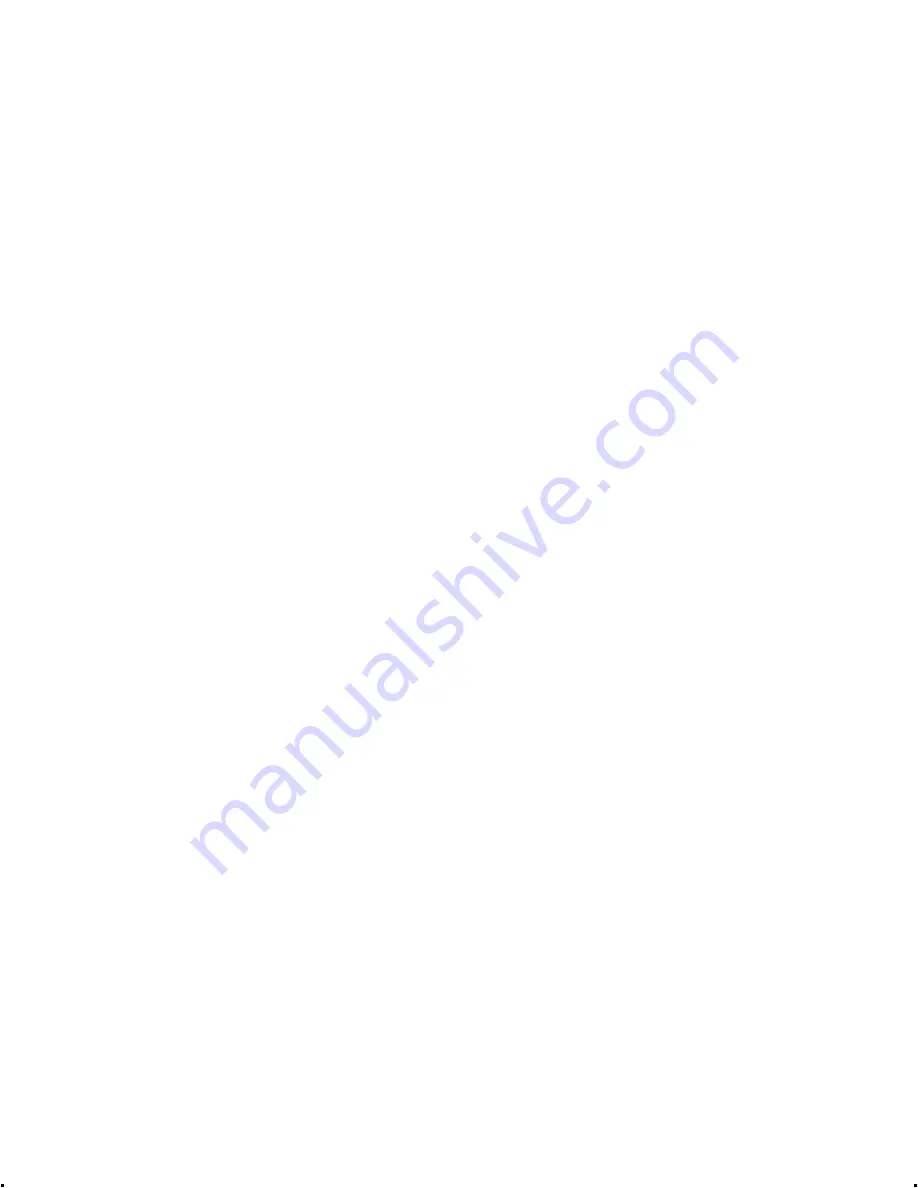
6-6
is not obvious which library routines included with your compiler use DOS functions. A rule of thumb is that
routines which write to the screen, or check the status of or read the keyboard, and any disk I/O routines use DOS
and should be avoided in your ISR.
The same problem of reentrancy exists for many floating point emulators as well, meaning you may have to
avoid floating point (real) math in your ISR.
Note that the problem of reentrancy exists, no matter what programming language you are using. Even if you
are writing your ISR in assembly language, DOS and many floating point emulators are not reentrant. Of course,
there are ways around this problem, such as those which involve checking to see if any DOS functions are
currently active when your ISR is called, but such solutions are well beyond the scope of this discussion.
The second major concern when writing your ISR is to make it as short as possible in terms of execution
time. Spending long periods of time in your ISR may mean that other important interrupts are being ignored.
Also, if you spend too long in your ISR, it may be called again before you have completed handling the first run.
This often leads to a hang that requires a reboot.
Your ISR should have this structure:
Push any processor registers used in your ISR. Most C and Pascal interrupt routines automatically do this
for you.
Put the body of your routine here.
Issue the EOI command to the 8259 interrupt controller by writing 20H to port 20H and port A0H (if you
are using IRQ8-IRQ15).
Pop all registers pushed on entrance. Most C and Pascal interrupt routines automatically do this for you.
The following C and Pascal examples show what the shell of your ISR should be like:
In C:
void interrupt ISR(void)
{
/* Your code goes here. Do not use any DOS functions! */
outportb(0x20, 0x20);
/* Send EOI command to 8259A (for all IRQs)*/
outportb(0x20, 0xA0);
/* Send EOI command to 8259B (if using IRQ8-
15) */
}
In Pascal:
Procedure ISR; Interrupt;
begin
{ Your code goes here. Do not use any DOS functions! }
Port[$20] := $20;
{ Send EOI command to 8259A (for all IRQs) }
Port[$A0] := $20;
{ Send EOI command to 8259B (if using IRQ8-
15) }
end;
Saving the Startup Interrupt Mask Register (IMR) and Interrupt Vector
The next step after writing the ISR is to save the startup state of the interrupt mask register and the interrupt
vector that you will be using. The IMR for IRQ0-IRQ7 is located at I/O port 21H; the IMR for IRQ8-IRQ15 is
located at I/O port A1H. The interrupt vector you will be using is located in the interrupt vector table which is
simply an array of 256 four-byte pointers and is located in the first 1024 bytes of memory (Segment = 0, Offset =
0). You can read this value directly, but it is a better practice to use DOS function 35H (get interrupt vector). Most
C and Pascal compilers provide a library routine for reading the value of a vector. The vectors for IRQ0-IRQ7 are
vectors 8 through 15, where IRQ0 uses vector 8, IRQ1 uses vector 9, and so on. The vectors for IRQ8-IRQ15 are
vectors 70H through 77H, where IRQ8 uses vector 70H, IRQ9 uses vector 71H, and so on. Thus, if the
DM6210 will be using IRQ15, you should save the value of interrupt vector 77H.
Summary of Contents for DM6210
Page 2: ......
Page 9: ...i 1 INTRODUCTION...
Page 10: ...i 2...
Page 14: ...1 2...
Page 22: ...1 10...
Page 24: ...2 2...
Page 28: ...2 6...
Page 30: ...3 2...
Page 34: ...4 2...
Page 44: ...5 2...
Page 48: ...5 6...
Page 50: ...6 2...
Page 56: ...6 8...
Page 58: ...7 2...
Page 61: ...8 1 CHAPTER 8 DIGITAL I O This chapter explains the digital I O circuitry on the DM6210...
Page 62: ...8 2...
Page 66: ...9 2...
Page 68: ...9 4...
Page 70: ...10 2...
Page 74: ...10 6...
Page 75: ...A 1 APPENDIX A DM6210 SPECIFICATIONS...
Page 76: ...A 2...
Page 78: ...A 4...
Page 79: ...B 1 APPENDIX B CN3 CONNECTOR PIN ASSIGNMENTS...
Page 80: ...B 2...
Page 82: ...B 4...
Page 83: ...APPENDIX C COMPONENT DATA SHEETS C 1...
Page 84: ......
Page 85: ...Intel 82C54 Programmable Interval Timer Data Sheet Reprint...
Page 86: ......
Page 88: ...D 2...
Page 91: ...DM6210 User Settings Base I O Address hex decimal IRQ Channel...