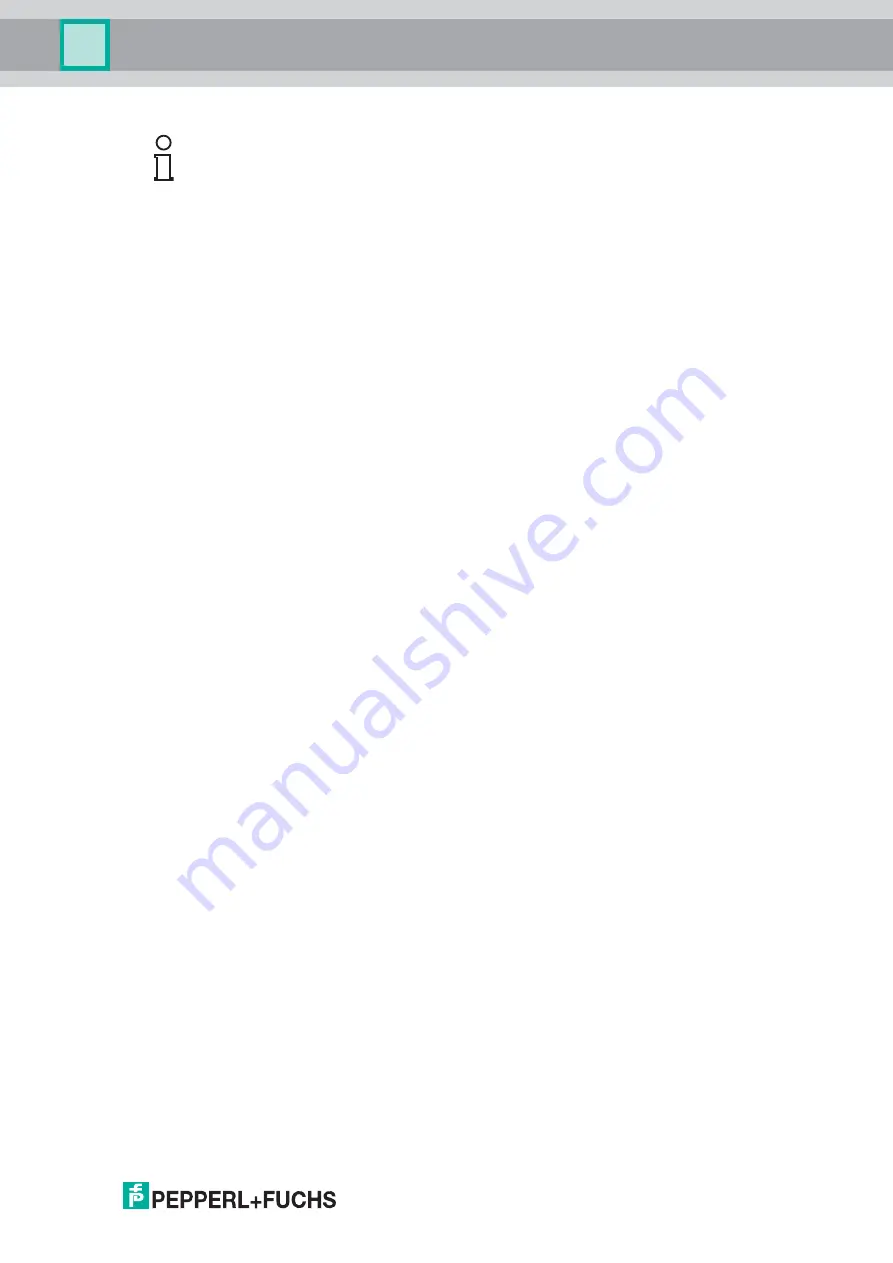
Communicating with the OIT System
2
019-
03
57
General
The library is used to support the creation of a graphic user interface for sensors that use the
VSX protocol. The library connects to the sensor and handles the communication in
accordance with the protocol. The user has functions for setting parameters on the sensor,
retrieving parameter values from the sensor, and saving and loading whole parameter sets
both locally and on the sensor. The user can also receive sensor images.
The library is implemented in C# and requires .NET 2.0 or higher as a minimum.
Make sure that the libraries supplied are in the project's execution folder.
Creating an Object
Create an object to access the library functions.
PFVsxFactoryVCCustom _vsxFactory =new PFVsxFactoryVCCustom();
Retrieving Parameter Data
Received parameter data is stored as a list in the sensor. Individual items of parameter data
from this list can be retrieved using the following function:
string GetSpecificSingleParameter(string version, string configId,
string parameterId)
Displaying Modified Data
If data is being received by the sensor, this is indicated by the event:
event ParameterDataReceived(DataModifier modifier)
Example!
Below is a sample program for integrating and triggering the sensor:
class Program
{
static void Main(string[] args)
{
PF.Foundation.VsxFactory.PFVsxFactoryVCCustom sensor;
sensor = new PF.Foundation.VsxFactory.PFVsxFactoryVCCustom();
sensor.Connect("192.168.2.3", 50005);
sensor.SetSpecificSingleParameter("Command", "TriggerStart",
"1");
System.Threading.Thread.Sleep(1000);
sensor.Disconnect();
}
}