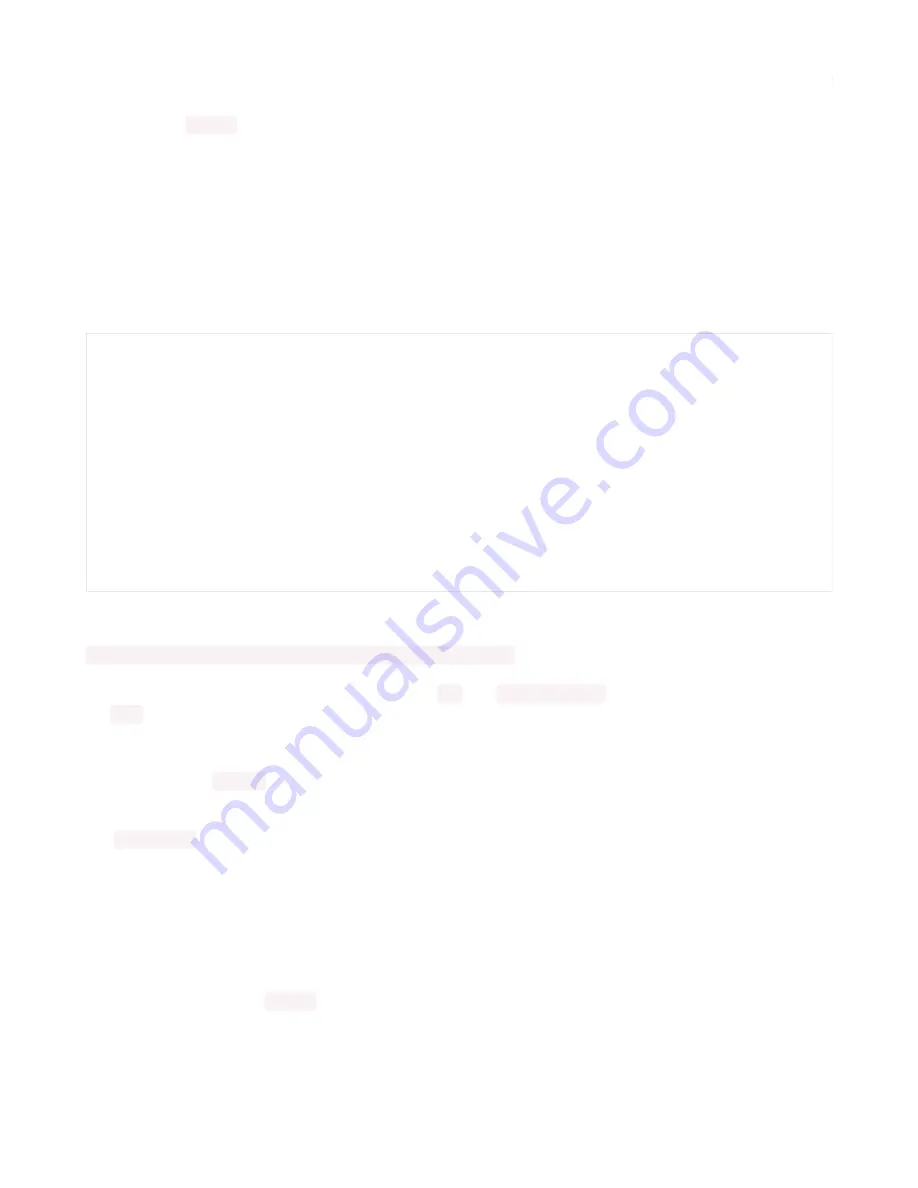
CircuitPython PWM
Your board has
pulseio
support, which means you can PWM LEDs, control servos, beep piezos, and manage "pulse
train" type devices like DHT22 and Infrared.
Nearly
every pin has PWM support! For example, all ATSAMD21 board have an A0 pin which is 'true' analog out and
does not
have PWM support.
PWM with Fixed Frequency
This example will show you how to use PWM to fade the little red LED on your board.
Copy and paste the code into code.py using your favorite editor, and save the file.
Create a PWM Output
led = pulseio.PWMOut(board.D13, frequency=5000, duty_cycle=0)
Since we're using the onboard LED, we'll call the object
led
, use
pulseio.PWMOut
to create the output and pass in
the
D13
LED pin to use.
Main Loop
The main loop uses
range()
to cycle through the loop. When the range is below 50, it PWMs the LED brightness up,
and when the range is above 50, it PWMs the brightness down. This is how it fades the LED brighter and dimmer!
The
time.sleep()
is needed to allow the PWM process to occur over a period of time. Otherwise it happens too quickly
for you to see!
PWM Output with Variable Frequency
Fixed frequency outputs are great for pulsing LEDs or controlling servos. But if you want to make some beeps with a
piezo, you'll need to vary the frequency.
The following example uses
pulseio
to make a series of tones on a piezo.
To use with any of the M0 boards, no changes to the following code are needed.
import time
import board
import pulseio
led = pulseio.PWMOut(board.D13, frequency=5000, duty_cycle=0)
while True:
for i in range(100):
# PWM LED up and down
if i < 50:
led.duty_cycle = int(i * 2 * 65535 / 100) # Up
else:
led.duty_cycle = 65535 - int((i - 50) * 2 * 65535 / 100) # Down
time.sleep(0.01)
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 119 of 183
Содержание Feather M4 Express
Страница 1: ...Adafruit Feather M4 Express Created by lady ada Last updated on 2019 03 04 10 41 14 PM UTC...
Страница 5: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 10 of 183...
Страница 58: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 63 of 183...
Страница 164: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 169 of 183...
Страница 168: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 173 of 183...