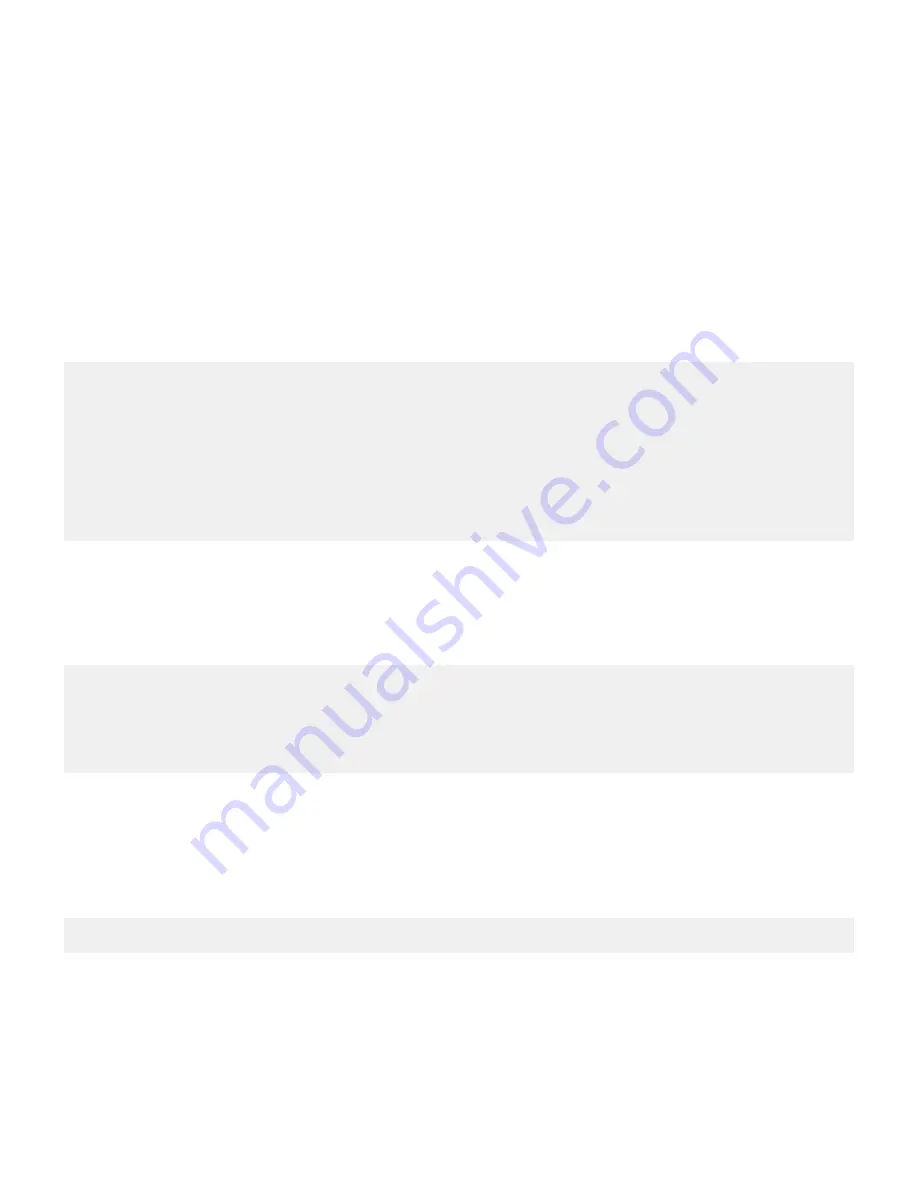
Avoiding java.util.Enumeration
Avoid using
java.util.Enumeration
objects unless you want to hide data (in other words, to return an enumeration
of the data instead of the data itself). Asking a vector or hash table for an
Enumeration
object is slow and creates unnecessary
garbage. If another thread might modify the vector, synchronize the iteration. The Java® SE uses an
Iterator
object for
similar operations, but
Iterator
objects are not available in the Java® ME.
Code sample
for( int i = v.size() - 1; i >=0; --i ) {
o = v.elementAt( i );
...
}
synchronized( v ) {
for( int i = v.size() - 1; i >=0; --i ) {
o = v.elementAt( i );
...
}
}
Performing casts using instanceof
Use
instanceof
to evaluate whether a cast succeeds.
Code sample
if( x instanceof String ) {
(String)x.whatever();
} else {
...
}
x
Evaluating conditions using instanceof
To produce smaller and faster code, if you evaluate a condition using
instanceof
, do not evaluate explicitly whether the
variable is null.
Code sample
if( e instanceof ExampleClass ) { ... }
if( ! ( e instanceof ExampleClass ) ) { ... }
Fundamentals Guide
Best practices for writing an efficient BlackBerry Java Application
16