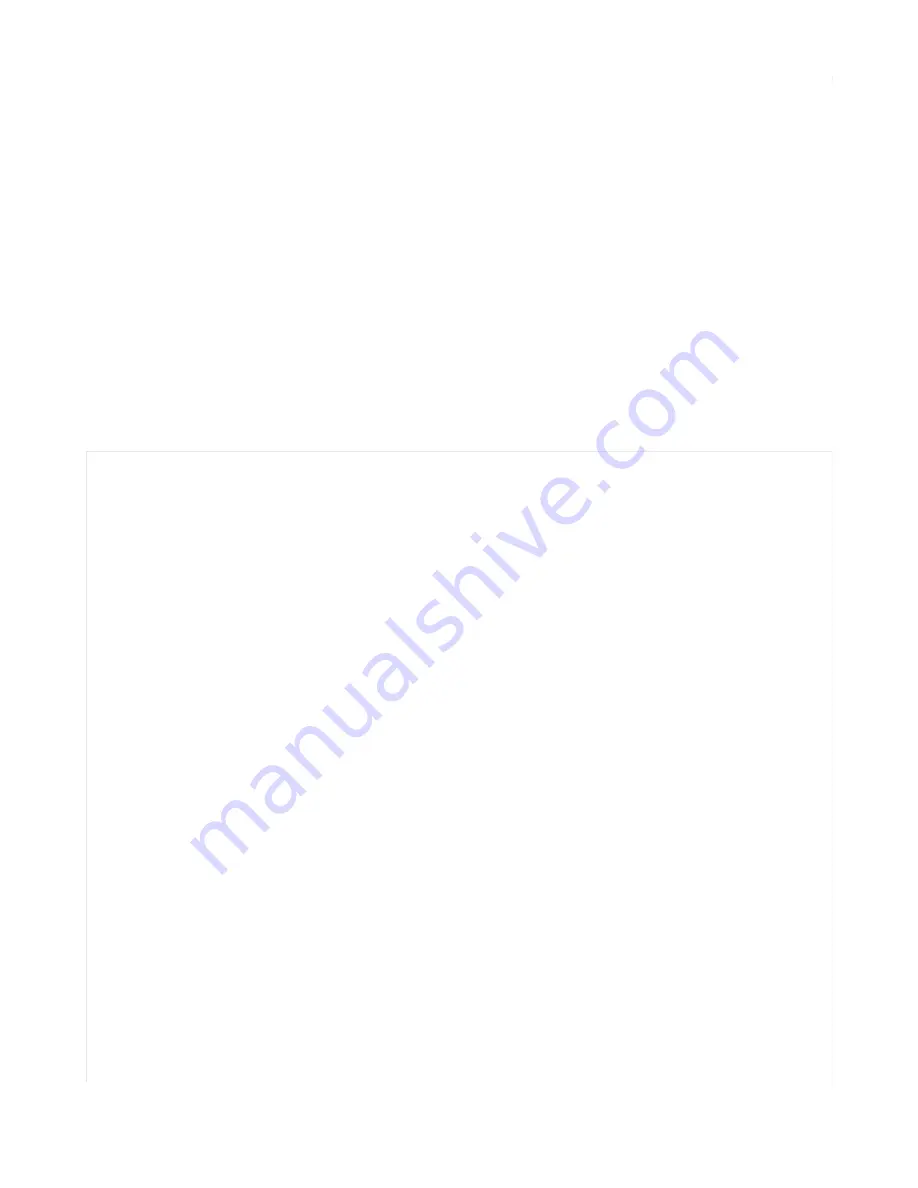
CircuitPython HID Keyboard and Mouse
One of the things we baked into CircuitPython is 'HID' (Human Interface Device) control - that means keyboard and
mouse capabilities. This means your CircuitPython board can act like a keyboard device and press key commands, or a
mouse and have it move the mouse pointer around and press buttons. This is really handy because even if you cannot
adapt your software to work with hardware, there's almost always a keyboard interface - so if you want to have a
capacitive touch interface for a game, say, then keyboard emulation can often get you going really fast!
This section walks you through the code to create a keyboard or mouse emulator. First we'll go through an example
that uses pins on your board to emulate keyboard input. Then, we will show you how to wire up a joystick to act as a
mouse, and cover the code needed to make that happen.
You'll need the adafruit_hid library folder if you don't already have it in your /lib folder! You can get it from the
CircuitPython Library Bundle
(https://adafru.it/y8E)
. If you need help installing the library, check out the
CircuitPython
Libraries page
(https://adafru.it/ABU)
.
CircuitPython Keyboard Emulator
Copy and paste the code into code.py using your favorite editor, and save the file.
# CircuitPython demo - Keyboard emulator
import time
import board
import digitalio
from adafruit_hid.keyboard import Keyboard
from adafruit_hid.keyboard_layout_us import KeyboardLayoutUS
from adafruit_hid.keycode import Keycode
# A simple neat keyboard demo in CircuitPython
# The pins we'll use, each will have an internal pullup
keypress_pins = [board.A1, board.A2]
# Our array of key objects
key_pin_array = []
# The Keycode sent for each button, will be paired with a control key
keys_pressed = [Keycode.A, "Hello World!\n"]
control_key = Keycode.SHIFT
# The keyboard object!
time.sleep(1) # Sleep for a bit to avoid a race condition on some systems
keyboard = Keyboard()
keyboard_layout = KeyboardLayoutUS(keyboard) # We're in the US :)
# Make all pin objects inputs with pullups
for pin in keypress_pins:
key_pin = digitalio.DigitalInOut(pin)
key_pin.direction = digitalio.Direction.INPUT
key_pin.pull = digitalio.Pull.UP
key_pin_array.append(key_pin)
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
print("Waiting for key pin...")
© Adafruit Industries
https://learn.adafruit.com/adafruit-feather-m4-express-atsamd51
Page 160 of 183
Содержание Feather M4 Express
Страница 1: ...Adafruit Feather M4 Express Created by lady ada Last updated on 2019 03 04 10 41 14 PM UTC...
Страница 5: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 10 of 183...
Страница 58: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 63 of 183...
Страница 164: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 169 of 183...
Страница 168: ...Adafruit Industries https learn adafruit com adafruit feather m4 express atsamd51 Page 173 of 183...