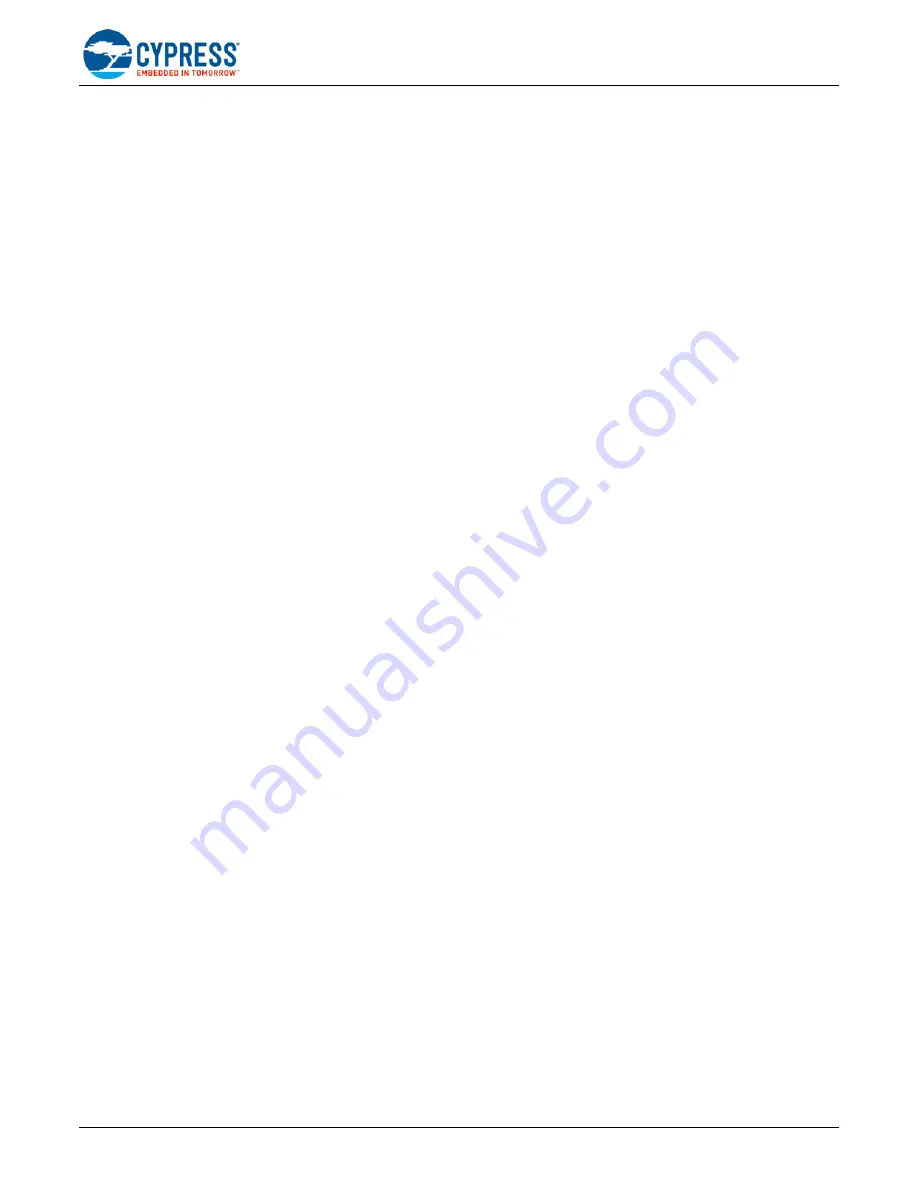
Interface Programming Information and Examples
CYW920706WCDEVAL Hardware User Guide Doc. No.: 002-16535 Rev. **
38
wiced_hal_gpio_register_pin_for_interrupt( WICED_GPIO_BUTTON,
gpio_interrrupt_handler, NULL );
/* Get the pin configuration set above */
pin_config = wiced_hal_gpio_get_pin_config( WICED_GPIO_BUTTON );
WICED_BT_TRACE( "Pin config of P%d is %d\n\r", WICED_GPIO_BUTTON, pin_config );
}
/* The function invoked on timeout of app seconds timer. */
void seconds_app_timer_cb( uint32_t arg )
{
wiced_time+;
WICED_BT_TRACE( "seconds periodic timer count: %d s\n", wiced_timer_count );
if(wiced_timer_count & 1)
{
wiced_hal_gpio_set_pin_output( LED_GPIO_1, GPIO_PIN_OUTPUT_LOW);
}
else
{
wiced_hal_gpio_set_pin_output( LED_GPIO_1, GPIO_PIN_OUTPUT_HIGH);
}
}
void gpio_test_led( )
{
WICED_BT_TRACE( "gpio_test_led\n\r" );
/* Configure LED PIN as input and initial outvalue as high */
wiced_hal_gpio_configure_pin( LED_GPIO_1, GPIO_OUTPUT_ENABLE, GPIO_PIN_OUTPUT_HIGH );
if ( wiced_init_timer( &seconds_timer, &seconds_app_timer_cb, 0,
WICED_SECONDS_PERIODIC_TIMER )== WICED_SUCCESS )
{
if ( wiced_start_timer( &seconds_timer, APP_TIMEOUT_IN_SECONDS_A )
!= WICED_SUCCESS )
{
WICED_BT_TRACE( "Seconds Timer Error\n\r" );
}
}
}
10.2 SPI1 Master Programming Example
The following code shows how to initialize SPI1 as a master, write a byte to a SPI slave, and read a byte from a SPI slave.
#include "wiced_hal_gpio.h"
#include "wiced_hal_pspi.h"
#define SPIFFY_SPEED 1000000 /* Use 1M speed */
#define SPIFFY_CS_ASSERT 1
#define SPIFFY_CS_DEASSSERT 0
uint8_t test_spiffy1_master_send_receive_byte( uint8_t byteToSend );
void test_pspi_driver( void )
{
/* Reset spi hardware block and set to default config*/
wiced_hal_pspi_reset( );
/* Initialize spiffy1 in master role */
/* available pin combinations are in spiffydriver.h */