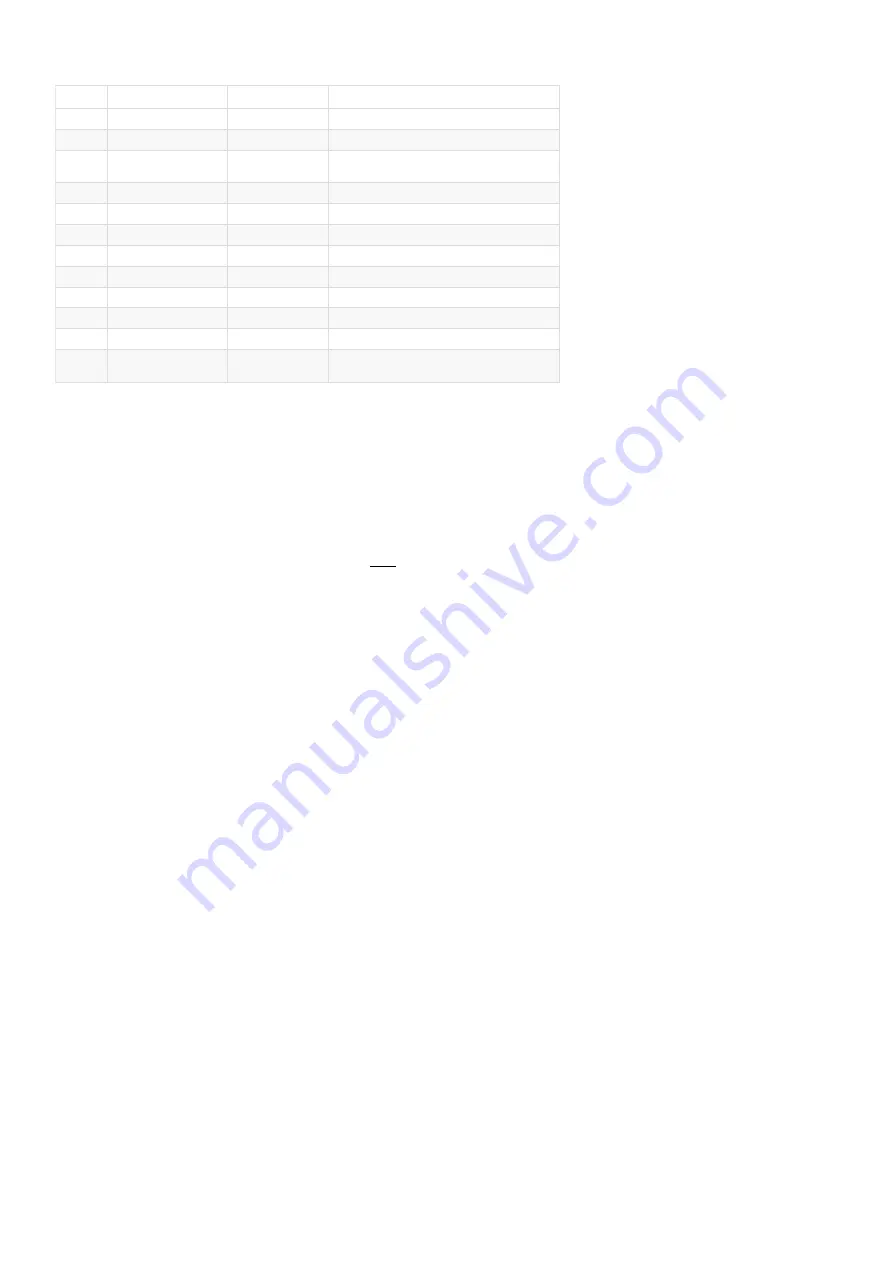
The ESP8266 has one SPI connection available to the user, referred to as HSPI. It uses GPIO14 as CLK, 12 as MISO, 13 as MOSI and 15
as Slave Select (SS). It can be used in both Slave and Master mode (in software).
GPIO overview
GPIO
Function
State
Restrictions
0
Boot mode select
3.3V
No Hi-Z
1
TX0
-
Not usable during Serial transmission
2
Boot mode select
TX1
3.3V (boot only)
Don’t connect to ground at boot time
Sends debug data at boot time
3
RX0
-
Not usable during Serial transmission
4
SDA (I²C)
-
-
5
SCL (I²C)
-
-
6 - 11
Flash connection
x
Not usable, and not broken out
12
MISO (SPI)
-
-
13
MOSI (SPI)
-
-
14
SCK (SPI)
-
-
15
SS (SPI)
0V
Pull-up resistor not usable
16
Wake up from sleep
-
No pull-up resistor, but pull-down instead
Should be connected to RST to wake up
The ESP8266 as a microcontroller - Software
Most of the microcontroller functionality of the ESP uses exactly the same syntax as a normal Arduino, making it really easy to get
started.
Digital I/O
Just like with a regular Arduino, you can set the function of a pin using
pinMode(pin, mode);
where
pin
is the GPIO number*, and
mode
can
be either
INPUT
, which is the default,
OUTPUT
, or
INPUT_PULLUP
to enable the built-in pull-up resistors for GPIO 0-15. To enable the pull-
down resistor for GPIO16, you have to use
INPUT_PULLDOWN_16
.
(*) NodeMCU uses a different pin mapping, read more here. To address a NodeMCU pin, e.g. pin 5, use D5: for instance:
pinMode(D5,
OUTPUT);
To set an output pin high (3.3V) or low (0V), use
digitalWrite(pin, value);
where
pin
is the digital pin, and
value
either 1 or 0 (or
HIGH
and
LOW
).
To read an input, use
digitalRead(pin);
To enable PWM on a certain pin, use
analogWrite(pin, value);
where
pin
is the digital pin, and
value
a number between 0 and 1023.
You can change the range (bit depth) of the PWM output by using
analogWriteRange(new_range);
The frequency can be changed by using
analogWriteFreq(new_frequency);
.
new_frequency
should be between 100 and 1000Hz.
Analog input
Just like on an Arduino, you can use
analogRead(A0)
to get the analog voltage on the analog input. (0 = 0V, 1023 = 1.0V).
The ESP can also use the ADC to measure the supply voltage (V
CC
). To do this, include
ADC_MODE(ADC_VCC);
at the top of your sketch, and
use
ESP.getVcc();
to actually get the voltage.
If you use it to read the supply voltage, you can’t connect anything else to the analog pin.
Communication
Serial communication
To use UART0 (TX = GPIO1, RX = GPIO3), you can use the
Serial
object, just like on an Arduino:
Serial.begin(baud)
.
To use the alternative pins (TX = GPIO15, RX = GPIO13), use
Serial.swap()
after
Serial.begin
.
To use UART1 (TX = GPIO2), use the
Serial1
object.
All Arduino Stream functions, like
read, write, print, println, ...
are supported as well.
I²C and SPI
You can just use the default Arduino library syntax, like you normally would.
Sharing CPU time with the RF part
One thing to keep in mind while writing programs for the ESP8266 is that your sketch has to share resources (CPU time and memory)
with the Wi-Fi- and TCP-stacks (the software that runs in the background and handles all Wi-Fi and IP connections).
If your code takes too long to execute, and don’t let the TCP stacks do their thing, it might crash, or you could lose data. It’s best to
keep the execution time of you loop under a couple of hundreds of milliseconds.
Every time the main loop is repeated, your sketch yields to the Wi-Fi and TCP to handle all Wi-Fi and TCP requests.
If your loop takes longer than this, you will have to explicitly give CPU time to the Wi-Fi/TCP stacks, by using including
delay(0);
or
yield();
. If you don’t, network communication won’t work as expected, and if it’s longer than 3 seconds, the soft WDT (Watch Dog
Timer) will reset the ESP. If the soft WDT is disabled, after a little over 8 seconds, the hardware WDT will reset the chip.