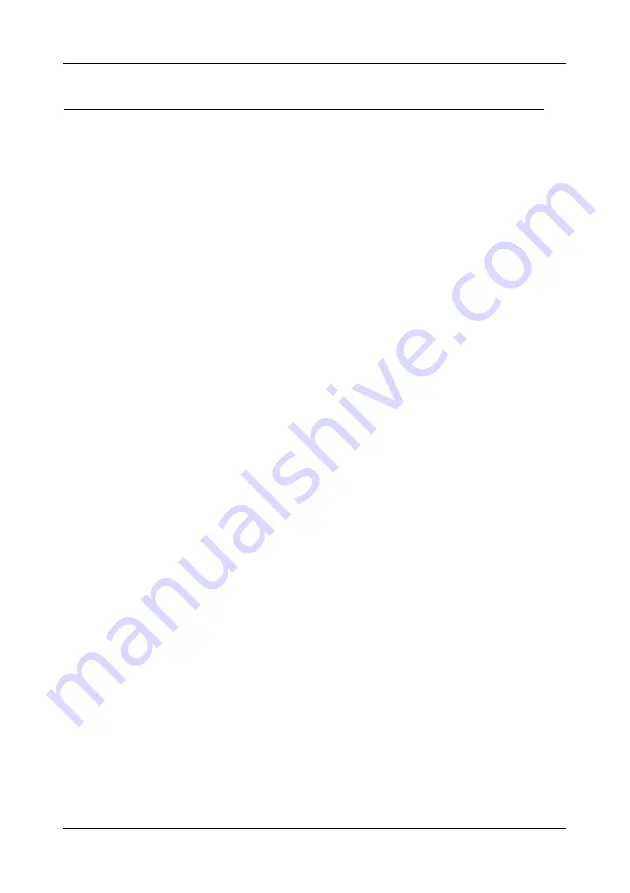
IIC-Bus
The port pins PJ6 and PJ7 grant access to the Inter-IC-Bus module
(IIC/I2C/I
2
C) of the MC9S12DP512. Since the IIC-Bus is implemented
as a hardware module, an IIC software emulation is obsolete.
For the two IIC-Bus signals (SDA, SCL), pull-up resistors are
required. They can be soldered in directly on the S12compact PCB
(R10, R11) or they can be added externally.
The following listing shows a simplified Master Mode implementa-
tion without using interrupts:
//=============================================================================
// File: S12_IIC.C - V1.00
// Func: Simplified I2C (Inter-IC Bus) Master Mode implementation
// using the IIC hardware module of the HCS12
// Rem.: For a real-world implementation, an interrupt-driven scheme should
// be preferred. See AppNote AN2318 and accompanying software!
// Hard: External pull-ups on SDA and SCL required!
// Value should be 1k..5k depending on cap. bus load
// Note: Adjust IBFD value if ECLK is not 8MHz!
//=============================================================================
//-- Includes -----------------------------------------------------------------
#include "datatypes.h"
#include <mc9s12dp512.h>
#include "s12_iic.h"
//-- Code ---------------------------------------------------------------------
// Func: Initialize IIC module
// Args: -
// Retn: -
//
void initIIC(void) {
IBFD = 0x18; // 100kHz IIC clock at 8MHz ECLK
// IBFD = 0x1f; // 100kHz IIC clock at 24MHz ECLK
IBCR = BM_IBEN; // enable IIC module, still slave
IBSR = BM_IBIF | BM_IBAL; // clear pending flags (just in case...)
}
//-----------------------------------------------------------------------------
// Func: Issue IIC Start Condition
// Args: -
// Retn: -
//
void startIIC(void) {
while((IBSR & BM_IBB) != 0) // wait if bus busy
; // CAUTION! no loop time limit implemented
IBCR = BM_IBEN | BM_MSSL | BM_TXRX; // transmit mode, master (issue START cond.)
while((IBSR & BM_IBB) == 0) // wait for busy state
; // CAUTION! no loop time limit implemented
}
//-----------------------------------------------------------------------------
S12compact
32