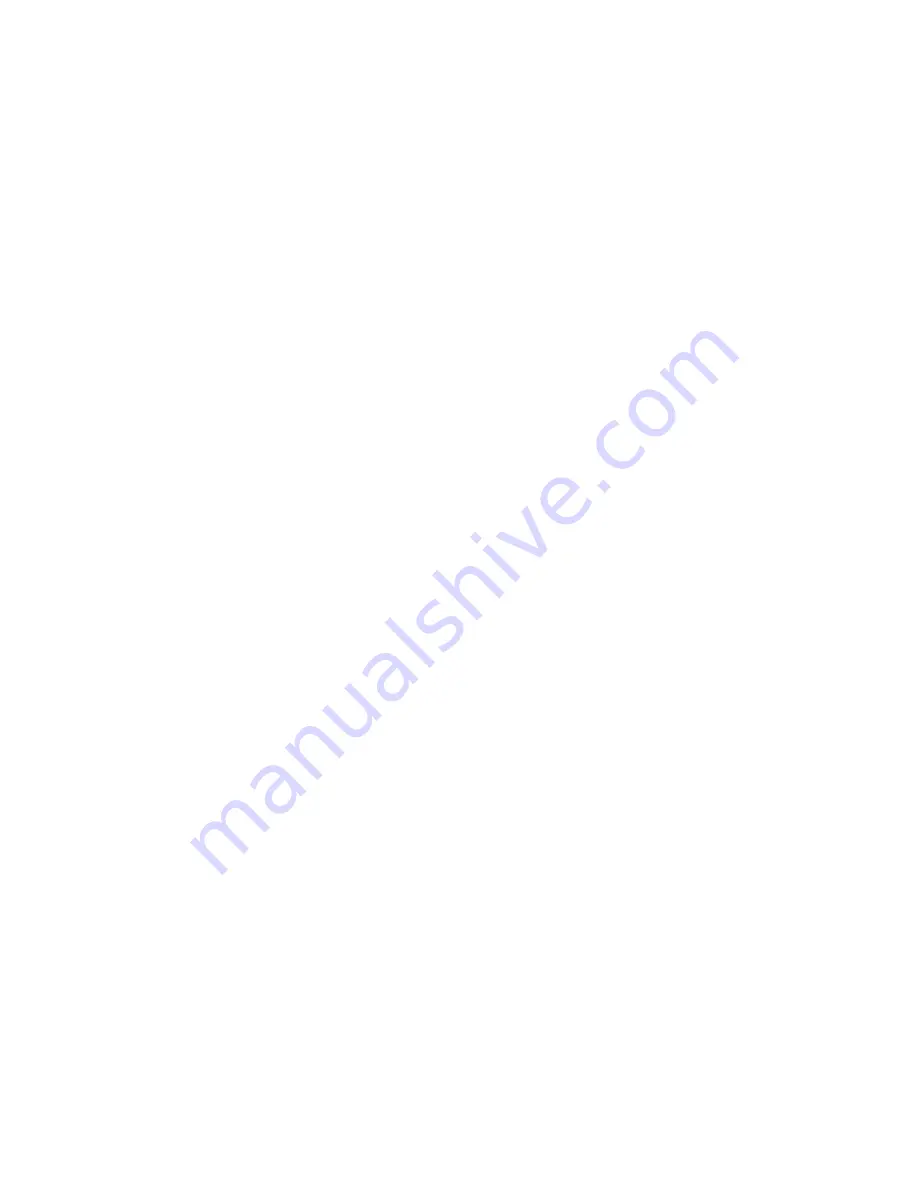
•
The data structure should consist of the minimum possible number of objects, especially when you use high-level objects
like a
Vector
or a
Hashtable
. These classes provide significant functionality but are not efficient storage mechanisms
and you should avoid using them in the persistent store if possible.
•
When possible, use primitives instead of objects, because primitives reduce the number of object handles that are consumed
on the BlackBerry device. An array of primitives is an object and consumes an object handle.
•
String
objects are as efficient as byte arrays. A
String
object consumes only one object handle and is equivalent if
your application stores all of the characters as a byte. In other words, the value of each character is less than or equal to
the decimal value of 255. If your application cannot store characters as a byte, you can store the characters as a
String
because it is equivalent to storing a
char
array.
Best practice: Consolidating objects into object groups
One of the most common errors that application developers encounter is an exhaustion of persistent object handles. The amount
of flash memory on the BlackBerry® device determines the fixed number of persistent object handles that are available in the
system. Depending on the data structure selection, stored records can quickly exhaust the number of persistent object handles.
A persistent object consumes a persistent object handle and an object handle. A transient object consumes only an object handle.
For example, a record that contains ten
String
fields, which represent items like a name, a phone number, and an address,
consumes 11 persistent object handles, one for the record object and one for each
String
. If a BlackBerry® Java Application
persists 3000 records, the application consumes 33,000 persistent object handles, which exceeds the number of persistent object
handles available on a BlackBerry device with 16 MB of flash memory.
You can use the
net.rim.device.api.system.ObjectGroup
class to consolidate the object handles for an object
into one group. Using the example in the previous paragraph, if you group the record, the record consumes one persistent object
handle instead of 11. The object handles for the
String
fields consolidate under the record object handle.
When you consolidate object handles into one group, the object handle is read-only. You must ungroup the object before you
can change it. After you complete the changes, group the object again. If you attempt to change a grouped object without first
ungrouping it, an
ObjectGroupReadOnlyException
is thrown.
Ungrouping an object has a performance impact. The system creates a copy of the grouped object and allocates handles to each
of the objects inside that group. Therefore, objects should only be ungrouped when necessary.
Fundamentals Guide
Conserving resources
26