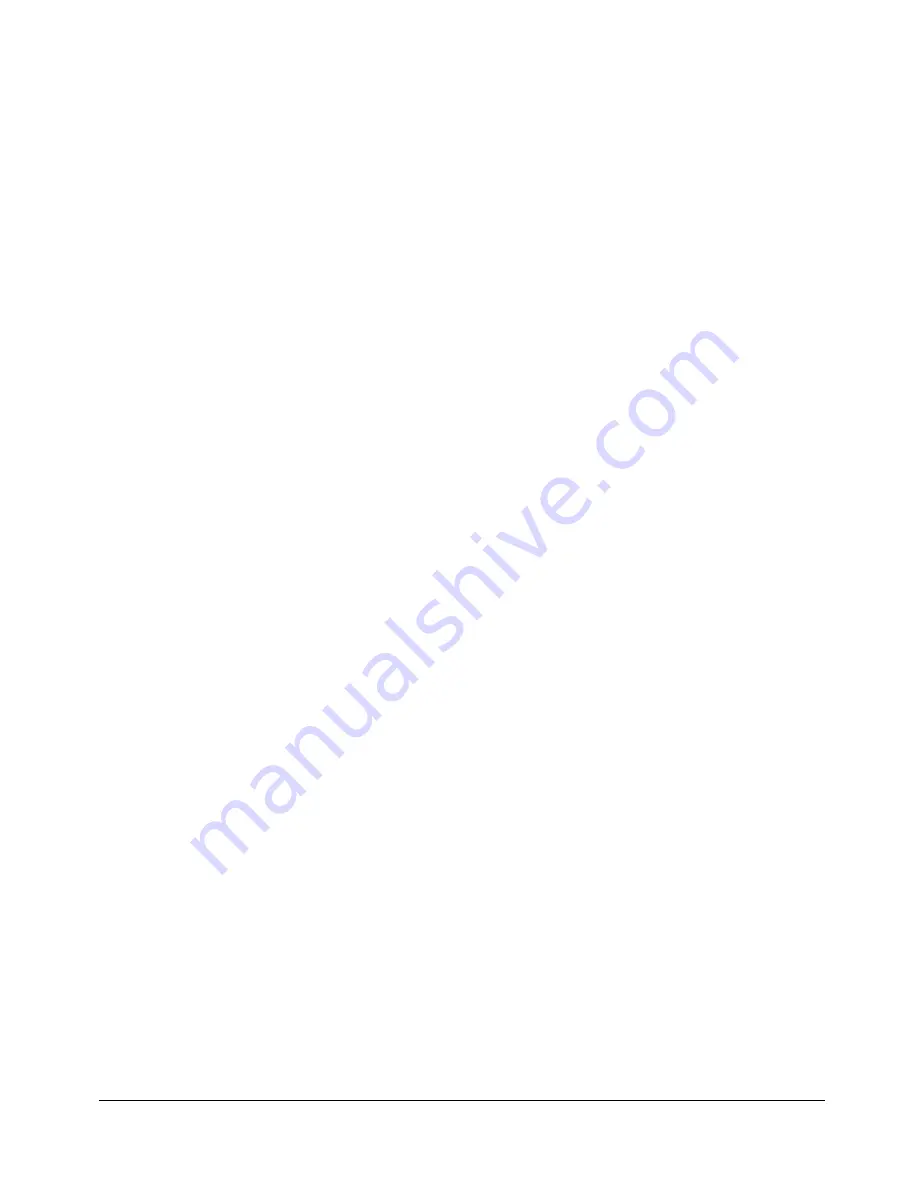
Copyright © 2014, Dr Robot Inc. All Rights Reserved. www.DrRobot.com V.04.11.14
- 23 -
Motion Control/Sensing System
Jaguar comes with a special version of PMS5005 as its motion control and sensing board. It follows the Dr Robot
WiRobotSDK protocol. User could control and access Jaguar by Dr Robot ActiveX control
(DrRobotSentinelActivexControl.ocx) and WiRobot gateway program.
Based on the protocol, you could develop your own program for any operation system. You could request
protocol sample code from Dr Robot using C++/Java. You should also contact Dr Robot with any questions
regarding SDK API and protocol.
The communication port is connected at Ethernet module-I port 1.
Gateway program will connect to this board at 192.168.0.60, port 10001.
Here is C# sample code to control Jaguar System with ActiveX control,
myJaguar
is DrRobotSentinelActiveXControl.
Arm and drive motor control:
private
void
myJaguar_MotorSensorEvent(
object
sender,
EventArgs
e)
{
//here is front arm ouput pwm value
armMotor[0].pwmOutput = myJaguar.GetMotorPWMValue1();
//here is front left arm encoder reading
armMotor[0].encoderDir = myJaguar.GetEncoderDir1();
armMotor[0].encoderPos = myJaguar.GetEncoderPulse1();
armMotor[0].encodeSpeed = myJaguar.GetEncoderSpeed1();
//here is front right arm encoder reading
armMotor[1].encoderDir = myJaguar.GetEncoderDir2();
armMotor[1].encoderPos = myJaguar.GetEncoderPulse2();
armMotor[1].encodeSpeed = myJaguar.GetEncoderSpeed2();
forwardPower = myJaguar.GetMotorPWMValue4();
turnPower = myJaguar.GetMotorPWMValue5();
}
You could read board voltage(5V) and battery voltage in standard sensor Event.
private
void
myJaguar_StandardSensorEvent(
object
sender,
EventArgs
e)
{
boardVol = ((
double
) myJaguar.GetSensorBatteryAD1() / 4095 * 9);
motVol = ((
double
) myJaguar.GetSensorBatteryAD2() / 4095 *
34.498);
}
You could read motor temperature in custom sensor event, function Trans2Temperature() is based on the sensor
specification to translate AD value to temperature (in celcius degree).
Also you could read left and right track/wheel motor encoder in this event.
private
void
myJaguar_CustomSensorEvent(
object
sender,
EventArgs
e)
{
// front arm motor temperature
double
tempM =
Trans2Temperature((
double
)myJaguar.GetCustomAD5());
tempM =
double
.Parse(tempM.ToString(
"0.00"
));
lblTemp1.Text = tempM.ToString(
"0.00"
);