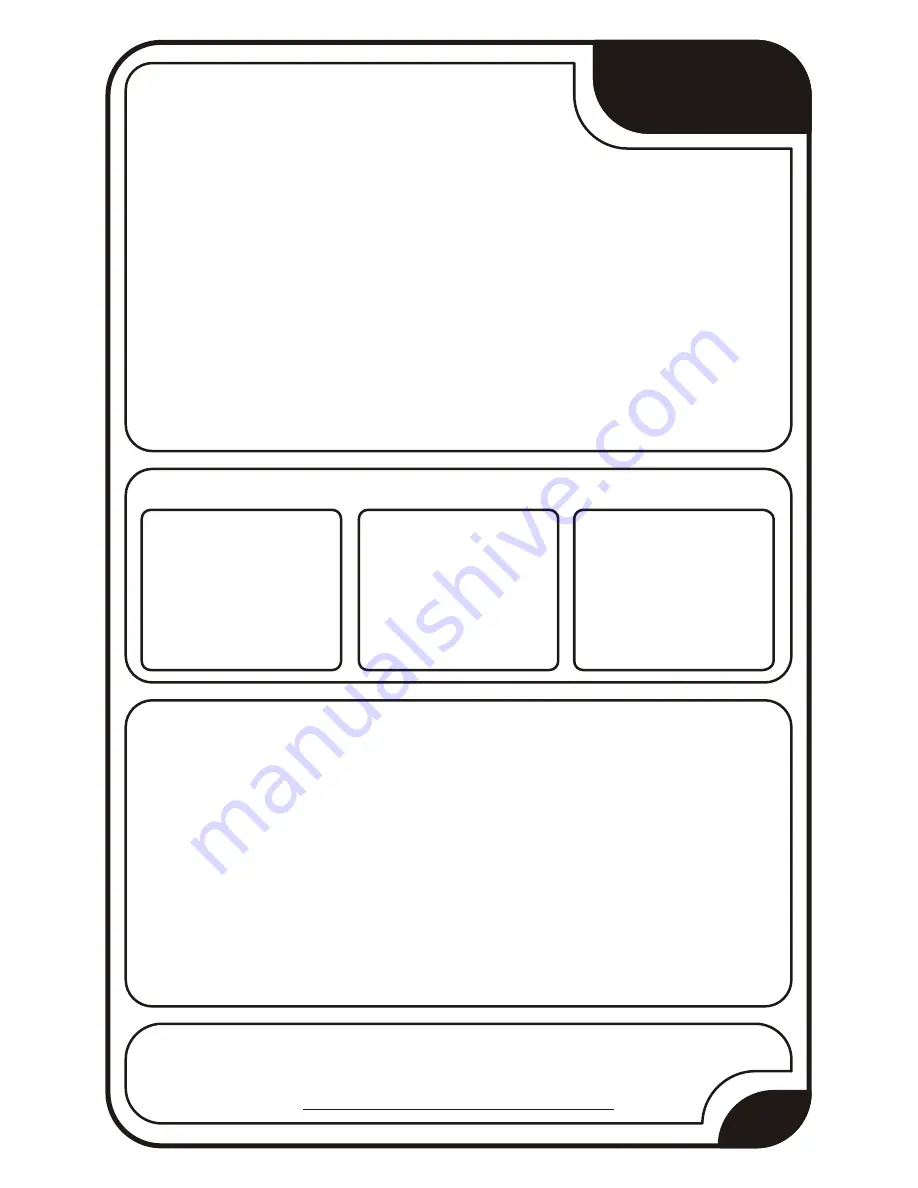
Still No Luck?
if you sourced your own motor,
double check that it will work
with 5 volts and that it does not
draw too much power.
13
CIRC-03
Code
(no need to type everything in just)
Not Working?
(3 things to try)
More, More, More:
More details, where to buy more parts, where to ask more questions.
http://tinyurl.com/d4wht7
Making it Better
Motor Not Spinning?
if you sourced your own
transistor, double check with
the data sheet that the pinout
is compatible with a P2N2222A
(many are reversed)
Controlling Speed:
In the
loop()
section change it to this
// motorOnThenOff();
We played with the Arduino's ability to control the
motorOnThenOffWithSpeed();
brightness of an LED earlier now we will use the same
//motorAcceleration();
Then upload the programme. You can change the speeds by
feature to control the speed of our motor. The Arduino
changing the variables onSpeed and offSpeed
does this using something called Pulse Width
Modulation (PWM). This relies on the Arduino's ability to
Accelerating and decelerating:
operate really really fast. Rather than directly controlling
Why stop at two speeds, why not accelerate and decelerate
the voltage coming from the pin the Arduino will switch
the motor. To do this simply change the loop() code to read
// motorOnThenOff();
the pin on and off very quickly. In the computer world
// motorOnThenOffWithSpeed();
this is going from 0 to 5 volts many times a second, but
motorAcceleration();
in the human world we see it as a voltage. For example
Then upload the program and watch as your motor slowly
if the Arduino is PWM'ing at 50% we see the light
accelerates up to full speed then slows down again. If you
dimmed 50% because our eyes are not quick enough to
would like to change the speed of acceleration change the
see it flashing on and off. The same feature works with
variable delayTime (larger means a longer acceleration time)
transistors. Don't believe me? Try it out.
Still Not Working?
Sometimes the Arduino board
will disconnect from the
computer. Try un-plugging and
then re-plugging it into your
USB port.
int motorPin = 9; //pin the motor is connected to
void setup() //runs once
void motorOnThenOffWithSpeed(){
{
int onSpeed = 200;// a number between
pinMode(motorPin, OUTPUT);
//0 (stopped) and 255 (full speed)
}
int onTime = 2500;
int offSpeed = 50;// a number between
void loop() // run over and over again
//0 (stopped) and 255 (full speed)
{
int offTime = 1000;
motorOnThenOff();
analogWrite(motorPin, onSpeed);
//motorOnThenOffWithSpeed();
// turns the motor On
//motorAcceleration();
delay(onTime); // waits for onTime milliseconds
}
analogWrite(motorPin, offSpeed);
// turns the motor Off
/*
delay(offTime); // waits for offTime milliseconds
* motorOnThenOff() - turns motor on then off
}
* (notice this code is identical to the code we
void motorAcceleration(){
used for
int delayTime = 50; //time between each speed step
* the blinking LED)
for(int i = 0; i < 256; i++){
*/
//goes through each speed from 0 to 255
void motorOnThenOff(){
analogWrite(motorPin, i); //sets the new speed
int onTime = 2500; //on time
delay(delayTime);//
waits for delayTime milliseconds
int offTime = 1000; //off time
}
digitalWrite(motorPin, HIGH);
for(int i = 255; i >= 0; i--){
// turns the motor On
//goes through each speed from 255 to 0
delay(onTime); // waits for onTime milliseconds
analogWrite(motorPin, i); //sets the new speed
digitalWrite(motorPin, LOW);
delay(delayTime);//
waits for delayTime milliseconds
// turns the motor Off
}
delay(offTime);
// waits for offTime milliseconds
}
}
Download the Code from ( http://tinyurl.com/dagyrb )
(then simply copy the text and paste it into an empty Arduino Sketch)
12
CIRC-03
What We’re Doing:
.:Spin Motor Spin:.
.:Transistor & Motor:.
The Arduino's pins are great for directly controlling small electric
items like LEDs. However, when dealing with larger items (like a
toy motor or washing machine), an external transistor is required.
A transistor is incredibly useful. It switches a lot of current using a
much smaller current. A transistor has 3 pins. For a negative type
(NPN) transistor you connect your load to collector and the emitter to ground. Then when a small
current flows from base to the emitter a current will flow through the transistor and your motor will
spin (this happens when we set our Arduino pin HIGH). There are literally thousands of different
types of transistors, allowing every situation to be perfectly matched. We have chosen a P2N2222AG
a rather common general purpose transistor. The important factors in our case are that its maximum
voltage (40 v) and its maximum current (600 milliamp) are both high enough for our toy motor (full
details can be found on its datasheet http://tinyurl.com/o2cm93 )
note: the transistor we use has a in order Base Collector Emitter pinout (differing from some other popular transistors)
(The 1N4001 diode is acting as a flyback diode for details on why its there visit: http://tinyurl.com/b559mx)
The Circuit:
Wire
Transistor
P2N2222AG (TO92)
x1
2.2k Ohm Resistor
Red-Red-Red
x1
2 Pin Header
x4
CIRC-03
Breadboard sheet
x1
Parts:
Toy Motor
x1
Schematic:
Arduino
pin 9
resistor
(2.2kohm)
gnd
(ground) (-)
Collector
Emitter
Base
Motor
+5 volts
Transistor
P2N2222AG
the transistor will have
P2N2222AG printed on it
(some variations will have
the pin assignment reversed)
Diode
(1N4001)
x1
Diode
.:download:.
breadboard layout sheet
http://tinyurl.com/d6jv63
.:view:.
assembling video
http://tinyurl.com/djapjg
The Internet
.:NOTE: if your arduino is resetting you need to install the optional capacitor:.