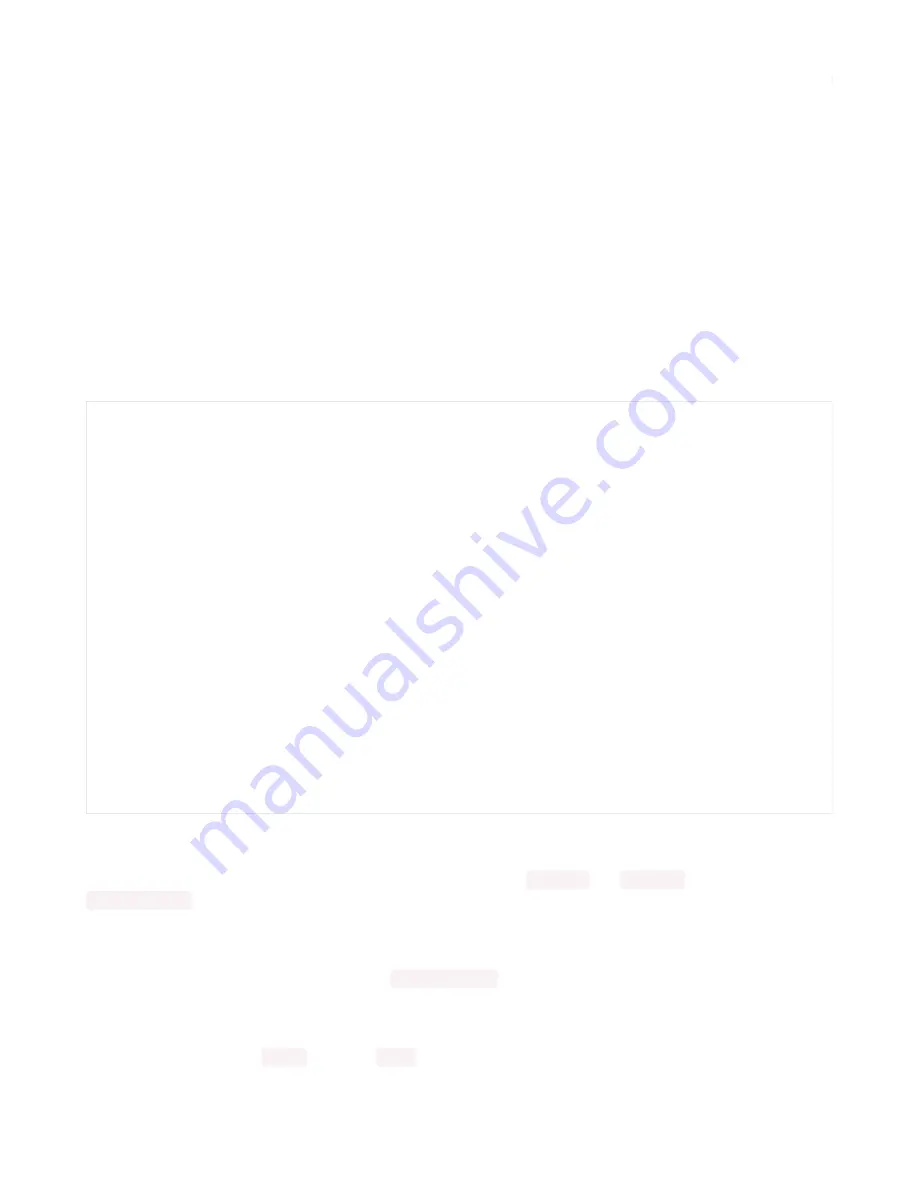
CircuitPython UART Serial
In addition to the USB-serial connection you use for the REPL, there is also a
hardware
UART you can use. This is
handy to talk to UART devices like GPSs, some sensors, or other microcontrollers!
This quick-start example shows how you can create a UART device for communicating with hardware serial devices.
To use this example, you'll need something to generate the UART data. We've used a GPS! Note that the GPS will give
you UART data without getting a fix on your location. You can use this example right from your desk! You'll have data
to read, it simply won't include your actual location.
You'll need the adafruit_bus_device library folder if you don't already have it in your /lib folder! You can get it from the
CircuitPython Library Bundle
(https://adafru.it/y8E)
. If you need help installing the library, check out the
CircuitPython
Libraries page
(https://adafru.it/ABU)
.
Copy and paste the code into code.py using your favorite editor, and save the file.
The Code
First we create the UART object. We provide the pins we'd like to use,
board.TX
and
board.RX
, and we set the
baudrate=9600
. While these pins are labeled on most of the boards, be aware that RX and TX are not labeled on
Gemma, and are labeled on the bottom of Trinket. See the diagrams below for help with finding the correct pins on
your board.
Once the object is created you read data in with
read(
numbytes
)
where you can specify the max number of bytes. It
will return a byte array type object if anything was received already. Note it will always return immediately because
there is an internal buffer! So read as much data as you can 'digest'.
If there is no data available,
read()
will return
None
, so check for that before continuing.
# CircuitPython Demo - USB/Serial echo
import board
import busio
import digitalio
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
uart = busio.UART(board.TX, board.RX, baudrate=9600)
while True:
data = uart.read(32) # read up to 32 bytes
# print(data) # this is a bytearray type
if data is not None:
led.value = True
# convert bytearray to string
data_string = ''.join([chr(b) for b in data])
print(data_string, end="")
led.value = False
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 145 of 183
Содержание Feather M4 Express
Страница 1: ...Adafruit Feather M4 Express Created by lady ada Last updated on 2019 03 04 10 41 14 PM UTC...
Страница 5: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 10 of 183...
Страница 58: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 63 of 183...
Страница 164: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 169 of 183...
Страница 168: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 173 of 183...