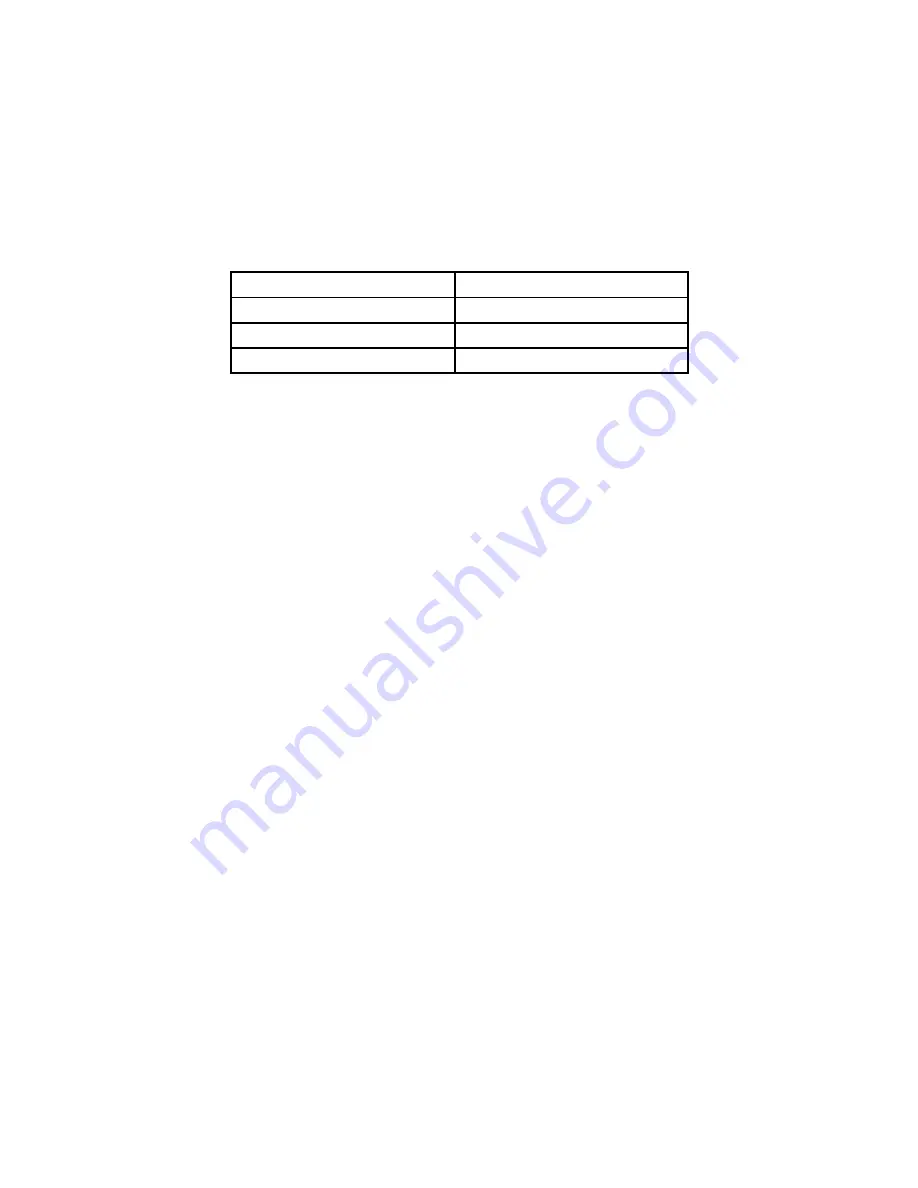
Logical-Not (!) Operator
The logical-not is a prefix operator that “negates” the Boolean expression that follows it. In other words,
if
Expression1
returns true (
1
),
!Expression1
returns false (
0
).
For example:
This expression...
... resolves to this value.
!0
1
!(4==3)
1
!(“Hello”==“Hello”)
0
Bitwise-And (&) Operator
The bitwise-AND operator performs the logical AND operation bit by bit between two integers. This is
better understood by looking at the example of
12&10. The binary value of 12 is 1100,
and the binary value of 10 is 1010. 12&10 is computed by performing
an AND between each of the corresponding four bits:
12:
1
1
0
0
10:
1
0
1
0
12&10:
1
0
0
0
Therefore:
12&10 = (Binary) 1000 = (Decimal) 8
Bitwise-Or (|) Operator
The bitwise-OR operator performs the logical OR operation bit by bit between two integers. This is
better understood by looking at the example of
12|10. The binary value of 12 is 1100,
and the binary value of 10 is 1010. 12|10 is computed by performing
an OR between each of the corresponding four bits:
12:
1
1
0
0
10:
1
0
1
0
12|10:
1
1
1
0
Therefore:
12|10 = (Binary) 1110 = (Decimal) 14
One's Complement (~) Operator
The one's complement operator is a bitwise-not prefix operator that performs a logical NOT to each bit of
an integer. This is better understood by looking at the example of
~5. The binary value of 5
is 101. ~5 is computed by performing a NOT on each of the three bits: