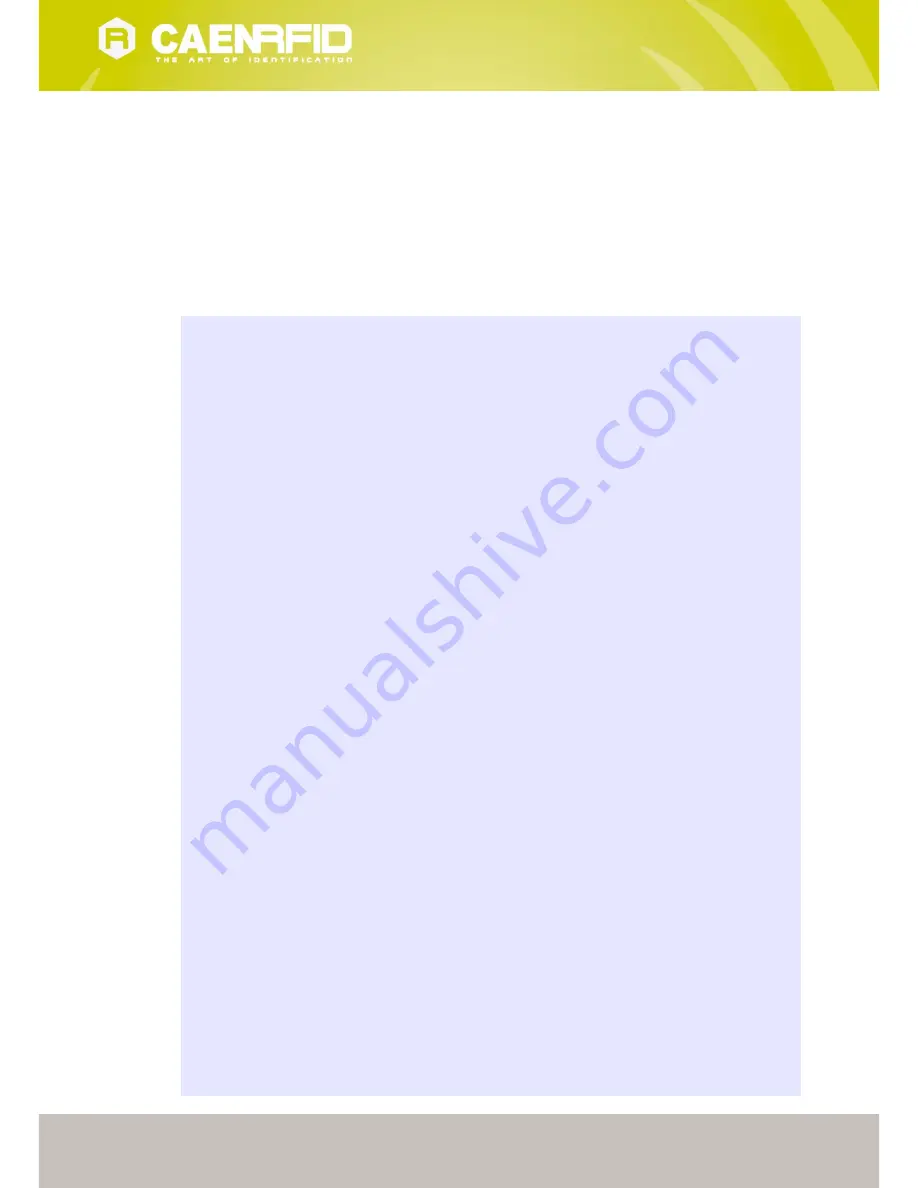
ION GETTING STARTED WITH C
- Ion Technical Information Manual
38
New API usage example
On the contrary to the standard API, only three functions are required to perform a basic inventory cycle using the new
API:
caenrfid_open(),
caenrfid_inventory()
caenrfid_close()
The code below shows how to use them.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
#include <caenrfid.h>
.......................
int main(int argc, char *argv[])
{
int i;
struct caenrfid_handle handle;
char string[] = "Source_0";
struct caenrfid_tag *tag;
size_t size;
char *str;
int ret;
if (argc < 2) {
fprintf(stderr, "usage: %s: <server_addr>\n", argv[0]);
exit(EXIT_FAILURE);
}
/* Start a new connection with the CAENRFIDD server */
ret = caenrfid_open(CAENRFID_PORT_TCP, argv[1], &handle);
if (ret < 0) {
fprintf(stderr, "cannot init lib (err=%d)\n", ret);
exit(EXIT_FAILURE);
}
/* Do the inventory */
ret = caenrfid_inventory(&handle, string, &tag, &size);
if (ret < 0) {
fprintf(stderr, "cannot get data (err=%d)\n", ret);
exit(EXIT_FAILURE);
}
/* Report results */
for (i = 0; i < size; i++) {
str = bin2hex(tag[i].id, tag[i].len);
if (!str) {
fprintf(stderr, "cannot allocate memory!\n");
exit(EXIT_FAILURE);
}
printf("%.*s %.*s %.*s %d\n",
tag[i].len * 2, str,
CAENRFID_SOURCE_NAME_LEN, tag[i].source,
CAENRFID_READPOINT_NAME_LEN, tag[i].readpoint,
tag[i].type);
free(str);
}
/* Free inventory data */
free(tag);