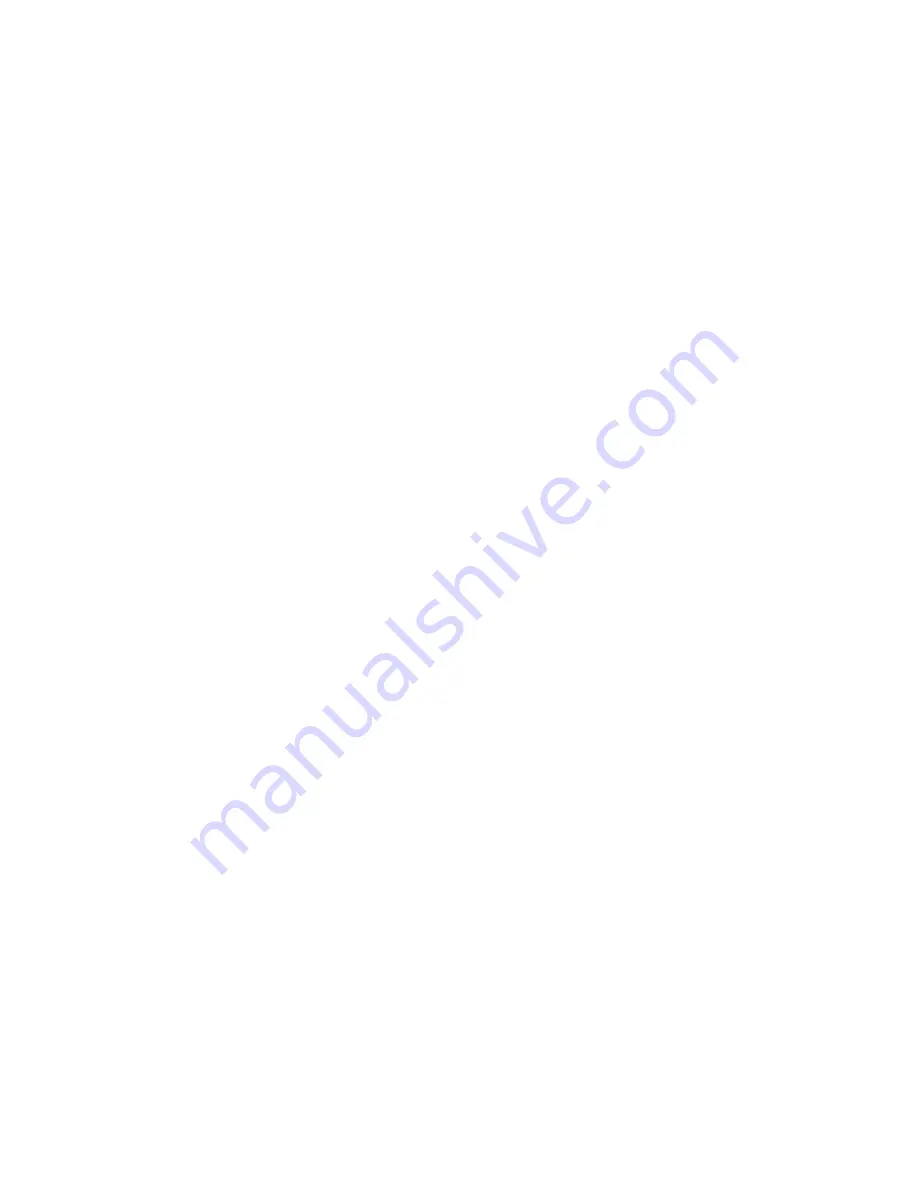
Standard V6 Series Communication Protocol
6
118109-001 REV B
The following is sample code, written in Visual Basic, for the generation of
checksums:
Public Function ProcessOutputString(outputString As String) As String
Dim i As Integer
Dim CSb1 As Integer
Dim CSb2 As Integer
Dim CSb3 As Integer
Dim CSb$
Dim X
X = 0
For i = 1 To (Len(outputString)) 'Starting with the CMD character
X = X + Asc(Mid(outputString, i, 1)) 'adds ascii values together
Next i
CSb1 = 256 - X
'Twos Complement
CSb2 = 63 And (CSb1)
CSb3 = 64 Or (CSb2) 'OR 0x40
CSb$ = Chr(Val("&H" & (Hex(CSb3))))
ProcessOutputString = Chr(2) & outputString & CSb$ & Chr(3)
End Function
Here is an example of an actual Checksum calculation for command 10
(Program kV setpoint)
The original message with a placeholder for the checksum is
<STX>10,4095,<CSUM><ETX>
First, you add up all the characters starting with the ‘1’ in the command
number, to the comma before the checksum with their ASCII values (in
hexadecimal):
0x31 + 0x30 + 0x2C + 0x34 + 0x30 + 0x39 + 0x35 + 0x2C = 0x18B
Next, you then take the two’s complement of that number by negating it,
by subtracting it from 0x100 (decimal 256), and only retain the lowest 7
bits by bitwise ANDing the results with 0x7F. :
NOTE: This combines the steps of getting the twos complement,
truncating the result to 8 bits and clearing the 8
th
bit.