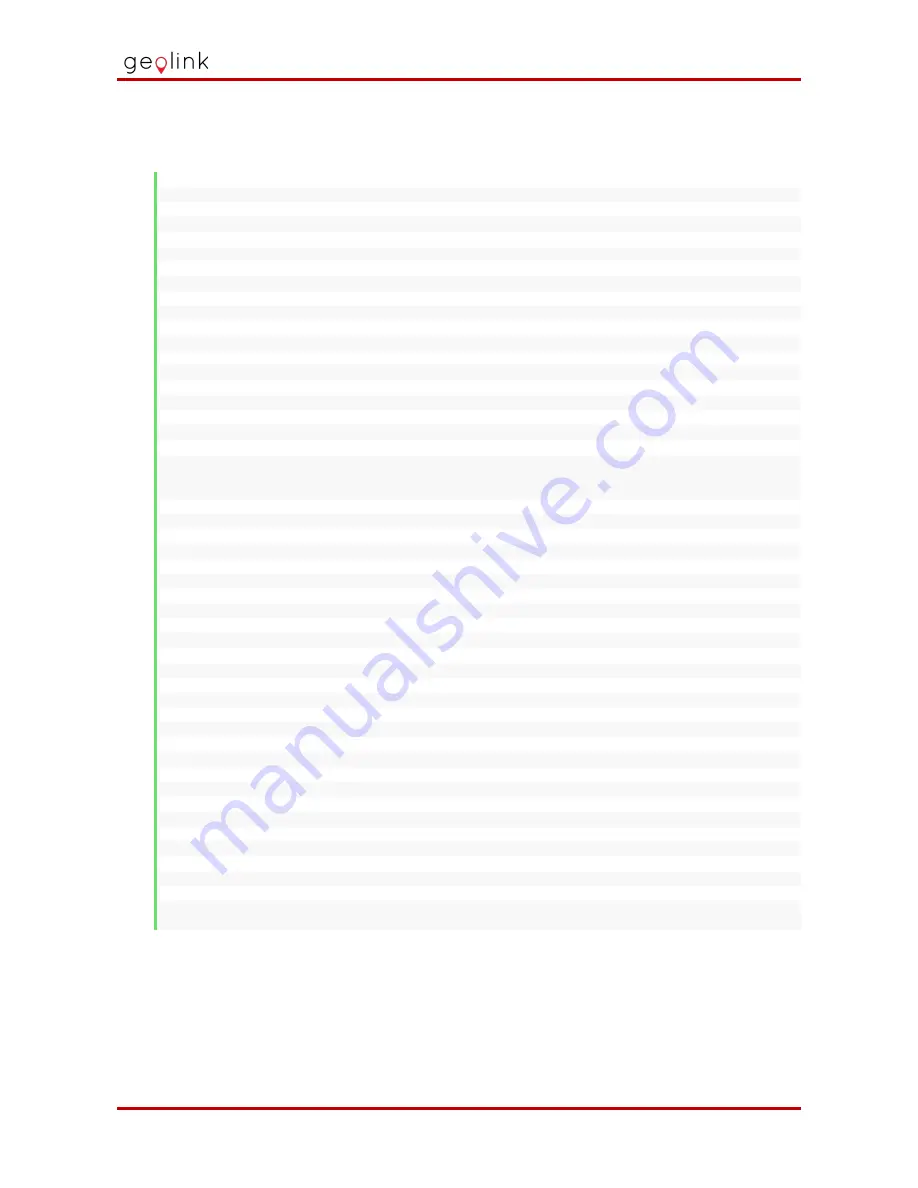
www.geolink.io
User Manual (1.4.0)
OpenTracker 2.4
15
Using the Inputs (IN1 / IN2)
This example shows how to read the analog inputs on the Main Connector (External IO) and print the output
to the debug port.
1.
#define DEBUG 1 //enable debug msg, sent to serial port
2.
#define debug_port SerialUSB
3.
4.
#ifdef DEBUG
5.
#define debug_print(x) debug_port.print(x)
6.
#else
7.
#define debug_print(x)
8.
#endif
9.
10.
// Variables will change:
11.
int
outputValue;
12.
int
sensorValue;
13.
14.
15.
void
setup() {
16.
// put your setup code here, to run once:
17.
18.
}
19.
20.
void
loop() {
21.
22.
// Read IN1 Value
23.
// read the analog in value:
24.
sensorValue = analogRead(AIN_EXT_IN1);
25.
// map it to the range of the analog out:
26.
outputValue = sensorValue * (242.0f / 22.0f * ANALOG_VREF / 1024.0f);
27.
28.
// print the results to the serial monitor:
29.
debug_print(F(
"IN1 = "
));
30.
debug_print(outputValue);
31.
debug_print(F(
"V ("
));
32.
debug_print(sensorValue);
33.
debug_print(F(
")"
));
34.
debug_port.println(
" "
);
35.
36.
// Read IN2 Value
37.
// read the analog in value:
38.
sensorValue = analogRead(AIN_EXT_IN2);
39.
// map it to the range of the analog out:
40.
outputValue = sensorValue * (242.0f / 22.0f * ANALOG_VREF / 1024.0f);
41.
42.
// print the results to the serial monitor:
43.
debug_print(F(
"IN2 = "
));
44.
debug_print(outputValue);
45.
debug_print(F(
"V ("
));
46.
debug_print(sensorValue);
47.
debug_print(F(
")"
));
48.
debug_port.println(
" "
);
49.
50.
delay(1000);
51.
}