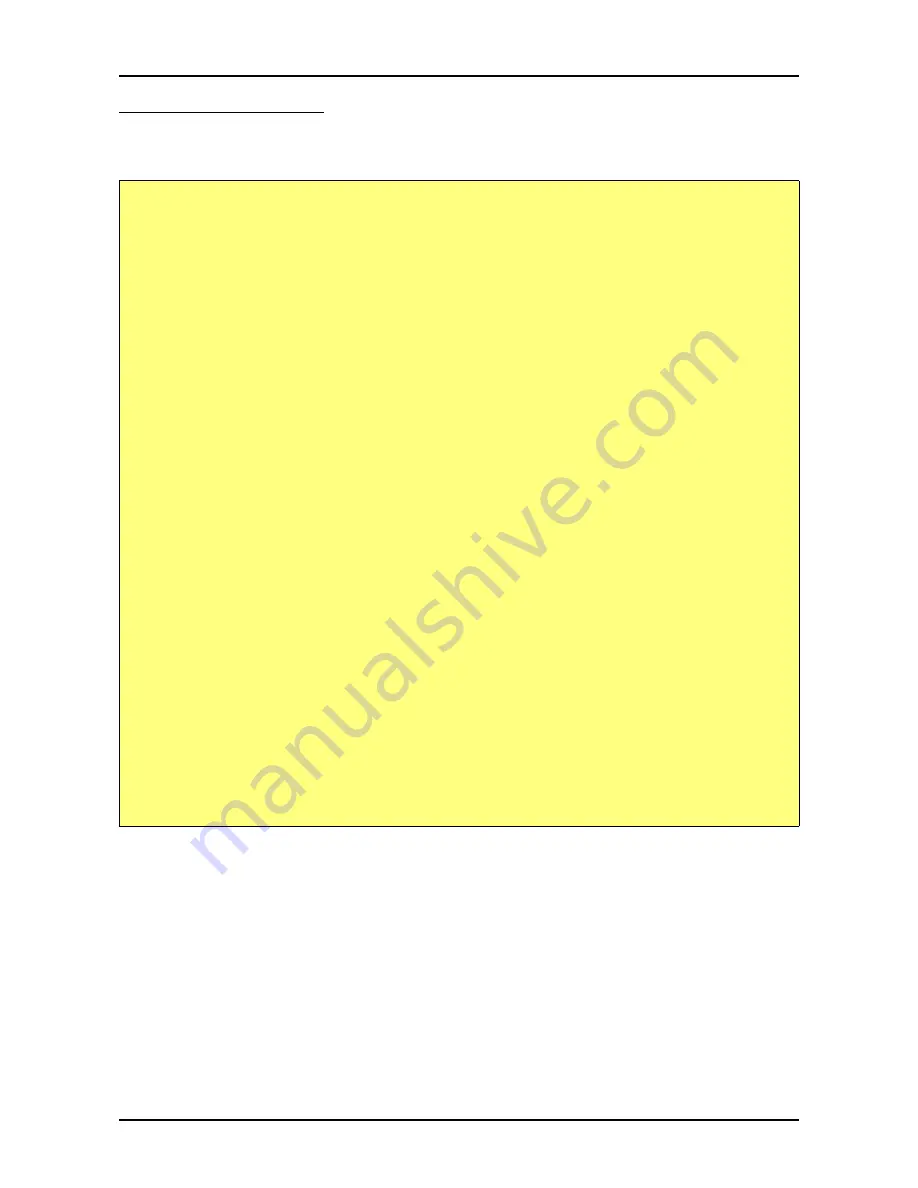
112
M2i.60xx / M2i.60xx-exp Manual
Programming example
Sequence Replay Mode
Programming example
The following example shows a very simple sequence as an example. Only two segments are used, the first is replayed 10 times and then
unconditionally left and replay switches over to the second segment. This segment is repeated until a trigger event is detected by the card.
After the trigger has been detected the sequence starts over again... until the card is stopped.
// Setup of channel enable, output conditioning as well as trigger setup not shown for simplicity
#define MAX_SEGMENTS 2 // only 2 segments used here for simplicity
int32 lBytesPerSample;
// Read out used bytes per sample
spcm_dwGetParam_i32 (hDrv, SPC_MIINST_BYTESPERSAMPLE, &lBytesPerSample);
// Setting up the card mode
spcm_dwSetParam_i32 (hDrv, SPC_CARDMODE, SPC_REP_STD_SEQUENCE); // enable sequence mode
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_MAXSEGMENTS, 2); // Divide on-board mem in two parts
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_STARTSTEP, 0); // Step#0 is the first step after card start
// Setting up the data memory and transfer data
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_WRITESEGMENT, 0); // set current configuration switch to segment 0
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_SEGMENTSIZE, 1024); // define size of current segment 0
// it is assumed, that the Buffer memory has been allocated and is already filled with valid data
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_PCTOCARD, 0, pData, 0, 1024 * lBytesPerSample);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// Setting up the data memory and transfer data
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_WRITESEGMENT, 1); // set current configuration switch to segment 1
spcm_dwSetParam_i32 (hDrv, SPC_SEQMODE_SEGMENTSIZE, 512); // define size of current segment 1
// it is assumed, that the Buffer memory has been allocated and is already filled with valid data
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_PCTOCARD, 0, pData, 0, 512 * lBytesPerSample);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// Setting up the sequence memory (Only two steps used here as an example)
lStep = 0; // current step is Step#0
llSegment = 0; // associated with memory
llLoop = 10; // Pattern will be repeated 10 times
llNext = 1; // Next step is Step#1
llCondition = SPCSEQ_ENDLOOPALWAYS; // Unconditionally leave current step
// combine all the parameters to one int64 bit value
llValue = (llCondition << 32) | (llLoop << 32) | (llNext << 16) | (llSegment);
spcm_dwSetParam_i64 (hDrv, SPC_SEQMODE_ST lStep, llValue);
lStep = 1; // current step is Step#1
llSegment = 1; // associated with memory segment 1
llLoop = 1; // Pattern will be repeated once before condition is checked
llNext = 0; // Next step is Step#0
llCondition = SPCSEQ_ENDLOOPNTRIG; // Repeat current step until a trigger has occurred
llValue = (llCondition << 32) | (llLoop << 32) | (llNext << 16) | (llSegment);
spcm_dwSetParam_i64 (hDrv, SPC_SEQMODE_ST lStep, llValue);
// Start the card
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_CARD_START | M2CMD_CARD_ENABLETRIGGER);
// ... wait here or do something else ...
// Stop the card
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_CARD_STOP);