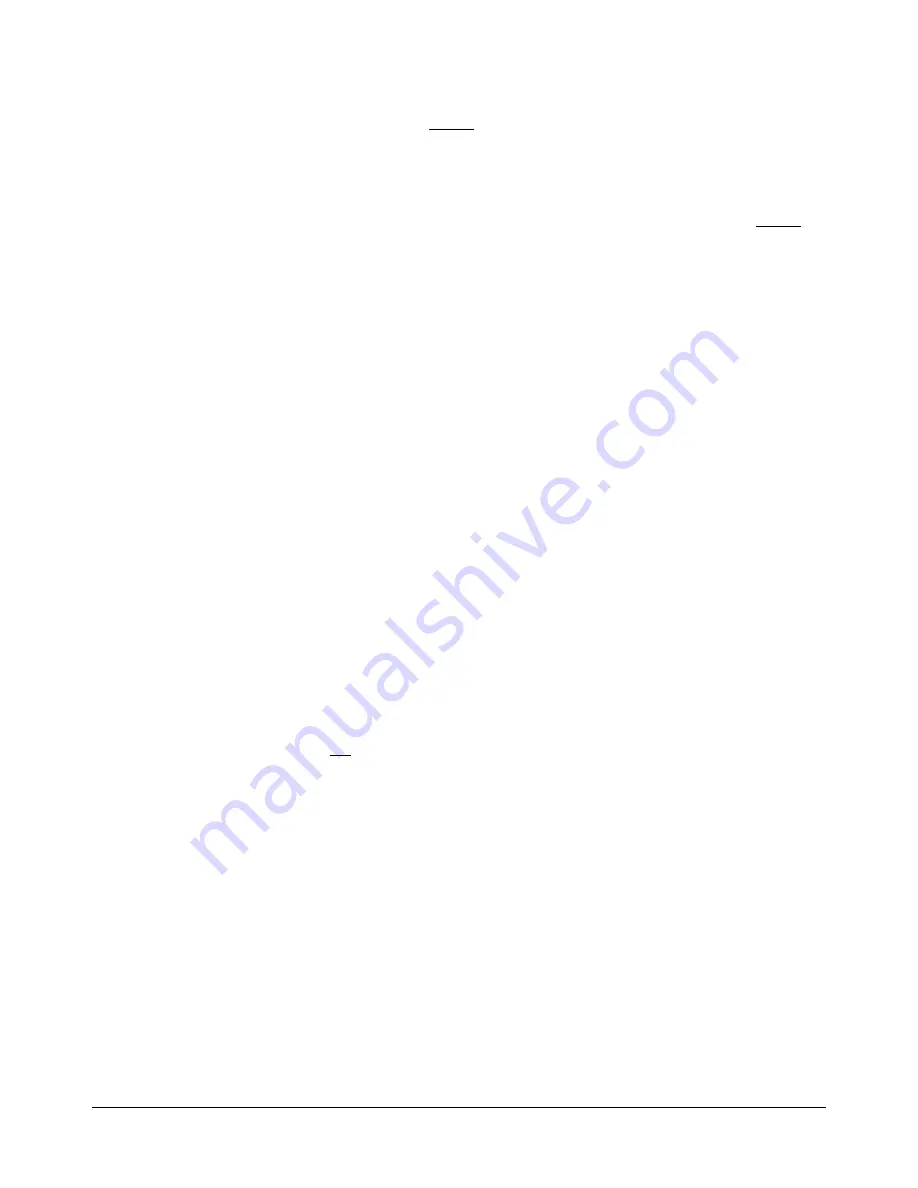
Algorithm Language Reference
141
When the HP E1415’s translator receives this statement, it simplifies it by
adding the two integer constants (5 and 8) and storing the sum of these as the
float constant 13. At algorithm run-time, the float constant 13 is assigned to
the variable "a". No surprises so far. Now analyze this statement;
a = ( 3 / 4 ) * 12;
Again the translator simplifies the expression by performing the integer
divide for 3 / 4. This results in the integer value 0 being multiplied by 12
which results in the float constant 0.0 being assigned to the variable "a" at
run-time. This is obviously not what you wanted but is exactly what your
algorithm instructed.
You can avoid these subtle problems by specifically including a decimal
point in decimal constants where an integer operation is not what you want.
For example, if you had made either of the constants in the division above a
float constant by including a decimal point, the translator would have
promoted the other constant to a float value and performed a float divide
operation resulting in the expected 0.75 * 12, or the value 8.0 So the
statement;
a = ( 3. / 4 ) * 12;
will result in the value float 8.0 being assigned to the variable "a".
The Static Modifier
All HP E1415 variables, local or global, must be declared as static. An
example:
static float gain_var, integer_var, deriv_var;
/* three vars declared */
In ’C’, local variables that are not declared as static lose their values once the
function completes. The value of a local static variable remains unchanged
between calls to your algorithm. Treating all variables this way allows your
algorithm to "remember" its previous state. The static variable is local in
scope, but otherwise behaves as a global variable. Also note that you may
not declare variables within a compound statement.
Data Structures
The HP E1415 Algorithm Language allows the following data structures:
•
Simple variables of type float:
Declaration
static float simp_var, any_var;
Use
simp_var = 123.456;
any_var = -23.45;
Another_var = 1.23e-6;
Storage
Each simple variable requires four 16-bit words of memory.
•
Single-dimensioned arrays of type float with a maximum of 1024
Summary of Contents for VXI 75000 C Series
Page 2: ......
Page 16: ...16 ...
Page 18: ......
Page 30: ...30 Getting Started Chapter 1 Notes ...
Page 32: ...32 Field Wiring Chapter 2 Figure 2 1 Channel Numbers at SCP Positions ...
Page 44: ...44 Field Wiring Chapter 2 Figure 2 11 HP E1415 Terminal Module ...
Page 54: ...54 Field Wiring Chapter 2 Notes ...
Page 61: ...Programming the HP E1415 for PID Control 61 Chapter 3 Programming Overview Diagram ...
Page 136: ...136 Creating and Running Custom Algorithms Chapter 4 Notes ...
Page 152: ...152 Algorithm Language Reference Chapter 5 Notes ...
Page 304: ...304 HP E1415 Command Reference Chapter 6 Command Quick Reference Notes ...
Page 308: ...308 Specifications Appendix A Thermocouple Type E 200 800C SCPs HP E1501 02 03 ...
Page 309: ...Specifications 309 Appendix A Thermocouple Type E 200 800C SCPs HP E1508 09 ...
Page 310: ...310 Specifications Appendix A Thermocouple Type E 0 800C SCPs HP E1501 02 03 ...
Page 311: ...Specifications 311 Appendix A Thermocouple Type E 0 800C SCPs HP E1509 09 ...
Page 312: ...312 Specifications Appendix A Thermocouple Type E Extended SCPs HP E1501 02 03 ...
Page 313: ...Specifications 313 Appendix A Thermocouple Type E Extended SCPs HP E1508 09 ...
Page 314: ...314 Specifications Appendix A Thermocouple Type J SCPs HP E1501 02 03 ...
Page 315: ...Specifications 315 Appendix A Thermocouple Type J SCPs HP E1508 09 ...
Page 316: ...316 Specifications Appendix A Thermocouple Type K SCPs HP E1501 02 03 ...
Page 317: ...Specifications 317 Appendix A Thermocouple Type R SCPs HP E1501 02 03 ...
Page 318: ...318 Specifications Appendix A Thermocouple Type R SCPs HP E1508 09 ...
Page 319: ...Specifications 319 Appendix A Thermocouple Type S SCPs HP E1501 02 03 ...
Page 320: ...320 Specifications Appendix A Thermocouple Type S SCPs HP E1508 09 ...
Page 321: ...Specifications 321 Appendix A Thermocouple Type T SCPs HP E1501 02 03 ...
Page 322: ...322 Specifications Appendix A Thermocouple Type T SCPs HP E1508 09 ...
Page 323: ...Specifications 323 Appendix A 5K Thermistor Reference SCPs HP E1501 02 03 ...
Page 324: ...324 Specifications Appendix A 5K Thermistor Reference SCPs HP E1508 09 ...
Page 325: ...Specifications 325 Appendix A RTD Reference SCPs HP E1501 02 03 ...
Page 326: ...326 Specifications Appendix A RTD SCPs HP E1501 02 03 ...
Page 327: ...Specifications 327 Appendix A RTD SCPs HP E1508 09 ...
Page 328: ...328 Specifications Appendix A 2250 Thermistor SCPs HP E1501 02 03 ...
Page 329: ...Specifications 329 Appendix A 2250 Thermistor SCPs HP E1508 09 ...
Page 330: ...330 Specifications Appendix A 5K Thermistor SCPs HP E1501 02 03 ...
Page 331: ...Specifications 331 Appendix A 5K Thermistor SCPs HP E1508 09 ...
Page 332: ...332 Specifications Appendix A 10K Thermistor SCPs HP E1501 02 03 ...
Page 333: ...Specifications 333 Appendix A 10K Thermistor SCPs HP E1508 09 ...
Page 334: ...334 Specifications Appendix A Notes ...
Page 346: ...346 Glossary Appendix C Notes ...
Page 388: ...388 Generating User Defined Functions Appendix F Notes ...