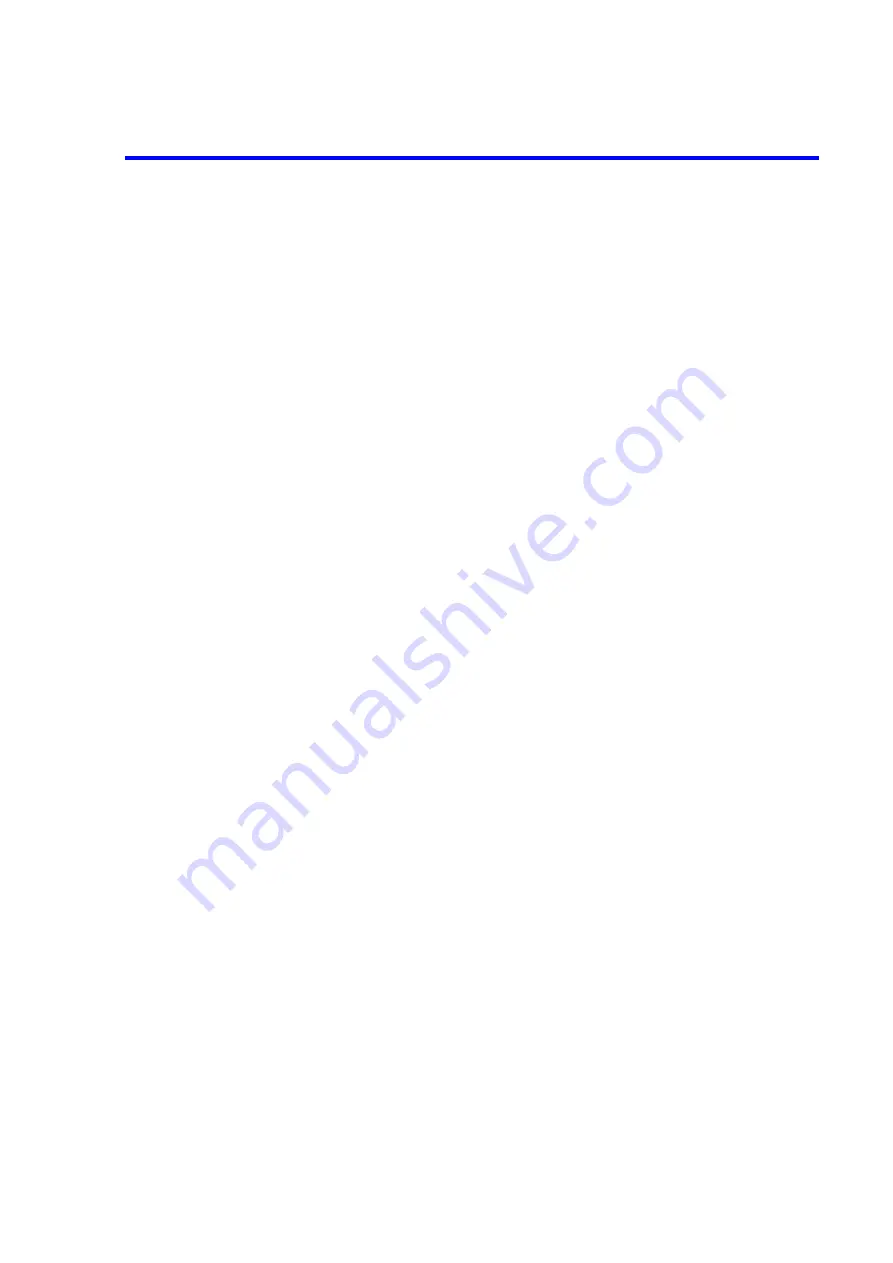
7352 Series Digital Multimeter Operation Manual
6.7.2 Sample Programs (SCPI commands for GPIB)
6-58
Example 2 Sets the DCV-Ach 2 V range for the left side display and the ACV-Ach 2 V range for the right
side display, specifies BUS as the trigger source, sets the sample count to 10, sets the mea-
surement data memory to ON, and then triggers to start the measurement. Detects the mea-
surement end by the SRQ interruption, reads the measurement memory data from the 7352A,
and then displays the data in cells.
Dim DMM_ADR As Integer
'Declares the GPIB address variable of the 7352A.
Dim dmm As Integer
'Declares the variable of the device descriptor.
Dim dt As String * 200
'Declares the variable of the buffer used for receiving the GPIB data.
Dim s As Integer
'The variable in which the serial polling result is stored
DMM_ADR = 1
'GPIB address of the 7352A
Call ibdev(0, DMM_ADR, 0, T10s, 1, 0, dmm)
'Initializes the GPIB I/F.
Call ibconfig(dmm, IbcUnAddr, 1)
'Sets the transmitting and receiving addresses individually.
Call ibconfig(0, IbcAUTOPOLL, 0)
'Disables the automatic serial polling.
Call ibwrt(dmm, "*RST" & vbLf)
'Initializes the 7352A.
Call ibwrt(dmm, ":SENSE:FUNCTION 'VOLTAGE:DC',(@1)" & vbLf)
'Sets the measurement function for the left side display to DCV.
Call ibwrt(dmm, ":SENSE:VOLTAGE:DC:RANGE 1.99999,(@1)" & vbLf)
'Sets the measurement range for the left side display to 2 V.
Call ibwrt(dmm, ":SENSE:VOLTAGE:DC:SRATE MED" & vbLf)
'Sets the sampling rate to MED.
Call ibwrt(dmm, ":SENSE:FUNCTION 'VOLTAGE:AC',(@2) & vbLf)
'Sets the measurement function for the right side display to ACV.
Call ibwrt(dmm, ":SENSE:VOLTAGE:AC:RANGE 1.99999,(@2) & vbLf)
'Sets the measurement range for the right side display to 2 V.
Call ibwrt(dmm, ":SENSE:VOLTAGE:AC:SRATE MED" & vbLf)
'Sets the sampling rate to MED.
Call ibwrt(dmm, ":SAMPLE:COUNT 10" & vbLf)
'Sets the sampling count to 10.
Call ibwrt(dmm, ":TRIGGER:SOURCE BUS" & vbLf)
'Sets the trigger source to "BUS".
Call ibwrt(dmm, ":INITIATE:CONTINUOUS ON" & vbLf)
'Sets CONTINUOUS ON.
Call ibwrt(dmm, ":TRACE:STATE ON" & vbLf)
'Enables the measurement memory.
Call ibwrt(dmm, ":TRACE:CLEAR" & vbLf)
'Clears the measurement memory.
Call ibwrt(dmm, "*CLS"" & vbLf)
'Clears the status byte.
Call ibwrt(dmm, ":STATUS:MEASUREMENT:ENABLE 256,(@2)" & vbLf)
'Sets the measurement end flag of MSR for the right side display.
Call ibwrt(dmm, "*SRE 1" & vbLf)
'Sets the measurement end flag of SRE.
Call ibwrt(dmm, "*TRG" & vbLf)
'Triggers.
Call ibwait(dmm, RQS Or TIMO)
'Waits for the SRQ transmission.
If (ibsta And TIMO) Then
'Judges whether a time-out occurs.
Call MsgBox("SRQ Time Out", vbOKOnly, "Error")
'Sends a message if a time-out occurs.
Call ibonl(dmm, 0)
'Terminates.
Exit Sub
Else
Call ibrsp(dmm, s)
'Reads the status byte.
End If