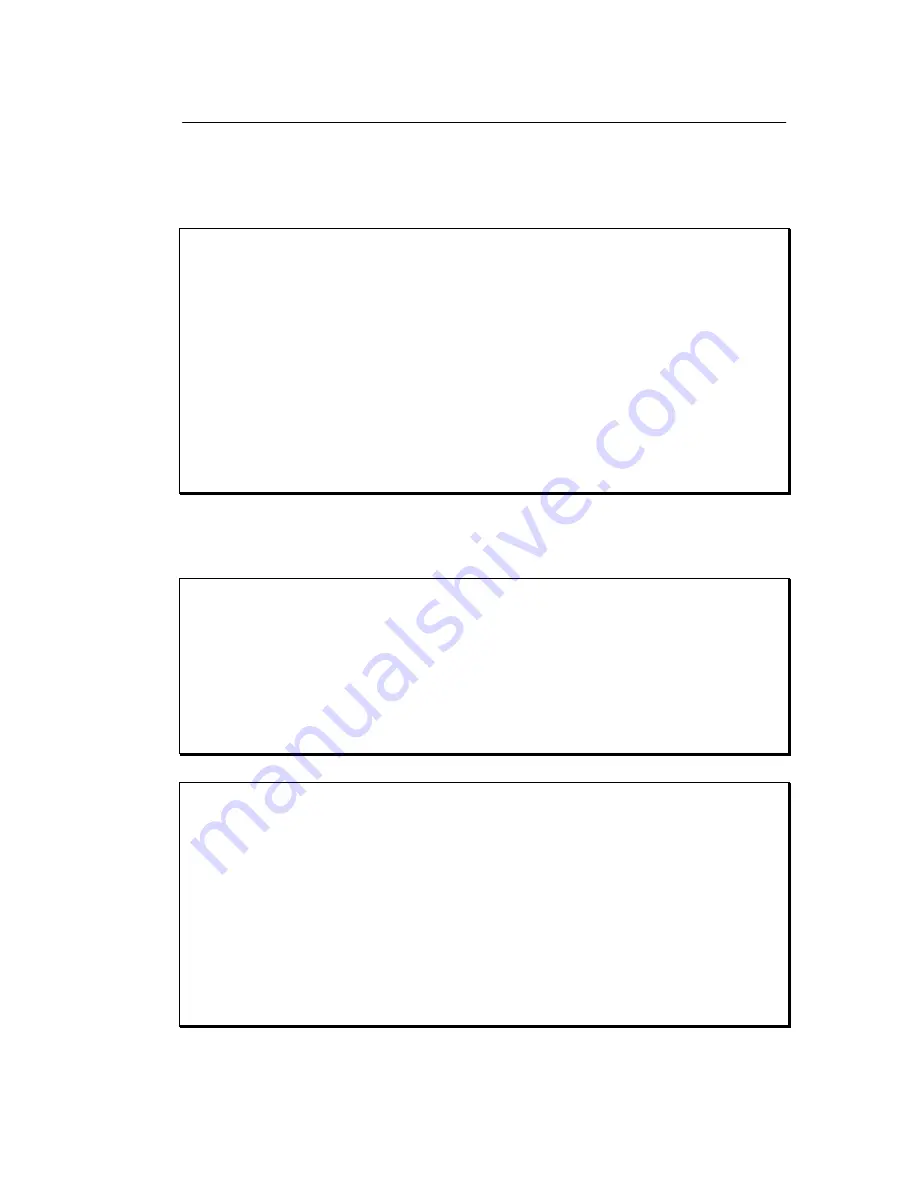
CAN-Engine
Chapter 4: Software
4-15
SOFTWARE INTERFACE
The CAN driver software interface is shown in the header file: \tern\186\include\can.h.
The library file for the CANE implementation is at location: \tern\186\lib\can.lib, and
\tern\186\lib\large\can_l.lib.
CAN messages are defined using this CanMsg structure (similar to SJA1000 hardware representation):
typedef struct _can_msg {
UCHAR8 descriptor[2];
UCHAR8 data[8];
} CanMsg;
The two-byte descriptor field consists of message ID (11 bits), Remote-Transmission-Request/RTR flag (1
bit), and Data Length Code/DLC value (4 bits). These fields can be accessed on a message using these
macros defined in can.h:
SET_CAN_MSG_ID(msg, val)
READ_CAN_MSG_ID(msg)
SET_CAN_MSG_RTR(msg, val)
READ_CAN_MSG_RTR(msg)
SET_CAN_MSG_DLC(msg, val)
READ_CAN_MSG_DLC(msg)
TERN firmware drivers use a ring-buffer to store messages for transmit and receipt. The overall
mechanism is similar to standard serial port implementation (see section 4.1). The best sample
demonstrating these functions is: \tern\186\samples\cane\can_echo.c
int can_set_hw
Arguments: unsigned char board_type
Return values: none
This function configures the CAN port according to the architecture of your board. This function should be
called first, before any other CAN function is accessed. Available
board_type
values are defined in
can.h. If this call is not accurate, the CAN port can not be accessed.
For the CAN-Engine, this call should read:
can_set_hw(BOARD_CANE);
int can_init
Arguments: unsigned char baud, CanMsg* inputBuf, int iSize, CanMsg*
outputBuf, int oSize, unsigned char address, unsigned char mask
Return values: 0 for success, non-zero error code.
This function is used to initialize message buffer support for the CAN port.
Baud
- specifies the baud rate to be used for communication; supported values are defined in can.h. These
include: 1MHz, 500KHz, 250KHz, 125KHz, 100KHz, 50KHz, 20KHz, and 10KHz. Note: at higher baud
rates, termination resistors may be required on your TERN board for clean transmit and receive.
inputBuf, iSize
– these variables represent the ring-buffer allocated for receiving messages.
inputBuf should be a CanMsg array, while iSize indicates the size of the array. TERN drivers will inject
messages into this array on an interrupt-driven basis.