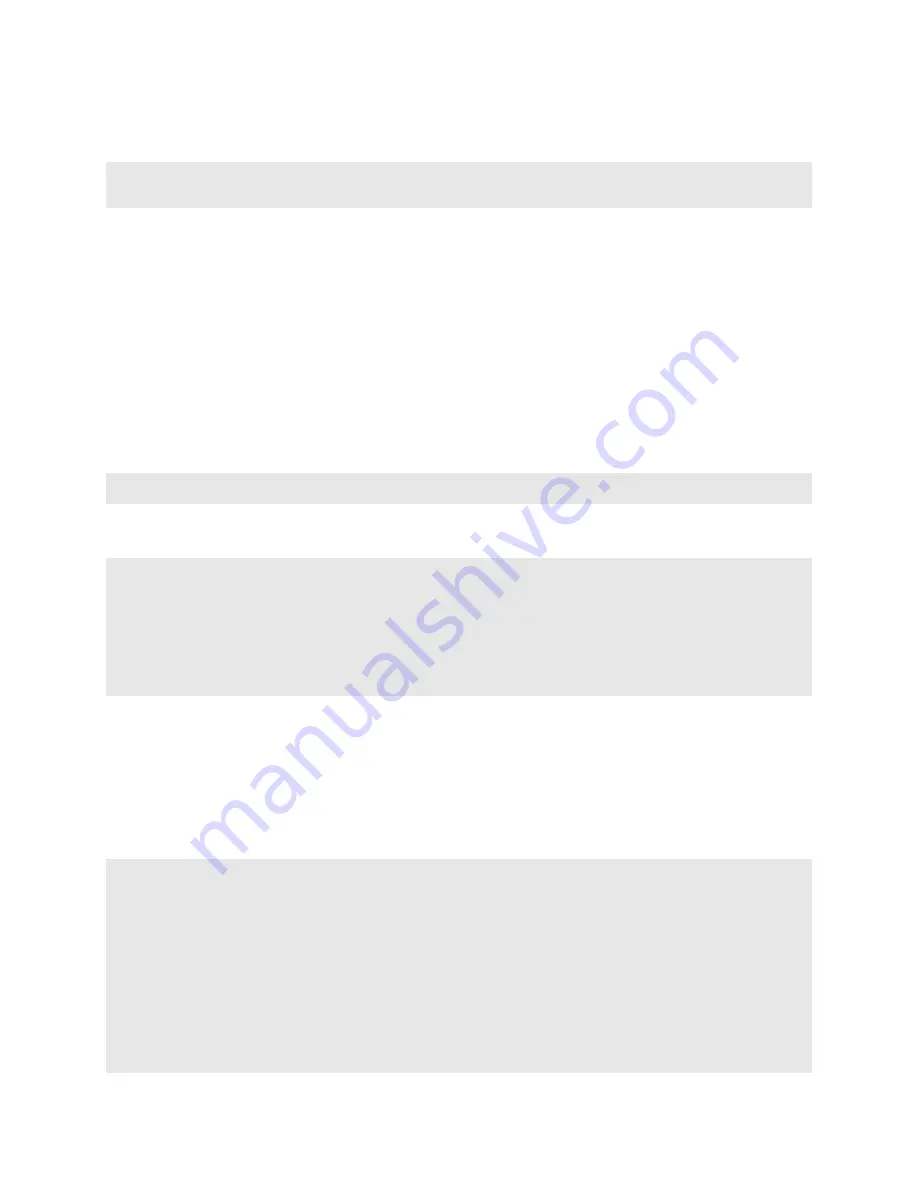
© Bueno Systems, Inc. • TSL1401-DB (2009.10.01)
Page 7 of 52
You can use
DEBUG
to display the acquired pixels, as in the following program fragment. (A somewhat
fancier version is given in the complete program later in this section.)
FOR i = 0 TO 7
DEBUG BIN16 pdata(i) REV 16
NEXT
The variable
i
can be declared as a
NIB
ble. The reason for the
REV
is because the data were read in
LSB first, but
DEBUG
’s
BIN
formatter displays data
MSB
-first. So we need to reverse the order of the
bits to get an accurate picture of the pixel order. One might well ask why we didn’t just read the data in
MSB first to begin with. After all,
SHIFTIN
, can do that just as easily. The answer lies in the image
analysis routines to follow.
Image Analysis
Analyzing a linescan image to extract useful information from it involves two major operations: pixel
counting, and pixel and edge location. The PBASIC subroutines that perform these operations treat the
original array of eight words as an array of 128 bits, each bit corresponding to a single pixel. Bit 0 is the
first pixel read; bit 127, the last. (This mapping is why the pixels needed to be read in LSB first.) Here’s
how the word and bit arrays are declared:
pdata VAR Word(8)
pixels VAR pdata.BIT0
Counting light or dark pixels within a given range is simple. Here’s the code that does it:
CountPix:
cnt = 0 'Initialize count.
IF (lptr <= rptr AND rptr <= 127) THEN 'Valid range?
FOR i = lptr TO rptr ' Yes: Loop over desired range.
IF (pixels(i) = which) THEN cnt = cnt + 1 ' Add to count when pixel matches.
NEXT
ENDIF
RETURN
cnt
can be declared as a byte, since it will never exceed 128.
lptr
and
rptr
are also bytes that can range
from 0 to 127, inclusive. They indicate the range over which the counting occurs.
which
is a bit variable
that indicates whether to count dark pixels (
0
) or light pixels (
1
). Counting pixels is handy for computing
an object’s area – either in one scan, or cumulatively over multiple scans for two-dimensional objects
passing under the camera on a conveyor.
Locating the first occurrence of a dark or light pixel within a given range isn’t much harder:
FindPix:
IF (found = 1 AND lptr <= rptr AND rptr <= 127) THEN
'Still looking & within bounds?
IF (dir = FWD) THEN ' Yes: Search left-to-right?
FOR lptr = lptr TO rptr ' Yes: Loop forward.
IF (pixels(lptr) = which) THEN RETURN ' Return on match.
NEXT
ELSE
FOR rptr = rptr TO lptr ' No: Loop backward.
IF (pixels(rptr) = which) THEN RETURN ' Return on match.
NEXT
ENDIF
ENDIF