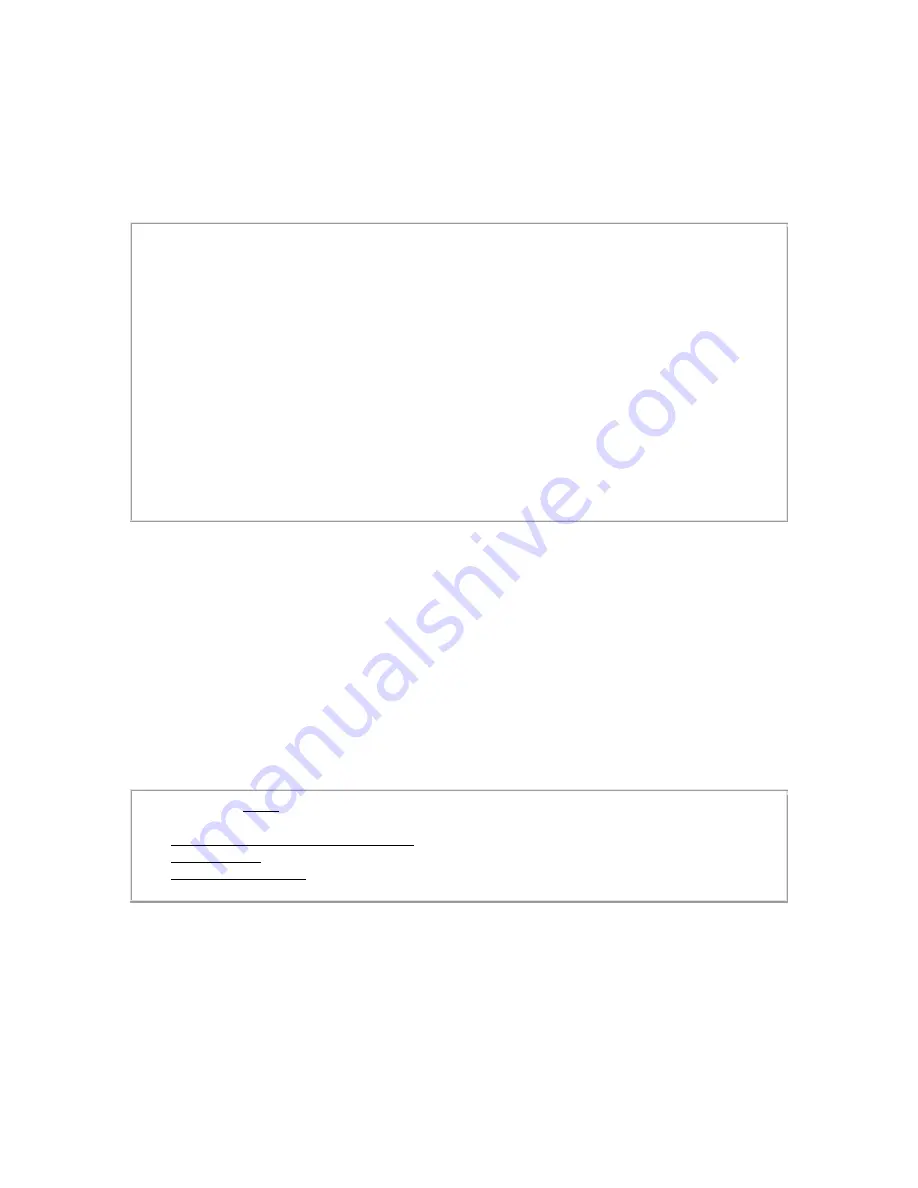
35
The following example shows a typical action body. This action is named "BTN_1_PRESS". It
has two local variables. The variable "count" is a static variable that will be incremented each
time the action is executed. After the count is incremented a message string is built up with the
count included and the message is printed to the console (a Telnet window).
// ---------------------------------------------------------
// This action will print the messages:
// SVM: This action has been executed 1 times.
// SVM: This action has been executed 2 times.
// SVM: This action has been executed 3 times.
// SVM: This action has been executed 4 times.
// etc ...
// ---------------------------------------------------------
action: BTN_1_PRESS
{
static variable: count = 0
variable: message
count = count + 1
message = "This action has been executed " # count # " times."
print (message)
}
9.7 - Action Parameters
When an action is executed a set of four parameters will be passed to the action. All four
parameters are not always used. If a particular action type does not use all four parameters, the
unused parameters will contain empty strings.
The meaning of the parameters is specified by the source of the action, see the section action
types. Action parameters are accessed by the built-in variable names "$1", "$2", "$3" and "$4".
9.8 - Subroutine Bodies
A subroutine declaration begins with the keyword "subroutine:" followed by the subroutine name,
then an open curly brace. Within the subroutine body are any number of statements. The end of a
subroutine is indicated by a closing curly brace. The following example shows the structure of a
subroutine body.
subroutine: name
{
local variable declarations
statements
optional return
}
9.9 - Subroutine Parameters
When a subroutine is executed a set of four parameters will be passed to the subroutine. All four
parameters are not always used. If a particular action type does not use all four parameters, the
unused parameters will contain empty strings.
Subroutine input parameters are accessed by the built-in variable names "$1", "$2", "$3" and
"$4". The following example shows the use of parameters within subroutines.