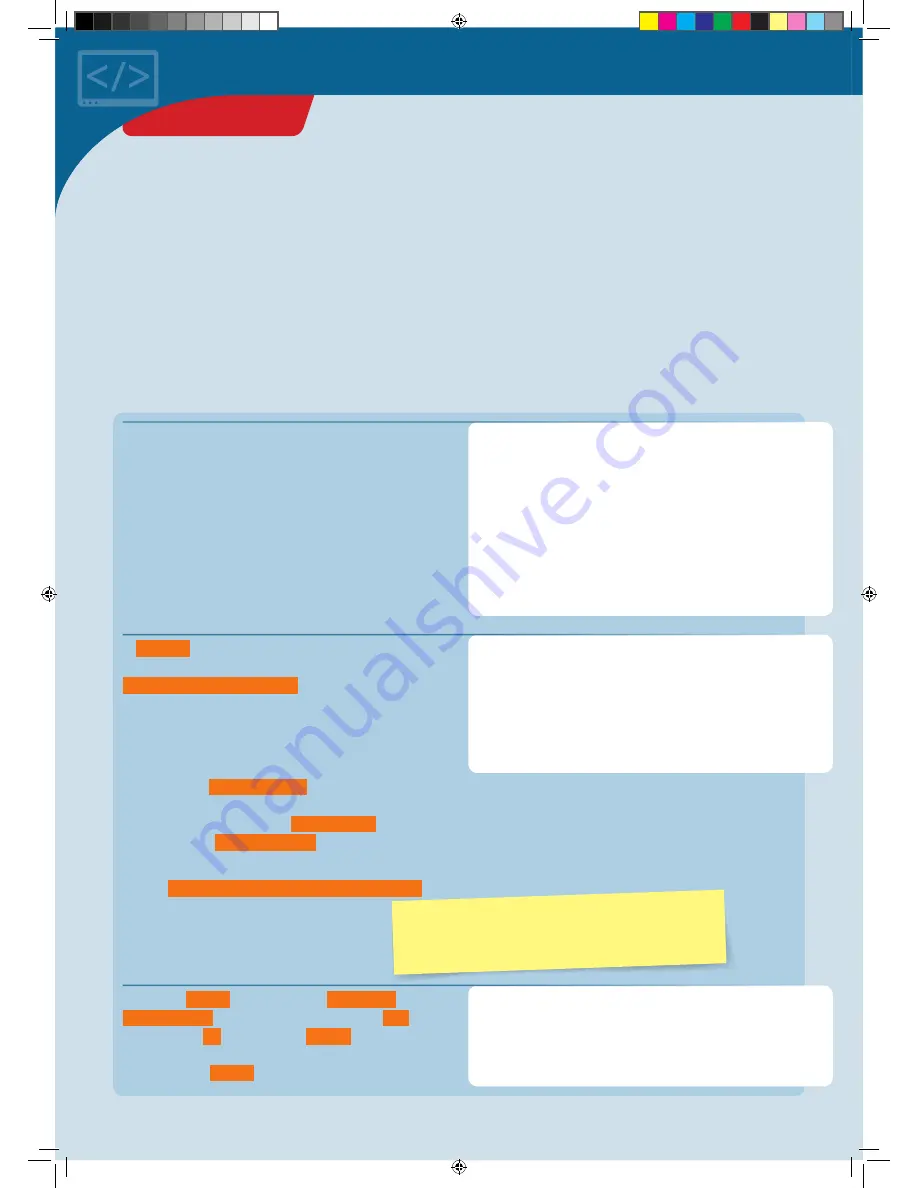
A blinking die
With this project, you will be turning your KosmoDuino
into a simple die. At the push of a button, it will generate a
random number from 1 to 6 and signal the result by
blinking the NeoPixel.
YOU WILL NEED
› KosmoDuino in the interaction board
#include <KosmoBits_Pins.h>
#include <Adafruit_NeoPixel.h>
#include <KosmoBits_Pixel.h>
KosmoBits_Pixel pixel;
const int buttonPin = KOSMOBITS_BUTTON_1_PIN;
const int blinkDuration = 500;
// Milliseconds
const int blinkPause = 250;
// Milliseconds
const int brightness = 50;
In
setup()
, you prepare your controller for the upcoming
tasks in the usual manner: Use
pinMode(buttonPin, INPUT)
to set the pin connected to
button 1 as the input pin.
Later, you will be generating a random number. This will
be handled by a random generator, which will need as
random a value as possible to start with. To get that, read
out pin 12 with
analogRead(12)
. Since nothing is
connected there, any values that you get will be random.
You will learn more about the
analogRead()
function a
little later. Then
randomSeed(...)
delivers the read value
to the random generator itself as a starting value.
Finally,
pixel.setColor(0, 0, 255, brightness);
makes the NeoPixel glow blue.
void setup() {
pinMode(buttonPin, INPUT);
randomSeed(analogRead(12));
// Starting value
// for random generator
pixel.setColor(0, 0, 255, brightness);
// Blue
// signals operational readiness
}
void loop() {
if (digitalRead(buttonPin) == LOW) {
roll();
}
}
You start by including the familiar libraries and add a
KosmoBits_Pixel to address the NeoPixel on the
interaction board.
Then, you add a few constants to make the code legible.
THE PLAN:
• If the controller is ready, the NeoPixel will glow blue.
• If you press button 1, the NeoPixel will begin to blink
green in accordance with the die result, i.e., once if the
rolled result was one, twice if it was two, etc.
• After a brief pause, the NeoPixel will return to blue. That
means that it’s ready to roll again.
In the main
loop()
, you read out the
buttonPin
with
digitalRead()
. When it is pushed, the result is
LOW
. With
the help of the
if
instruction the
roll()
function is
invoked, which calculates and outputs the result of the die
roll. Otherwise,
loop()
does nothing.
</>
THE PROGRAM
This is a shortened version of the notatio
n that you
learned in Project 4. The numbers in the b
rackets control
the RGB value (red/green/blue).
26
PROJECT 6
CodeGamer manual inside english.indd 26
7/19/16 12:32 PM