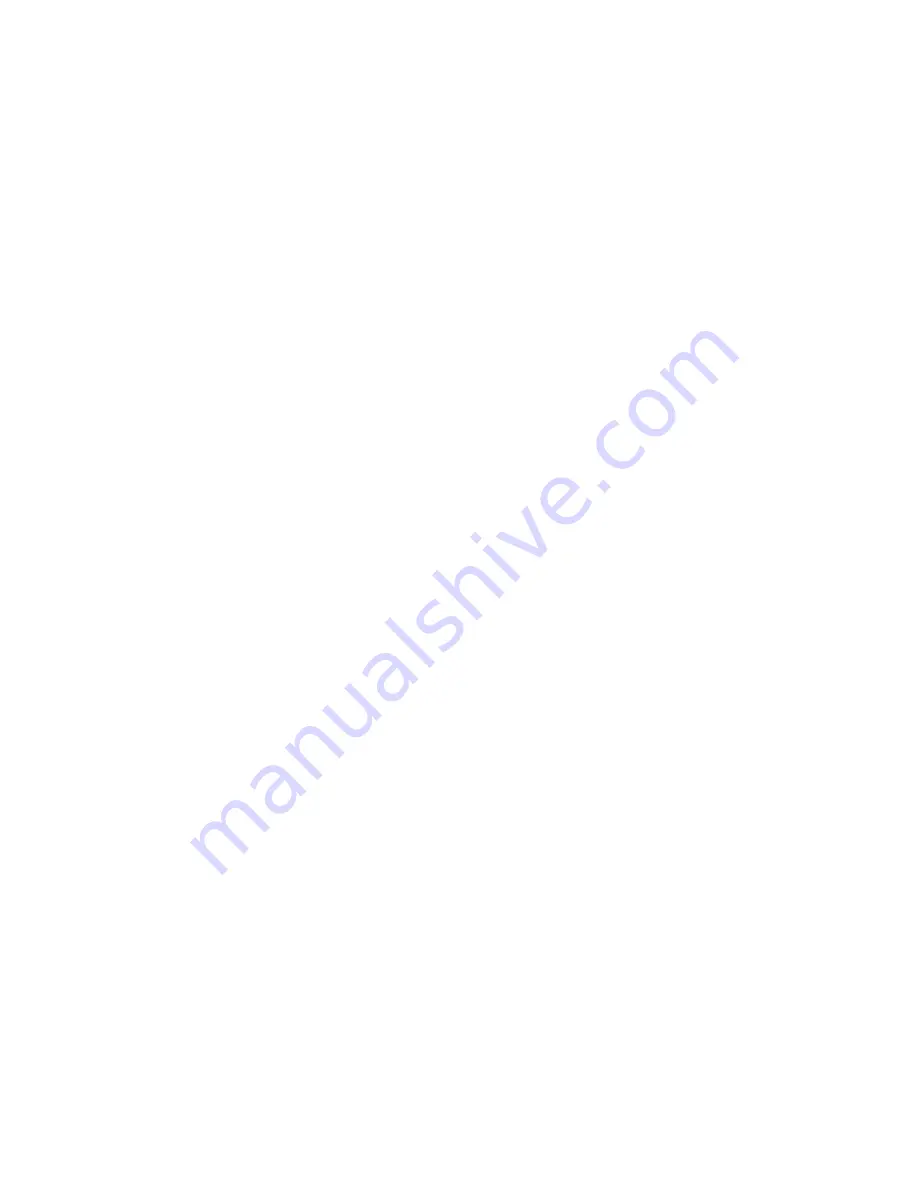
Making classes final
When you create code libraries, mark classes as
final
if you know that developers will never extend them. The presence of the
final
keyword allows the compiler to generate more efficient code.
By default, the BlackBerry® Java® Development Environment compiler marks any classes that you do not extend in an
application .cod file as
final
.
Using int instead of long
In Java®, a
long
is a 64-bit integer. Because BlackBerry® devices use a 32-bit processor, operations can run two to four times
faster if you use an
int
instead of a
long
.
Avoiding garbage collection
Avoid calling
System.gc()
to perform a garbage collection operation because it might take too much time on
BlackBerry® devices with limited available memory. Let the BlackBerry® Java® Virtual Machine collect garbage.
Using static variables for Strings
When you define static fields (also called class fields) of type
String
, you can increase application speed by using static
variables (not
final
) instead of constants (
final
). The opposite is true for primitive data types, such as
int
.
For example, you might create a
String
object as follows:
private static final String x ="example";
For this static constant (denoted by the
final
keyword), each time that you use the constant, a temporary
String
instance
is created. The compiler eliminates
"x"
and replaces it with the string
"example"
in the bytecode, so that the
BlackBerry® Java® Virtual Machine performs a hash table lookup each time that you reference
"x"
.
In contrast, for a static variable (no
final
keyword), the
String
is created once. The BlackBerry JVM performs the hash table
lookup only when it initializes
"x"
, so access is faster.
private static String x = "example";
You can use public constants (that is,
final
fields), but you must mark variables as private.
Avoiding the String(String) constructor
In a BlackBerry® Java Application, each quoted string is an instance of the
java.lang.String
class. Create a
String
without using the
java.lang.String(String)
constructor.
Fundamentals Guide
Best practices for writing an efficient BlackBerry Java Application
14