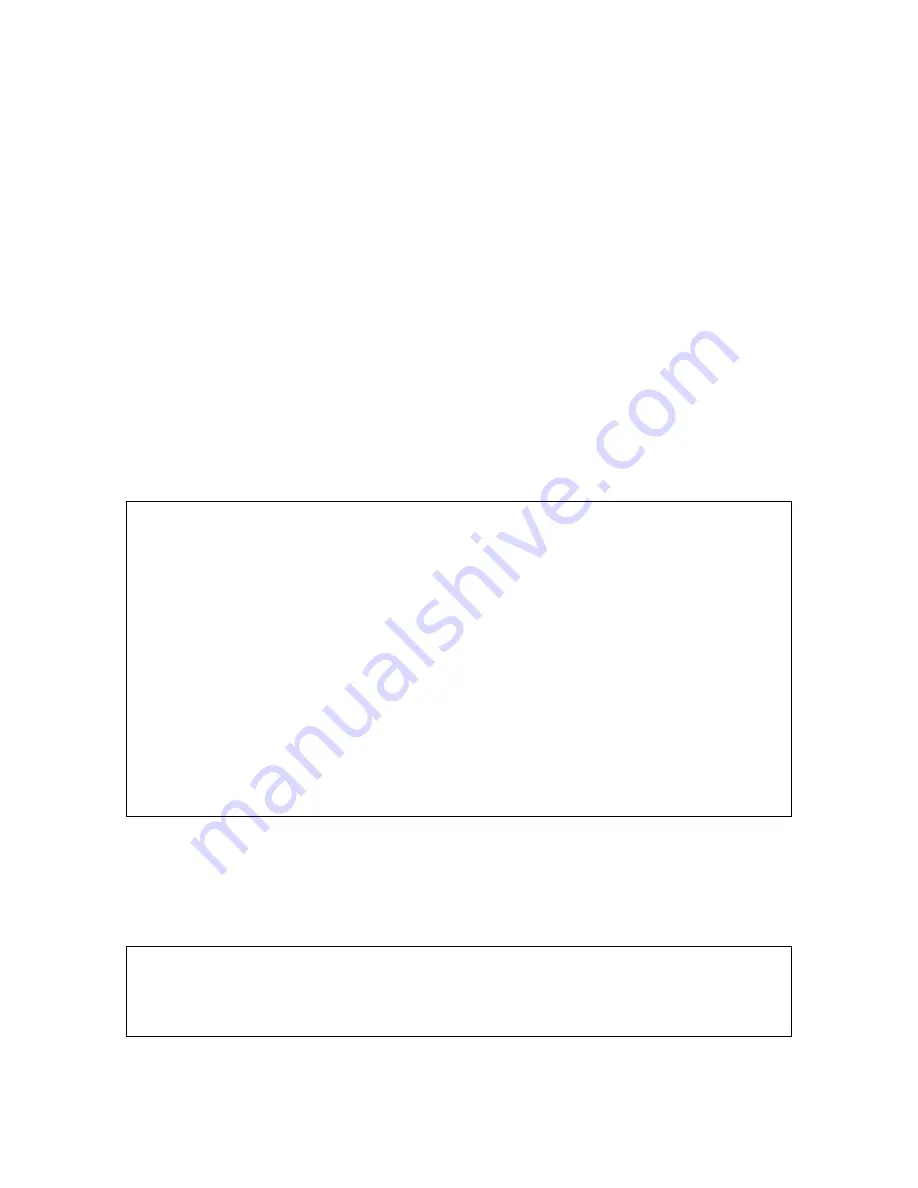
27
7.7 - Example Script – Subroutines
The example script uses two subroutines – one to handle the switch presses and one to store the
last switch pressed so it’s LED can be turned OFF on a subsequent switch press. Note that a
custom startup routine was not included. Try writing a startup subroutine that figures out which
source is currently feeding “dest_a” and then light the appropriate button’s LED.
The first subroutine – handle_sw_press - is called by the Button Actions defined at the end of
the Script. Button Actions “pass” two variables, $1 and $2 to this subroutine.
This subroutine:
•
Modifies the value of “switch” to equal $1 and “source” to equal $2.
•
Turns OFF the previously selected switch’s LED.
•
Calls subroutine to store the currently selected switch number.
•
Connects the currently selected source.
•
Lights the LED in the currently selected switch.
This subroutine includes a “Print” statement to print a message to a Telnet window –please see
the Script de-bugging section for details on using Print and Telnet.
//***********************
// Subroutines
//***********************
subroutine: handle_sw_press //This subroutine does most of the work.
//It receives switch# and source info from the button
//press actions.
{
switch = $1 // $1(reads “string one”) is the switch number passed here when subroutine called by
// action.
source = $2 // $2
btn_led (last_led, OFF)
call store_switch (switch)
connect (dest_a, source) //dest_a is a fixed destination defined above as a constant
btn_led (switch, ON)
print ("connecting Source ID " # source # " to Dest " # dest_a # "." )
}
The second subroutine simply receives a variable value, “switch”, and stores it. Note that this
could have been done in the “handle_sw_press” subroutine, but as an exercise this illustrates
variable passing and subroutine nesting. Notice that the variable “current_switch” was never used
in the script.
subroutine: store_switch //
{
current_switch = $1 // string 1 passed here = value of the “switch” variable in the calling subroutine.
last_led = $1 // the “last led” variable is set to = the “switch” variable.
}