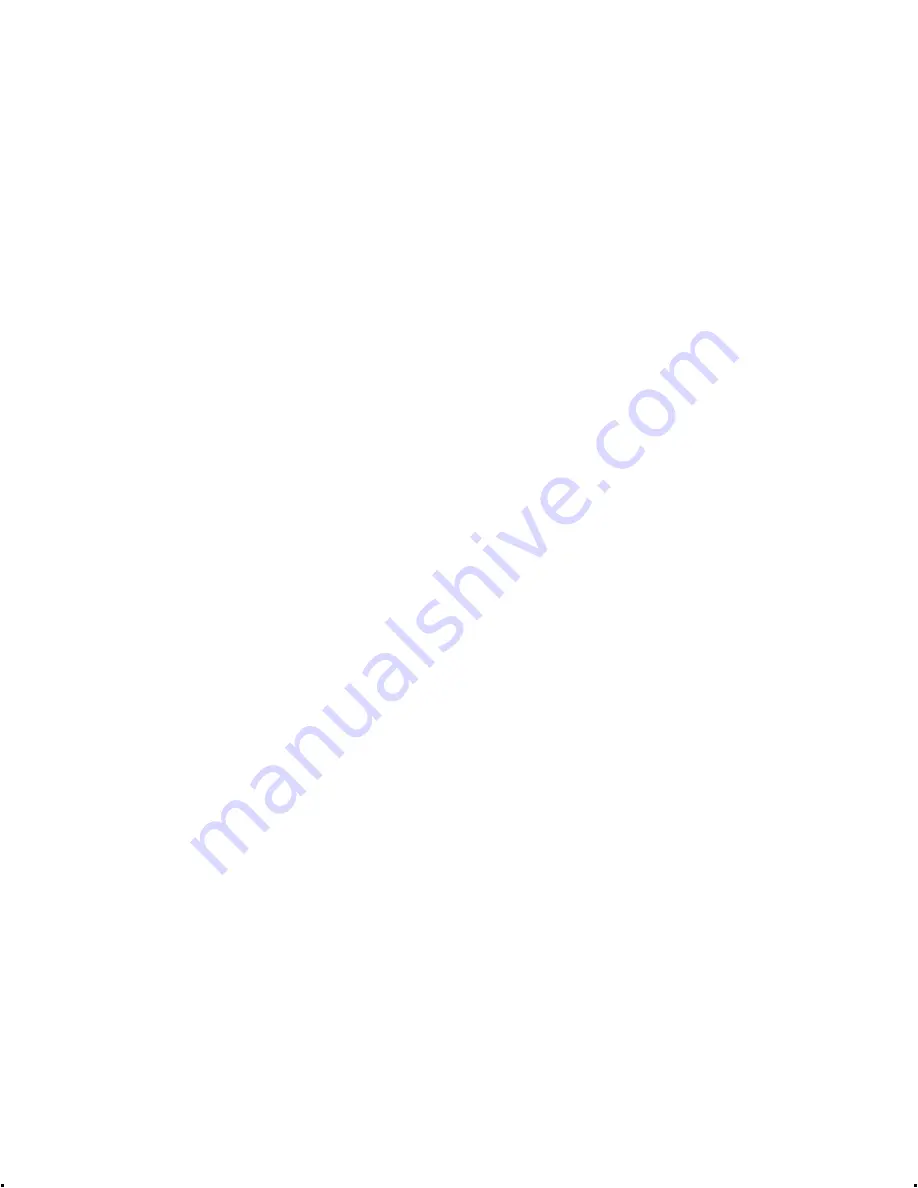
6-5
End-of-Interrupt (EOI) Command
After an interrupt service routine is complete, the appropriate 8259 interrupt controller must be notified.
When using IRQ0-IRQ7, this is done by writing the value 20H to I/O port 20H only; when using IRQ8-IRQ15,
you must write the value 20H to I/O ports 20H and A0H.
What Exactly Happens When an Interrupt Occurs?
Understanding the sequence of events when an interrupt is triggered is necessary to properly write software
interrupt handlers. When an interrupt request line is driven high by a peripheral device (such as the DM6210),
the interrupt controllers check to see if interrupts are enabled for that IRQ, and then checks to see if other
interrupts are active or requested and determine which interrupt has priority. The interrupt controllers then
interrupt the processor. The current code segment (CS), instruction pointer (IP), and flags are pushed on the
stack for storage, and a new CS and IP are loaded from a table that exists in the lowest 1024 bytes of memory.
This table is referred to as the interrupt vector table and each entry is called an interrupt vector. Once the new
CS and IP are loaded from the interrupt vector table, the processor begins executing the code located at CS:IP.
When the interrupt routine is completed, the CS, IP, and flags that were pushed on the stack when the interrupt
occurred are now popped from the stack and execution resumes from the point where it was interrupted.
Using Interrupts in Your Programs
Adding interrupts to your software is not as difficult as it may seem, and what they add in terms of perfor-
mance is often worth the effort. Note, however, that although it is not that hard to use interrupts, the smallest
mistake will often lead to a system hang that requires a reboot. This can be both frustrating and time-consuming.
But, after a few tries, youll get the bugs worked out and enjoy the benefits of properly executed interrupts. In
addition to reading the following paragraphs, study the example programs included on your DM6210 pro-
gram disk for a better understanding of interrupt program development.
Writing an Interrupt Service Routine (ISR)
The first step in adding interrupts to your software is to write the interrupt service routine (ISR). This is the
routine that will automatically be executed each time an interrupt request occurs on the specified IRQ. An ISR is
different than standard routines that you write. First, on entrance, the processor registers should be pushed onto
the stack
BEFORE
you do anything else. Second, just before exiting your ISR, you must write an end-of-interrupt
command to the 8259 controller(s). Since 8259B generates a request on IRQ2 which is handled by 8259A, an EOI
must be sent to both 8259A and 8259B for IRQ8-IRQ15. Finally, when exiting the ISR, in addition to popping all
the registers you pushed on entrance, you must use the IRET instruction and
not
a plain RET. The IRET auto-
matically pops the flags, CS, and IP that were pushed when the interrupt was called.
If you find yourself intimidated by these requirements, take heart. Most Pascal and C compilers allow you to
identify a procedure (function) as an interrupt type and will automatically add these instructions to your ISR, with
one important exception: most compilers
do not
automatically add the end-of-interrupt command to the proce-
dure; you must do this yourself. Other than this and the few exceptions discussed below, you can write your ISR
just like any other routine. It can call other functions and procedures in your program and it can access global
data. If you are writing your first ISR, we recommend that you stick to the basics; just something that will
convince you that it works, such as incrementing a global variable.
NOTE:
If you are writing an ISR using assembly language, you are responsible for pushing and popping
registers and using IRET instead of RET.
There are a few cautions you must consider when writing your ISR. The most important is,
do not use any
DOS functions or routines that call DOS functions from within an ISR
. DOS is
not
reentrant; that is, a DOS
function cannot call itself. In typical programming, this will not happen because of the way DOS is written. But
what about when using interrupts? Then, you could have a situation such as this in your program. If DOS function
X is being executed when an interrupt occurs and the interrupt routine makes a call to DOS function X, then
function X is essentially being called while it is already active. Such a reentrancy attempt spells disaster because
DOS functions are not written to support it. This is a complex concept and you do not need to understand it. Just
make sure that you do not call any DOS functions from within your ISR. The one wrinkle is that, unfortunately, it
Summary of Contents for DM6210
Page 2: ......
Page 9: ...i 1 INTRODUCTION...
Page 10: ...i 2...
Page 14: ...1 2...
Page 22: ...1 10...
Page 24: ...2 2...
Page 28: ...2 6...
Page 30: ...3 2...
Page 34: ...4 2...
Page 44: ...5 2...
Page 48: ...5 6...
Page 50: ...6 2...
Page 56: ...6 8...
Page 58: ...7 2...
Page 61: ...8 1 CHAPTER 8 DIGITAL I O This chapter explains the digital I O circuitry on the DM6210...
Page 62: ...8 2...
Page 66: ...9 2...
Page 68: ...9 4...
Page 70: ...10 2...
Page 74: ...10 6...
Page 75: ...A 1 APPENDIX A DM6210 SPECIFICATIONS...
Page 76: ...A 2...
Page 78: ...A 4...
Page 79: ...B 1 APPENDIX B CN3 CONNECTOR PIN ASSIGNMENTS...
Page 80: ...B 2...
Page 82: ...B 4...
Page 83: ...APPENDIX C COMPONENT DATA SHEETS C 1...
Page 84: ......
Page 85: ...Intel 82C54 Programmable Interval Timer Data Sheet Reprint...
Page 86: ......
Page 88: ...D 2...
Page 91: ...DM6210 User Settings Base I O Address hex decimal IRQ Channel...