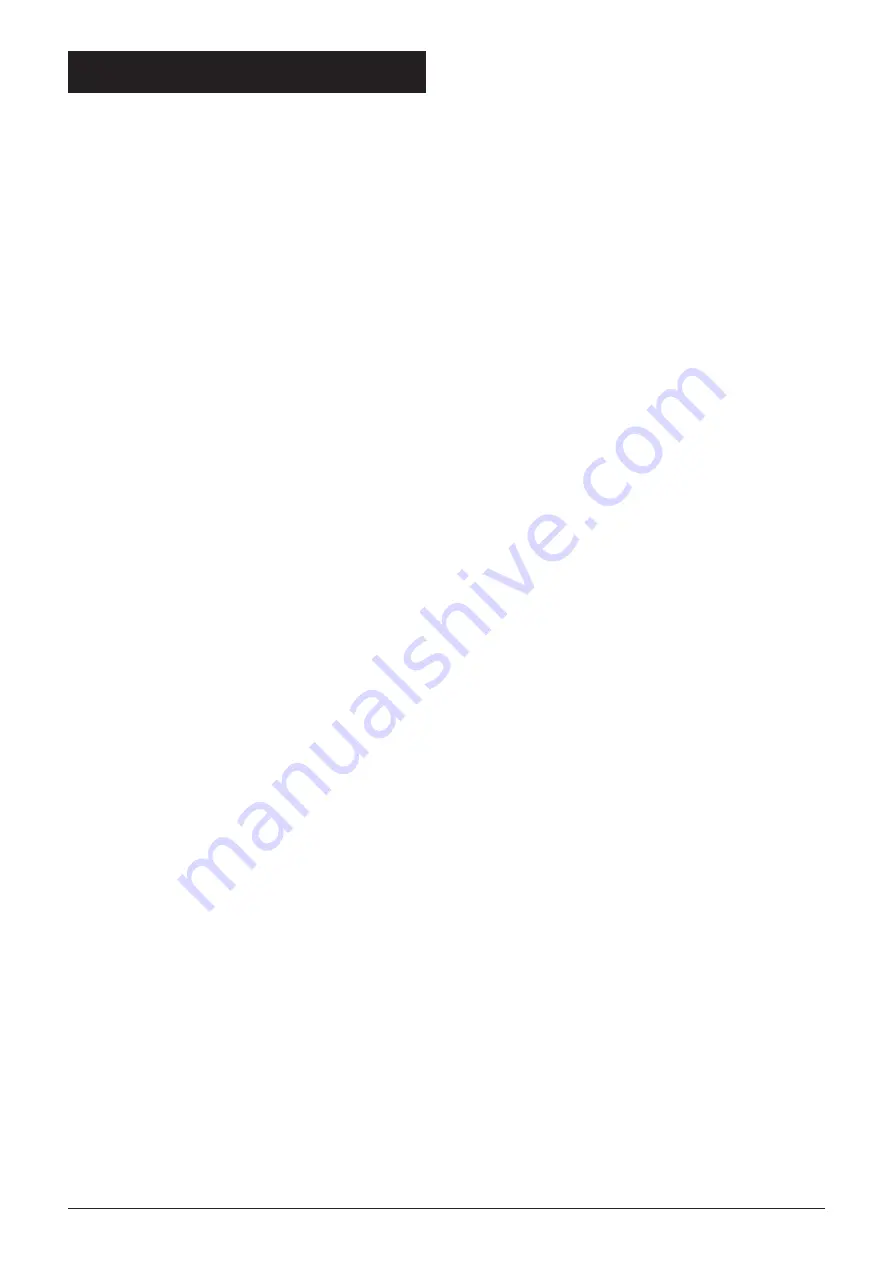
5
IM 77J01A0E11-01E
8th Edition
6. EXAMPLE OF COMMUNICATION
PROGRAM
This chapter shows a sample program to read/write data
operating by Microsoft Visual Basic 6.0. The program shown
here is for your reference when you create a program. All
operation is not guaranteed.
’/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
’
’Example of VJET Communication Procedure by Microsoft Visual Basic
6.0
’
’
’Procedure to read the input value of VJU7 (Address: 01) via VJET from
LAN port of a PC.
’Connect and send command by Connect, SendData of Winsock control,
and receice data by DataArrival event.
’Procedure to connect to VJET by TCP/IP, create command and send the
command.
’
’ [ PC ]
’ |
’ |
’ --+-----+-------- Ethernet
’ |
’ [VJET] IP Adr:192.168.1.101
’ |
’ +--[VJU7]Address:01
’ RS485BUS|
’ +--[VJU7]Address:02
’ | :
’
’
’The following procedure is described using the real numbers and real
character strings for explanation. Check error processing and retry
processing in normal conditions are omitted. The program does not
operate only by this procedure. Please make it the refernce at the time of
actual application creation.
’
’/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
’
’--------------------------------------------------------------------------------------------------
’Variable declaration
’
Option Explicit
Dim strSendData As String
’Sending data
Dim strReceive As String
’Received data
Dim binChrs(11) As Byte
’Store binary data
Dim i As Integer
’Variable declaration
’
’--------------------------------------------------------------------------------------------------
Private Sub cmdSend()
’
’Procedure to connect to VJET by TCP/IP, create command and send the
command.
’
’
’Variable declaration
’
Dim sChr2 As String
Dim varChrs As String
’
’Set properties of Winsock control
’
Winsock1.Protocol = sckTCPProtocol
’Set protocol used for TCP
connection
Winsock1.RemoteHost = “192.168.1.101”
’Set IP address of VJET
Winsock1.RemotePort = 502
’Set a port of VJET (502: default)
’
’Request TCP connection of VJET
’
Winsock1.Connect
’Request TCP connection
Do Until Winsock1.State = sckConnected
’Wait for the
comletion of connection
i = DoEvents()
Loop
’
’Create sending data
’
’
’Create command to read input value data and D register addresses from
“D0002” to “D0005” of VJU7 (Address: 01) connected to RS-485 of VJET.
D0002: Input value (engineering unit); D0003: Number of digits below
decimal popint; D0004: Input value (ratio of input to span, %); D0005: Unit
of input value (engineering unit)
’
’
strSendData = “123400000006010300010004”
’Sending command
character string
’
’ Explanation of command character string
’ 1234 0000 0006 01 03 0001 0004
’ | | | | | | +---
Number of D register (4)
’ | | | | | +---
D register start number (D0002)
’ | | | | +---Funcion code (03: Read data from
’ | | | | multiple registers)
’ | | | +---RS-485 connection device address (Address: 1)
’ | | +---The number of sending data bytes after
’ | | “RS-485 connection device address” (6 bytes)
’ | +------Protocol ID (0000 fixed)
’ +------
Transaaction ID (1234: Arbitrary value of 2 bytes)
’
varChrs = StrConv(strSendData, vbFromUnicode)
’To change
sending command character string into binary data
For i = 1 To 12
’Change every two character strings into Unicode and arrange
them to store in binDhrs
sChr2 = MidB(varChrs, 2 * i - 1, 2)
binChrs(i - 1) = CByte(“&H” & StrConv(sChr2, vbUnicode))
Next i
’
’Send sending command binChrs to VJET
’
Winsock1.SendData binChrs
’
End Sub
’--------------------------------------------------------------------------------------------------
Private Sub Winsock1_DataArrival(ByVal bytesTotal As Long)
’
’Receive data from VJET DataArrival of Winsock and cut connection with
VJET.
’
’
’Variable declaration
’
Dim strData() As Byte
Dim strHex1 As String
Dim strReceive As String
Dim varReceive As String
’
’Receive data from VJET
’
Winsock1.GetData strData
’Get received data of Winsock1 control
into strData
’
’Change received binary data strings to character strings.
’
’
For i = 0 To bytesTotal - 1
varReceive = varReceive & ChrB(strData(i))
Next i
’
For i = 1 To LenB(varReceive)
strHex1 = Right(“0” & Hex(AscB(MidB(varReceive, i, 1))), 2)
strReceive = strReceive & strHex1
Next i
’Received data character string are stored in strReceive
’
’Example of received characters strReceive from VJU7 (Instrument range: 0
to 1000
°
C; Input value: 680.2
°
C)
’
’ 1234 0000 000B 01 03 08 1A92 0001 02A8 0003
’ | | | | | | | | | |
’ | | | | | | | | | +--D0005 register
| | | | | | | | | data (Unit: 3=
°
C)
’ | | | | | | | | +--D004 register data
(Input value %: 02A8h=680:68.0)
’ | | | | | | | +--D0003 register data
(Number of digits below decimal pont: 1)
’ | | | | | | +--D0002 register data
(Input value: 1A92h=6802: 680.2)
’ | | | | | +--Data amount bytes (8 bytes)
’ | | | | +--Function code (03: Read data from mutiple registers) |
’ | | | +--RS-485 connection device address (Address 1) | |
’ | | +--
The number of send data bytes after “RS-485 connection device address” (000Bh=11 bytes)
’ | +--Protocol ID (0000 fixed)
’ +--Transaction ID (The value when sending 1234 command)
’
’Cut TCP connection
’
Winsock1.Close
’
End Sub
’--------------------------------------------------------------------------------------------------
’
’/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////