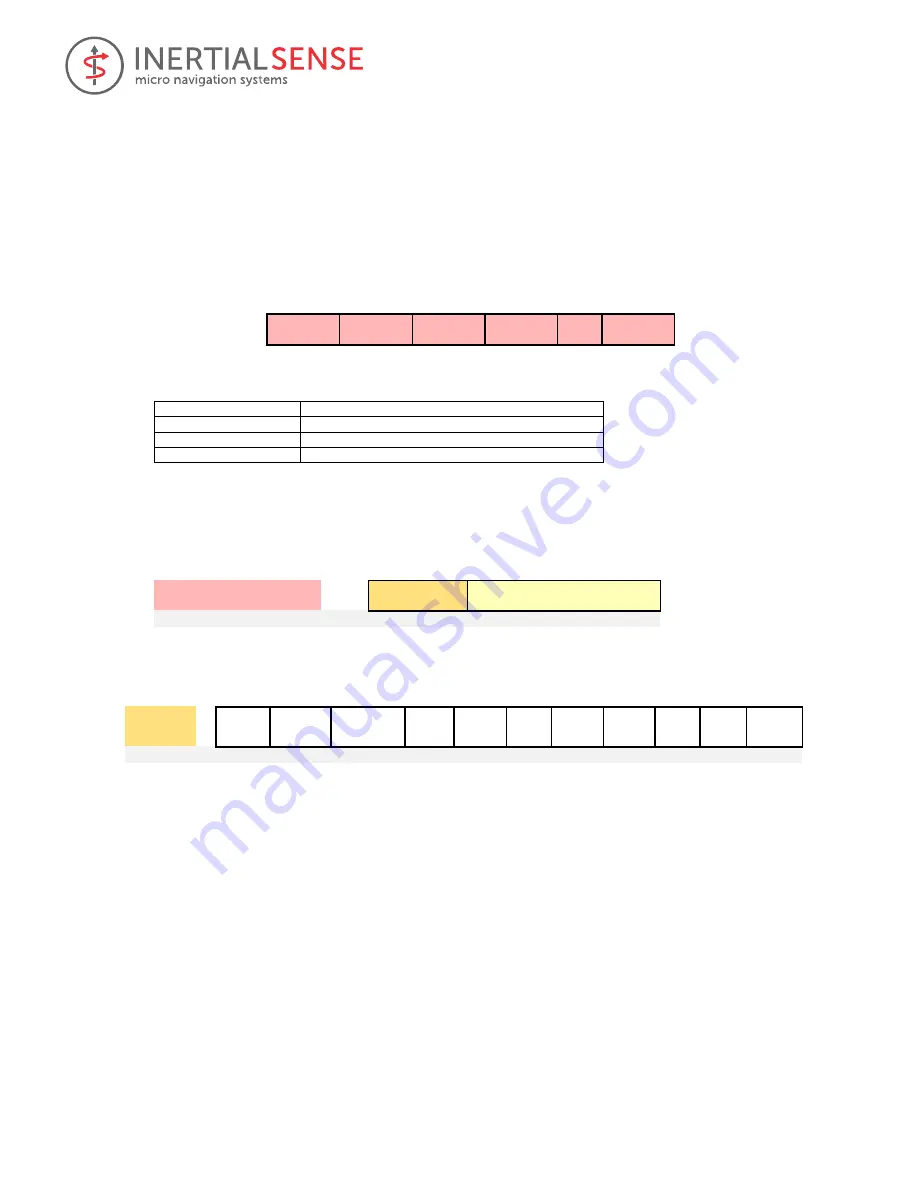
User Manual
© 2017 Inertial Sense, LLC
26
11/30/2017
9
Data Logging
Inertial Sense provides data loggers written in C++ , which can read and write data in various formats to file. These
loggers are useful for storing, replaying, and analyzing data. SDK/src/ISLogger.h defines the logging interface.
9.1
Log File
The data log file name has the format
LOG_SNXXXXX_YYYYMMDD_HHMMSS_CNT.dat
which contains the device
serial number, date, time, and log file count. The two primary log file formats are .dat and .sdat. These log files
consist of a series of data Chunks.
Data Log File
(.dat or .sdat)
=
CHUNK
1
CHUNK
2
CHUNK
3
CHUNK
4
…
CHUNK
N
Standard data types are stored in the log files and are defined as:
U32
unsigned int
U16
unsigned short
S8
char
U8
unsigned char
9.1.1
Log Chunk
The data log file is composed of Chunks. A Chunk is a data container that provides an efficient method for
organizing, handling, and parsing data in a file. A Chunk starts with a header which has a unique identifiable
marker and ends with the data to be stored.
CHUNK
=
CHUNK
Header
CHUNK
Data
Byte Size
40
9.1.2
Chunk Header
The header, found at the start of each Chunk, is as follows:
CHUNK
Header
=
Marker
Version
Classification
Name
Name
Inverted
Data
Size
Data
Size
Inverted
Group
Number
Device
Serial
#
Port
Handle
Reserved
Byte Size
4
2
2
4
4
4
4
4
4
4
4
Data Type
U32
U16
U16
S8 (x4)
S8 (x4)
U32
U32
U32
U32
U32
U32
The C structure implementation of the Chunk header is:
//!< Chunk Header
#pragma pack
(
push
, 1)
struct
sChunkHeader
{
Unsigned int
marker
;
//!< Chunk marker (0xFC05EA32)
unsigned short
version
;
//!< Chunk Version
unsigned short
classification
;
//!< Chunk classification
char
name
[4];
//!< Chunk name
char
invName
[4];
//!< Bitwise inverse of chunk name
unsigned int
dataSize
;
//!< Chunk data length in bytes
unsigned int
invDataSize
;
//!< Bitwise inverse of chunk data length
unsigned int
grpNum
;
//!< Chunk group number: 0=serial data, 1=sorted data
unsigned int
devSerialNum
;
//!< Device serial number
unsigned int
pHandle
;
//!< Device port handle
unsigned int
reserved
;
//!< Unused
};
#pragma pack
(
pop
)