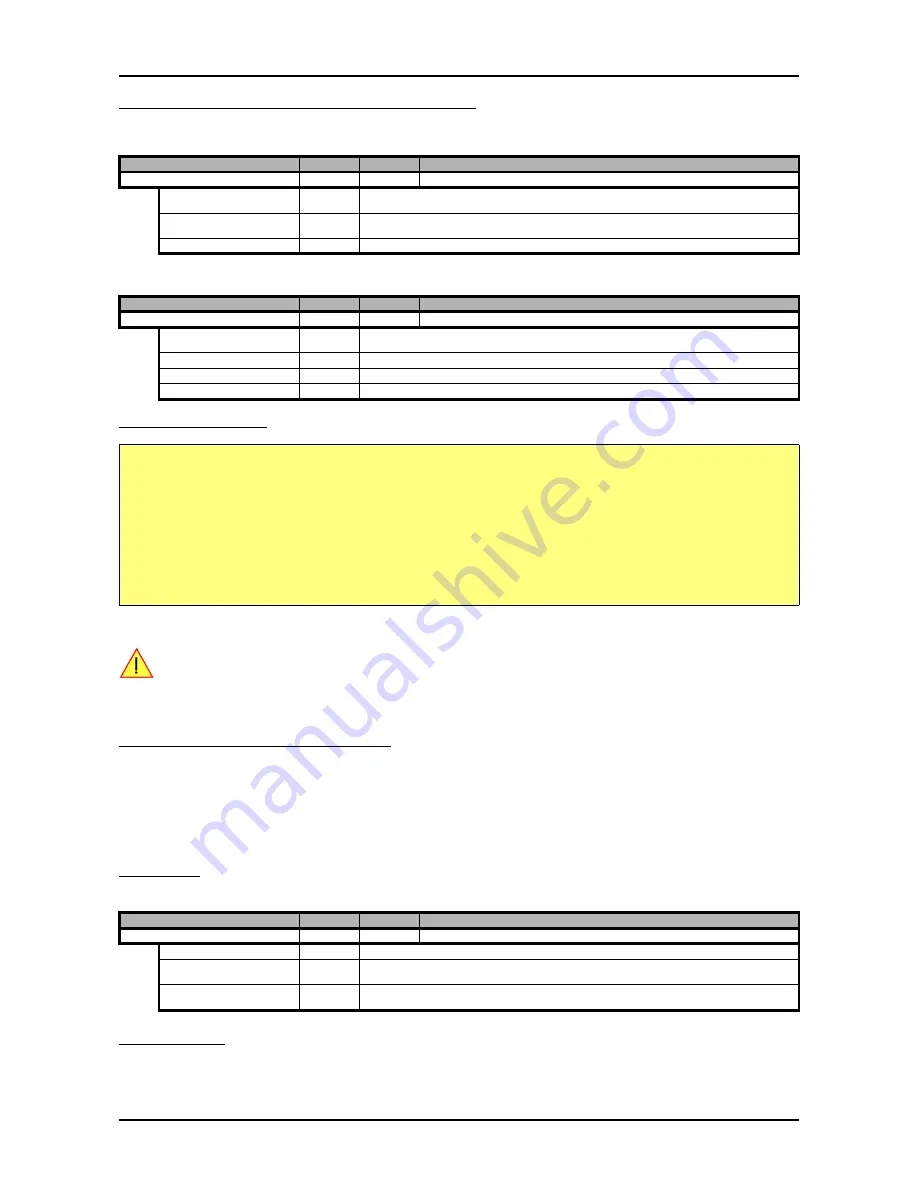
74
M2i.60xx / M2i.60xx-exp Manual
Standard Single Replay modes
Generation modes
Commands and Status information for data transfer buffers.
As explained above the data transfer is performed with the same command and status registers like the card control. It is possible to send
commands for card control and data transfer at the same time as shown in the examples further below.
The data transfer can generate one of the following status information:
Example of data transfer
To keep the example simple it does no error checking. Please be sure to check for errors if using these command in real world programs!
Users should take care to explicitely send the M2CMD_DATA_STOPDMA command prior to invalidating the
buffer, to avoid crashes due to race conditions when using higher-latency data transportation layers, such
as to remote ethernet devices.
Standard Single Replay modes
The standard single modes are the easiest and mostly used modes to generate analog or digital data with a Spectrum arbitrary waveform
generation or digital output card. In standard single replay mode the card is working totally independent from the PC, after the card setup is
done and the data has been transferred into the on-board memory. The advantage of the Spectrum boards is that regardless to the system
usage the card will refresh the outputs with equidistant time intervals.
The data for replay is stored in the on-board memory and is held there for being replayed after the trigger event. This mode allows sample
generation at very high refresh rates without the need to transfer the data from the memory of the host system to the card at high speed.
Card mode
The card mode has to be set to the correct mode SPC_REP_STD_SINGLE.
Memory setup
You have to define, how many samples are to be replayed from the on-board memory and how many times the complete memory should be
replayed after the trigger event.
Register
Value
Direction
Description
SPC_M2CMD
100
write only
Executes a command for the card or data transfer
M2CMD_DATA_STARTDMA
10000h
Starts the DMA transfer for an already defined buffer. In acquisition mode it may be that the card hasn’t received a
trigger yet, in that case the transfer start is delayed until the card receives the trigger event
M2CMD_DATA_WAITDMA
20000h
Waits until the data transfer has ended or until at least the amount of bytes defined by notify size are available. This
wait function also takes the timeout parameter described above into account.
M2CMD_DATA_STOPDMA
40000h
Stops a running DMA transfer. Data is invalid afterwards.
Register
Value
Direction
Description
SPC_M2STATUS
110
read only
Reads out the current status information
M2STAT_DATA_BLOCKREADY
100h
The next data block as defined in the notify size is available. It is at least the amount of data available but it also can
be more data.
M2STAT_DATA_END
200h
The data transfer has completed. This status information will only occur if the notify size is set to zero.
M2STAT_DATA_OVERRUN
400h
The data transfer had on overrun (acquisition) or underrun (replay) while doing FIFO transfer.
M2STAT_DATA_ERROR
800h
An internal error occurred while doing data transfer.
void* pvData = (void*) new int8[1024];
// transfer data from PC memory to card memory
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_PCTOCARD , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// transfer the same data back to PC memory
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_CARDTOPC , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// explicitely stop DMA tranfer prior to invalidating buffer
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STOPDMA);
delete [] (int8*) pvData;
Register
Value
Direction
Description
SPC_CARDMODE
9500
read/write
Defines the used operating mode, a read command will return the currently used mode.
SPC_REP_STD_SINGLE
100h
Data generation from on-board memory replaying the complete programmed memory once.
SPC_REP_STD_CONTINUOUS
4000h
Data generation from on-board memory replaying the complete memory either endless until stopped by user or a pro-
grammed number of loops.
SPC_REP_STD_SINGLERESTART
8000h
Data generation from on-board memory replaying the complete programmed memory on every detected trigger
event. The number of replays can be programmed by loops.