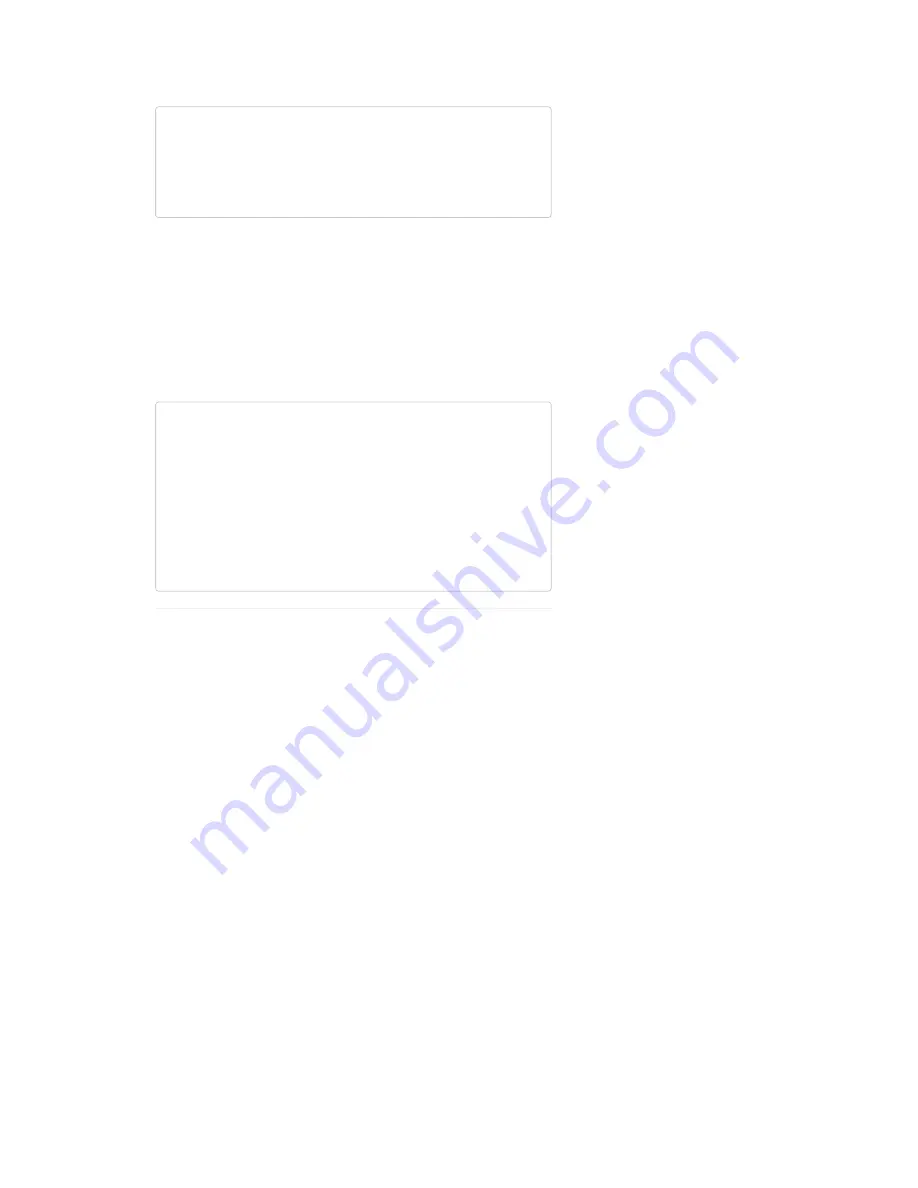
Here’s an example usage:
sms.start(sms.getSender());
sms.print("Analog 0 is: ");
sms.print(analogRead(A0));
int8_t status = sms.send();
if (status > 0)
Serial.println("SMS successfully sent");
Deleting a Stored SMS
Due to limitations of the library and the Arduino’s memory, we can only
keep track of so many (256 by default) unread messages. If you can
stomach letting them go, we recommend using the
deleteMessage(index)
function to remove a message from a SIM card’s memory whenever
possible.
To use the
deleteMessage()
function, simply supply the index of your
message as a parameter. For example, to delete a message after you’ve
read it:
// Get first available unread message:
int messageIndex = sms.available(REC_UNREAD);
// If an index was returned, proceed to read that message:
if (messageIndex > 0)
{
// Call sms.read(index) to read the message:
sms.read(messageIndex);
Serial.println(sms.getMessage());
// Delete the message after reading:
sms.deleteMessage(i);
}
A second example in the library –
MG2639_SMS_Read
– allows you to go
through the logs of your text messages and delete any or save them.
Example 2: GPRS & TCP
One of the most powerful aspects of the MG2639 is its ability to connect to
a GPRS network and interact with the Internet. The module supports
TCP/IP, DNS lookup, and most of the features you’d expect from a similar
WiFi or Ethernet shield.
There are a couple examples demonstrating GPRS and TCP in the
SFE_MG2639_CellularShield library. Open up the general example –
MG2639_GPRS
– by going to File > Examples >
SFE_MG2639_CellularShield > MG2639_GPRS.
Running the Example
This example demonstrates how to turn the MG2639 into a simple browser
client. It shows off most of the GPRS functions, including enabling GPRS,
finding local and remote IP addresses, connecting to a server, and
sending/receiving data.
A char array near the top of the sketch –
const char server[] = "example.com";
– defines the remote URL your
shield will try to connect to.
As with the other sketches, after uploading the sketch, run it by opening the
serial monitor and sending any character. The MG2639 will open GPRS,
find its IP, find the destination IP and send an HTTP GET request.
Page 15 of 22