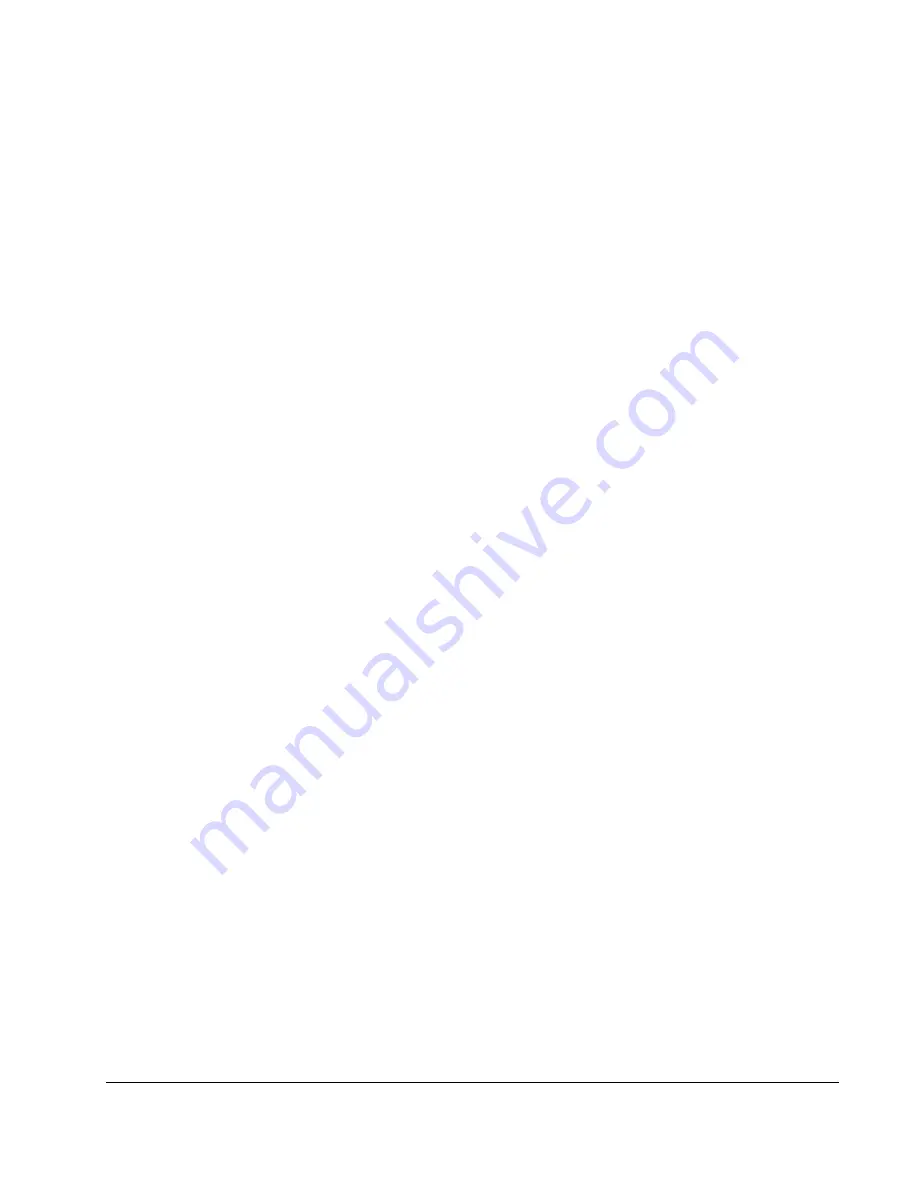
SB AWE32 Developer's Information Pack
PART III Windows Driver API
••
79
Copyright
Creative Technology Ltd., 1994-1996
Version 3.00
Retrieving selections
The 'Get' class API provided by the Manager allows you to retrieve the AWE's current selection. You
can retrieve the current in use selection from Effect Types and Variations. For Synthesizer Bank, User
Bank and Instruments in the bank, these API will be used to retrieve their descriptors.
The following set of functions retrieve the current selection and updates the global variables. This
example can be added to your program.
#include "string.h"
#include "windows.h"
#include "AWE_DLL.H"
#define BUF_SIZE 255
AWEHANDLE hID;
enum SBANK m_CurSynthBank; // Current synthesizer bank
enum TYPEINDEX m_CurEfxType; // Current effects type
enum VARIINDEX m_CurTypeVari[6]; // Current type variations
char cUBankDescriptor[30]; // User Bank Descriptor
void GetCurrentSelection( void )
//
// Retrieve the current Hardware Settings
//
{
CParamObject param;
CBufferObject buffer;
char scratch;
// Get current Synthesizer Bank from AWEMAN
buffer.m_Size = sizeof(scratch);
buffer.m_Buffer = (LPSTR)&scratch;
AWEManager(hID,AWE_GET_SYN_BANK,(LPARAM)(LPBUFFEROBJECT)&buffer,0L);
// should return an error, since buffer not enough
// Ignore error, since we only want m_Flag => SBANK INDEX
m_CurSynthBank = (enum SBANK)buffer.m_Flag;
// Get Current Effects Type and Variations
param.m_VariIndex[REVERB] = param.m_VariIndex[CHORUS] = 0;
AWEManager(hID, AWE_GET_EFX_EX, (LPARAM)(LPPARAMOBJECT)¶m,0L);
m_CurEfxType = (enum TYPEINDEX) param.m_TypeIndex;
m_CurTypeVari[0] = (enum VARIINDEX) param.m_VariIndex[0];
m_CurTypeVari[1] = (enum VARIINDEX) param.m_VariIndex[1];
m_CurTypeVari[2] = (enum VARIINDEX) param.m_VariIndex[2];
m_CurTypeVari[3] = (enum VARIINDEX) param.m_VariIndex[3];
m_CurTypeVari[4] = (enum VARIINDEX) param.m_VariIndex[4];
m_CurTypeVari[5] = (enum VARIINDEX) param.m_VariIndex[5];
}
void GetUBankDescriptor( int nUBNum )
//
// Retrieve the User Bank's Descriptor.
//
{
char scratch[BUF_SIZE];
CBufferObject buffer;
buffer.m_Size = BUF_SIZE;
buffer.m_Buffer = (LPSTR)&scratch;
AWEManager(hID, AWE_GET_USER_BANK, (LPARAM)(WORD) nUBNum,
(LPARAM)(LPBUFFEROBJECT) &buffer);
if (buffer.m_SizeUsed == 1)
strcpy((char *) &cUBankDescriptor[0],
(const char*)"NO DESCRIPTOR\0");
else
strcpy((char *) &cUBankDescriptor[0], (const char*) &scratch[0]);
}