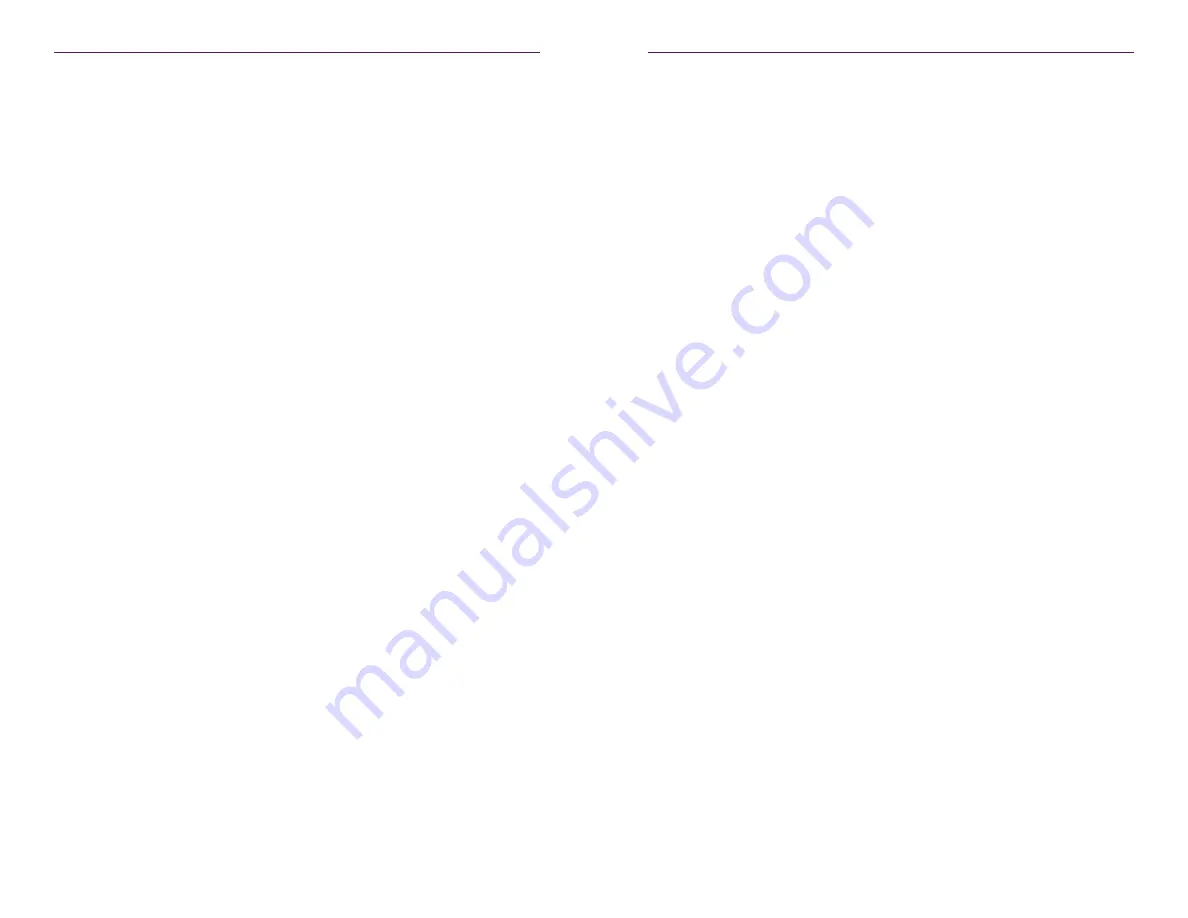
52
53
Advanced Operation
Command Request / Response
The following code excerpt sends an AJAX request to list all ZyPer encoders and decoders:
function zt(){
xmlhttp = new XMLHttpRequest();
xmlhttp.open(“POST”, url, true);
xmlhttp.setRequestHeader(“Content-Type”, “application/x-www-form-
urlencoded”);
xmlhttp.onreadystatechange = function(){
if (xmlhttp.readyState == 4 && xmlhttp.status == 200){
procResp(xmlhttp.responseText);
}
}
xmlhttp.send(encodeURIComponent(“commands:show device-status
all”));
}
In this example, the
encodeURIComponent
function has two parts: The request type,
which is
commands
and the command
show device-status all
. Refer to the
show device status
command for more information. Currently,
commands
is the
only request type that is supported and only a single command can be suppplied for each
request.
Here, we handle the AJAX response:
function procRespTest(jsonData){
var jsData = JSON.parse(jsonData);
# jsData.status may have the values:
# “Success”
# “Request failed authentication”
# “Server not running”
# “no commands provided”
#
if (jsData.status == “Success”){
var element = document.getElementById(“responseError”);
element.innerHTML = jsData.responses[0].error;
element = document.getElementById(“responseWarning”);
element.innerHTML = jsData.responses[0].warning;
element = document.getElementById(“numObjectsInResponse”);
element.innerHTML = jsData.responses[0].text.length;
}
else{
// Failed authentication
}
}
The JSON data is decoded using the
JSON.parse()
method. In this example, information
about the response data is displayed on the web page (HTML code not shown).
Advanced Operation
The JavaScript object that is returned is:
var jsObj = {
status: true | false;
responses: [ {error: “errorText”,
warning: “warningText”,
text: [ { param1: “val1”, parmN: “paramN” } ]
}
]
};
The return value is an object that contains two members:
status
and
responses
.
If the
status
member is not equal to “Success”, then the
responses
member is not
valid. If the request fails authentication, then the
status
value will be “Request failed
authentication”. Note that there may be other web-server level failures that can be returned
in the
status
string.
The second member in the returned object,
responses
, which is an array of objects.
Each of these objects contains three members:
error
,
warning
, and
text
. The
error
and
warning
members are strings. The
text
member is an array of objects with the
desired parameters and values. If the
error
string is non-null, then the
warning
and
text
members will be null. If
text
is non-null, then the
warning
string may still be valid.
Currently, the
responses
member is always an array size of 1.