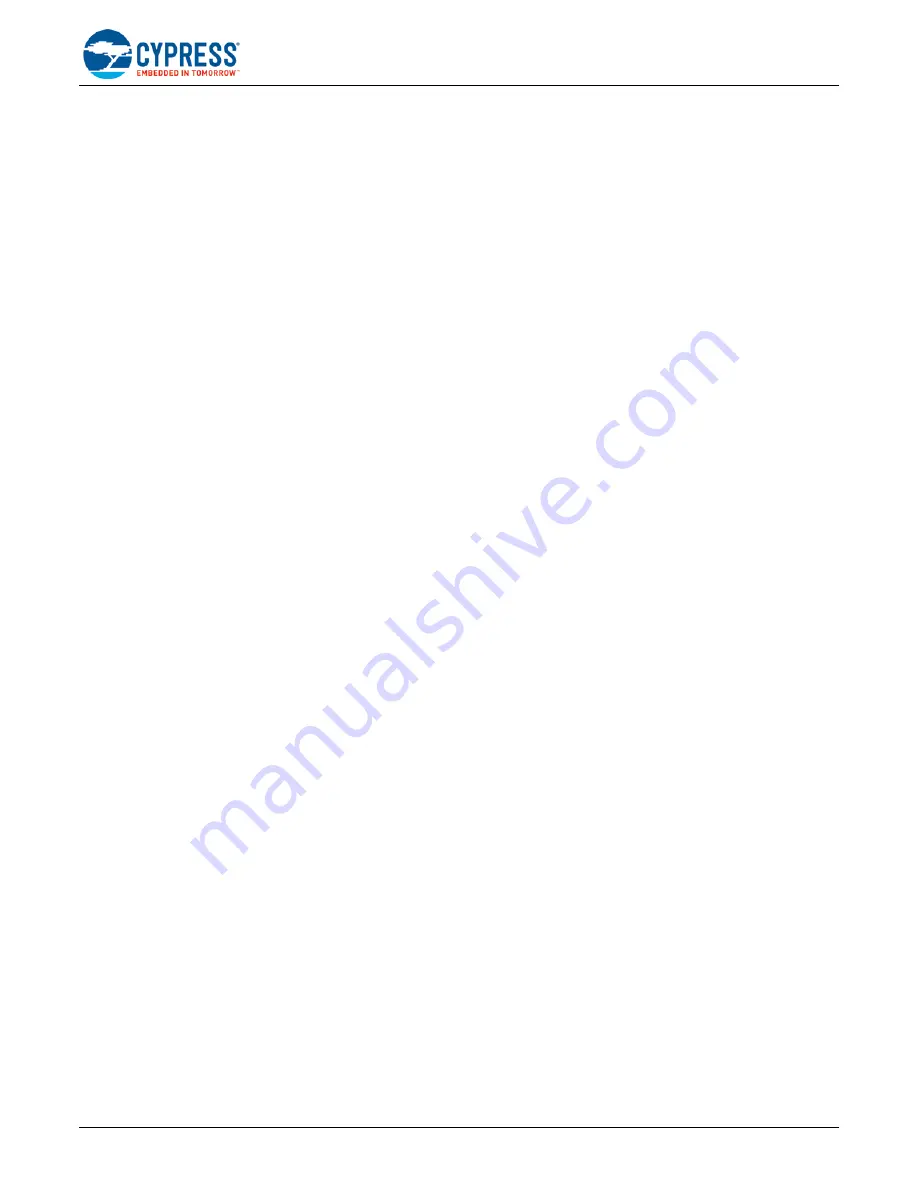
Interface Programming Information and Examples
CYW920706WCDEVAL Hardware User Guide Doc. No.: 002-16535 Rev. **
39
wiced_hal_pspi_init( MASTER, GPIO_PULL_UP, MASTER1_P24_CLK_P27_MOSI_P25_MISO,
SPIFFY_SPEED, SPI_MSB_FIRST, SPI_SS_ACTIVE_LOW, SPI_MODE_3, WICED_GPIO_33 );
/* Send a byte and receive a byte from slave*/
test_spiffy1_master_send_receive_byte( 1 );
}
/* Sends one byte and receives one byte from the SPI slave.
* byteToSend - The byte to send to the slave.
* Returns the byte received from the slave.
*/
uint8_t test_spiffy1_master_send_receive_byte( uint8_t byteToSend )
{
uint8_t byteReceived;
/* Assert chipselect by driving O/P on the GPIO */
wiced_hal_gpio_set_pin_output( WICED_GPIO_15, SPIFFY_CS_ASSERT);
/* Tx one byte of data */
wiced_hal_pspi_tx_data( 1, &byteToSend );
/* Rx one byte of data */
wiced_hal_pspi_rx_data( 1, &byteReceived );
/* Deassert chipselect */
wiced_hal_gpio_set_pin_output( WICED_GPIO_15, SPIFFY_CS_DEASSSERT );
}
10.3 SPI1 Slave Programming Example
The following code shows how to initialize SPI1 as a slave, write a byte to a SPI master, and read a byte from a SPI master.
#include "wiced_hal_pspi.h"
#define SLAVE1_P36_CS_P24_CLK_P27_MOSI_P25_MISO 0x24181b19 /* Refer Hardware
peripherals doc */
#define SPIFFY_SPEED 1000000 /* Use 1M speed */
void test_pspi_driver( void )
{
/* Initialize spiffy1 in slave role, available pin combinations are in spiffydriver.h
DO NOT CONFIGURE csPin in SLAVE mode - the HW takes care of this. There is no need
to configure the speed too - the master selects the speed.
*/
wiced_hal_pspi_init( SLAVE, GPIO_PULL_DOWN,
SLAVE1_P36_CS_P24_CLK_P27_MOSI_P25_MISO,
SPIFFY_SPEED, SPI_MSB_FIRST,
SPI_SS_ACTIVE_LOW, SPI_MODE_3,
WICED_GPIO_03 );
/* Rx a byte from master, increment and Tx it back to master*/
test_spiffy1_slave_send_receive_byte( );
}
/* Receives a byte from the SPI master, increments the byte and sends it back
* byteToSend - The byte to send to the slave.
* Returns the byte received from the slave.