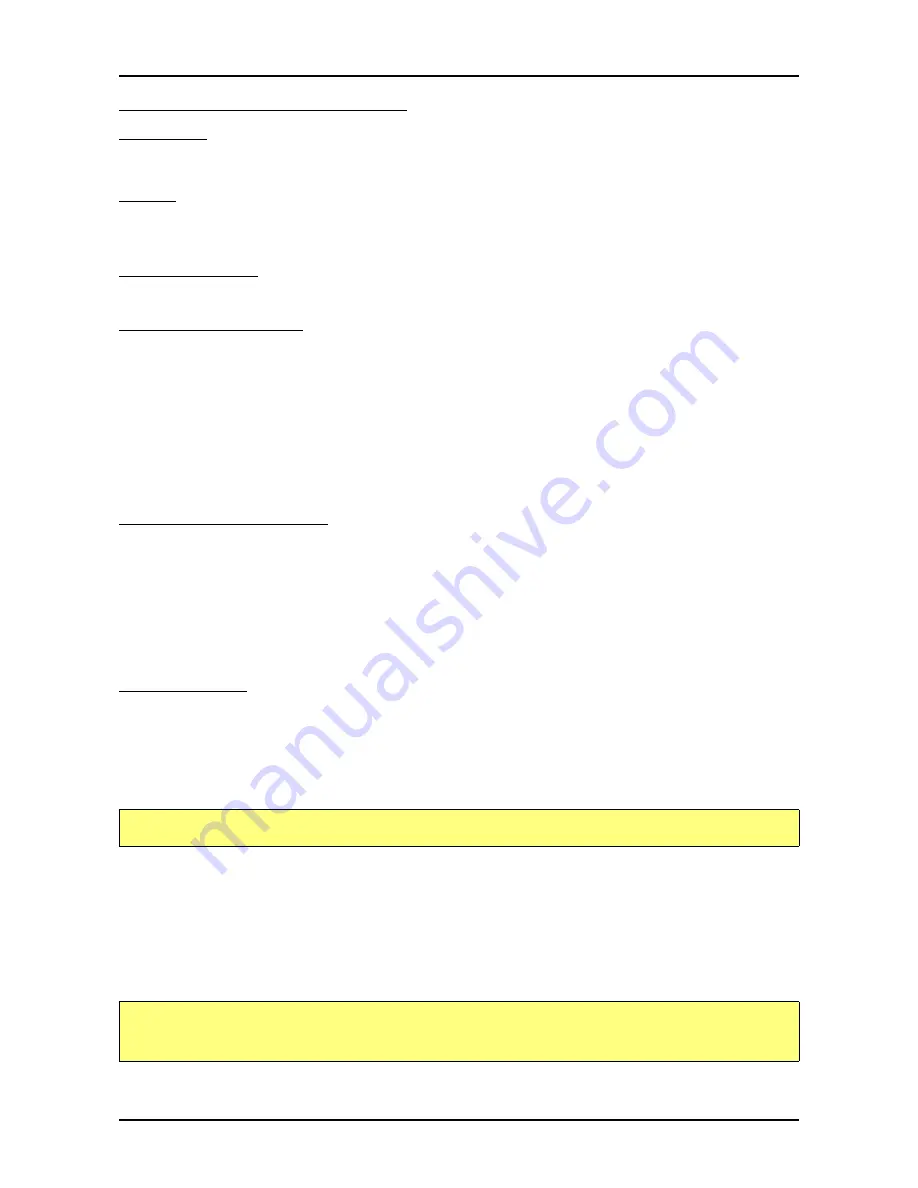
Software
Driver functions
(c) Spectrum GmbH
45
National Instruments LabWindows/CVI
Include Drivers
To use the Spectrum driver under LabWindows/CVI it is necessary to first load the functions from the driver dll. Please use the library file
spcm_win32_cvi.lib to access the driver functions.
Examples
Examples for LabWindows/CVI can be found on CD in the directory /examples/cvi. Please mix these examples with the standard C/C++
examples to have access to all functions and modes of the cards.
Driver functions
The driver contains seven main functions to access the hardware.
Own types used by our drivers
To simplify the use of the header files and our examples with different platforms and compilers and to avoid any implicit type conversions we
decided to use our own type declarations. This allows us to use platform independent and universal examples and driver interfaces. If you
do not stick to these declarations please be sure to use the same data type width. However it is strongly recommended that you use our defined
type declarations to avoid any hard to find errors in your programs. If you’re using the driver in an environment that is not natively supported
by our examples and drivers please be sure to use a type declaration that represents a similar data width
Notation of variables and functions
In our header files and examples we use a common and reliable form of notation for variables and functions. Each name also contains the
type as a prefix. This notation form makes it easy to see implicit type conversions and minimizes programming errors that results from using
incorrect types. Feel free to use this notation form for your programs also-
Function spcm_hOpen
This function initializes and opens an installed card supporting the new SpcM driver interface. At the time of printing this manual this are all
cards of the M2i/M3i cards. The function returns a handle that has to used for driver access. If the card can’t be found or the loading of the
driver generated an error the function returns a NULL. When calling this function all card specific installation parameters are read out from
the hardware and stored within the driver. It is only possible to open one device by one software as concurrent hardware access may be
very critical to system stability. As a result when trying to open the same device twice an error will raise and the function returns NULL.
Function spcm_hOpen (char* szDeviceName):
Under Linux the device name in the function call need to be a valid device name. Please change the string according to the location of the
device if you don’t use the standard linux device names. The driver is installed as default under /dev/spcm0, /dev/spcm1 and so on. The
kernel driver numbers the devices starting with 0.
Under windows the only part of the device name that is used is the tailing number. The rest of the device name is ignored. Therefore to keep
the examples simple we use the Linux notation in all our examples. The tailing number gives the index of the device to open. The Windows
kernel driver numbers all devices that it finds on boot time starting with 0.
Example for local installed cards
Declaration
Type
Declaration
Type
int8
8 bit signed integer (range from -128 to +127)
uint8
8 bit unsigned integer (range from 0 to 255)
int16
16 bit signed integer (range from -32768 to 32767)
uint16
16 bit unsigned integer (range from 0 to 65535)
int32
32 bit signed integer (range from -2147483648 to 2147483647)
uint32
32 bit unsigned integer (range from 0 to 4294967295)
int64
64 bit signed integer (full range)
uint64
64 bit unsigned integer (full range)
drv_handle
handle to driver, implementation depends on operating system platform
Declaration
Notation
Declaration
Notation
int8
byName (byte)
uint8
cName (character)
int16
nName
uint16
wName (word)
int32
lName (long)
uint32
dwName (double word)
int64
llName (long long)
uint64
qwName (quad word)
int32*
plName (pointer to long)
char
szName (string with zero termination)
drv_handle _stdcall spcm_hOpen ( // tries to open the device and returns handle or error code
char* szDeviceName); // name of the device to be opened
drv_handle hDrv; // returns the handle to the opended driver or NULL in case of error
hDrv = spcm_hOpen ("/dev/spcm0"); // string to the driver to open
if (!hDrv)
printf (“open of driver failed\n”);