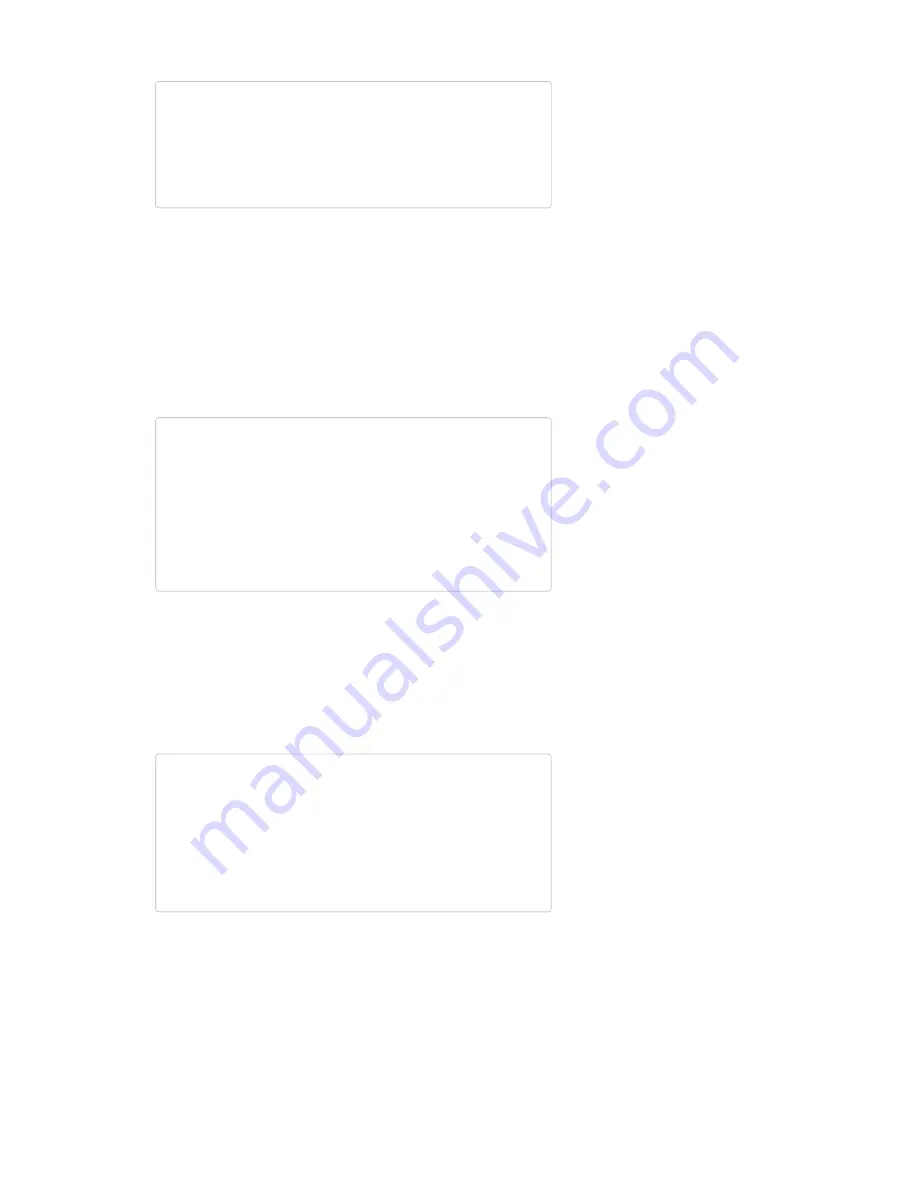
const char sparkfunDomain[]
= "sparkfun.com";
//
Domain
we
wan
t
to
look
up
IPAddress
sparkfunIP;
//
IPAddress
variable
where
we'll
store
the
domain's
IP
gprs.hostByName(sparkfunDomain,
&sparkfunIP);
Serial.print("sparkfun.com's
IP
address
is:
");
Serial.println(sparkfunIP);
hostByName()
returns an error code as well. It’ll be a positive number if the
lookup was successful, and a negative number if there was an error or
timeout.
Connecting
To connect to a remote IP address, use either
gprs.connect(IPAddress
ip,
unsigned
int
port)
or
gprs.connect(const
char
*
domain,
unsigned
int
port)
to connect to
an IP address or domain string.
For example, to connect to SparkFun on port 80 (the good, old, HTTP port),
send this:
const char sparkFunDomain[]
= "sparkfun.com";
int connectStatus;
connectStatus
= gprs.connect(sparkFunDomain,
80);
if (connectStatus
> 0)
Serial.println("Connected
to
SparkFun,
port
80");
else if (connectStatus
==
1)
Serial.println("Timed
out
trying
to
connect
to
SparkFu
n.");
else if (connectStatus
==
2)
Serial.println("Received
an
error
trying
to
connect.");
Sending and Receiving
As with other stream classes,
print()
,
write()
,
available()
, and
read()
functions are defined for
gprs
. You can use
print()
to send just
about any, defined variable type in Arduino to a connected server.
Each
print()
takes a long-ish time to execute (about 1-2 seconds), so if
speed is a factor we recommend using as few separate
print()
calls as
possible. For example, these blocks of code do the same thing:
//
You
can
do
this
to
send
a
GET
string..
gprs.println("GET
/
HTTP/1.1");
gprs.print("Host:
");
gprs.print(server);
gprs.println();
gprs.println();
//
But
this
one
is
faster:
gprs.print("GET
/
HTTP/1.1\nHost:
example.com\n\n");
But the latter finishes about 8 seconds faster.
To check for data coming from a connected server, back to the MG2639,
use
gprs.available()
and
gprs.read()
.
gprs.available()
returns the
number of bytes currently stored in the library’s receive buffer. If it’s greater
than 0, there’s at least one character there – use
gprs.read()
to read the
first available character in the buffer.
As an example from the sketch:
Page 17 of 22