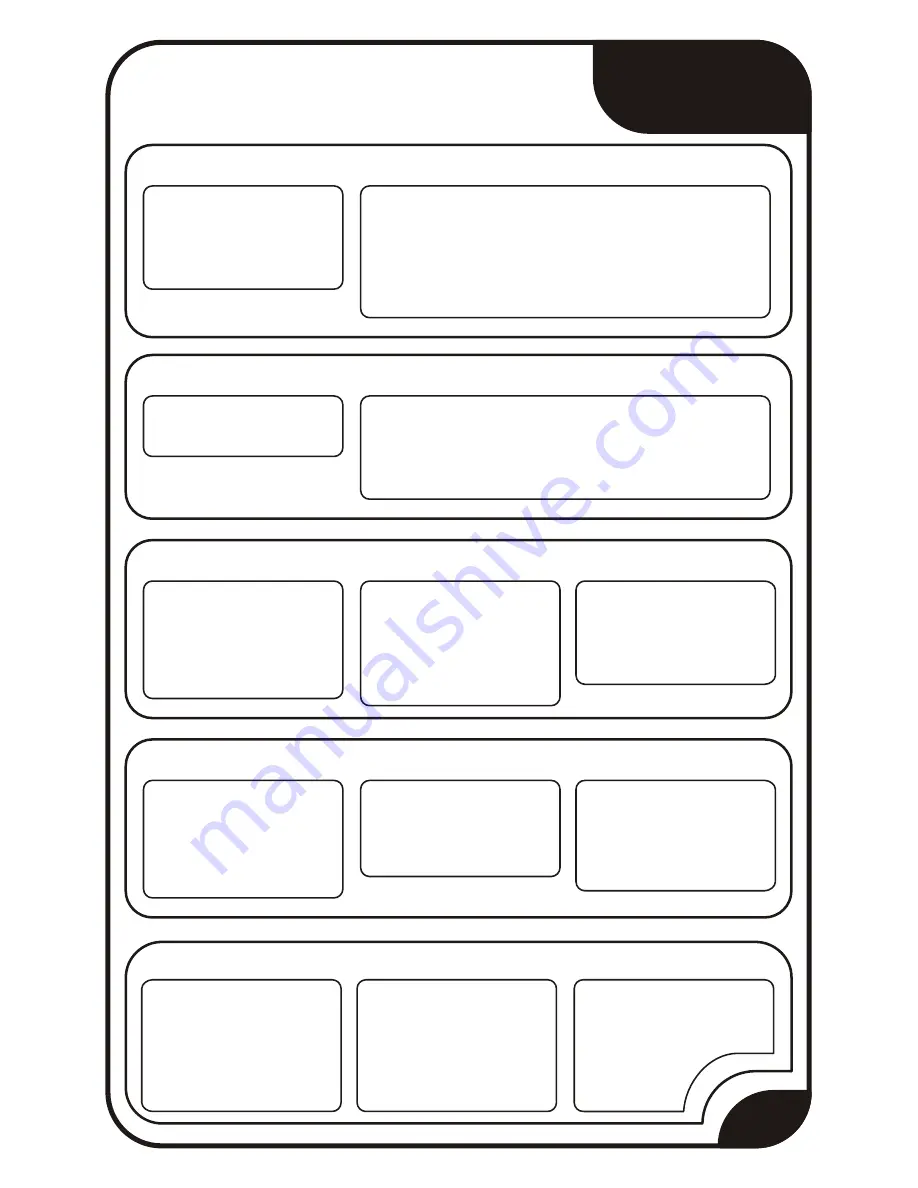
//
(single line comment)
It is often useful to write notes
to yourself as you go along
about what each line of code
does. To do this type two back
slashes and everything until the
end of the line will be ignored by
your program.
{ }
(curly brackets)
Used to define when a block of
code starts and ends (used in
functions as well as loops)
05
04
03 PROG
programming
primer
.: A Small Programming Primer:.
03 PROG
programming
primer
Arduino Programming in Brief
The Arduino is programmed in the C language. This is a quick little primer targeted at people
who have a little bit of programing experience and just need a briefing on the ideosyncrocies of
C and the Arduino IDE. If you find the concepts a bit daunting, don't worry, you can start going
through the circuits and pick up most of it along the way. For a more in depth intro the
Arduino.cc website is a great resource
(the foundations page http://tinyurl.com/954pun)
Structure
void setup(){ }
All the code between the two
curly brackets will be run once
when your Arduino program
first runs.
Each Arduino program
(often called a sketch) has
two required functions
(also called routines).
Variables
void loop(){ }
This function is run after setup
has finished. After it has run
once it will be run again,
and
aga
in, until power is removed.
Syntax
;
(semicolon)
Each line of code must be
ended with a semicolon (a
missing semicolon is often the
reason for a programme
refusing to compile)
One of the slightly
frustrating elements of C is
its formating requirements
(this also makes it very
powerful). If you remember
the following you should be
alright.
/* */
(multi line comment)
If you have a lot to say you can
span several lines as a
comment. Everything between
these two symbols will be
ignored in your program.
A program is nothing more
than instructions to move
numbers around in an
intelligent way. Variables are
used to do the moving
long
(long)
Used when an integer is not
large enough. Takes 4 bytes
(32 bits) of RAM and has a
range between -2,147,483,648
and 2,147,483,648.
int
(integer)
The main workhorse, stores a
number in 2 bytes (16 bits).
Has no decimal places and will
store a value between -32,768
and 32,768.
boolean
(boolean)
A simple True or False variable.
Useful because it only uses one
bit of RAM.
char
(character)
Stores one character using the
ASCII code (ie 'A' = 65). Uses
one byte (8 bits) of RAM. The
Arduino handles strings as an
array of char’s
float
(float)
Used for floating point math
(decimals). Takes 4 bytes (32
bits) of RAM and has a range
between -3.438 and
3.438.
Maths Operators
=
(assignment) makes something equal to something else (eg. x =
10 * 2 (x now equals 20))
%
(modulo) gives the remainder when one number is divided by
another (ex. 12 % 10 (gives 2))
+
(addition)
-
(subtraction)
*
(multiplication)
/
(division)
Operators used for
manipulating numbers.
(they work like simple
maths)
Comparison Operators
==
(equal to)
(eg. 12 == 10 is FALSE or 12 == 12 is TRUE)
!=
(not equal to)
(eg. 12 != 10 is TRUE or 12 != 12 is FALSE)
<
(less
than)
(eg. 12 < 10 is FALSE or 12 < 12 is FALSE or 12 < 14 is TRUE)
>
(greater
than)
(eg. 12 > 10 is TRUE or 12 > 12 is FALSE or 12 > 14 is
FALSE)
Operators used for logical
comparison
Control Structure
if(condition){ }
else if( condition ){ }
else { }
This will execute the code between
the curly brackets if the condition is
true, and if not it will test the
else
if
condition if that is also false the
else
code will execute.
Programs are reliant on
controlling what runs
next, here are the basic
control elements (there
are many more online)
for(int i = 0; i <
#repeats; i++){ }
Used when you would like to
repeat a chunk of code a number
of times (can count up i++ or
down i-- or use any variable)
Digital
digitalWrite(pin, value);
Once a pin is set as an
OUTPUT
,
it can be set either
HIGH
(pulled
to +5 volts) or
LOW
(pulled to
ground).
pinMode(pin, mode);
Used to set a pins mode,
pin
is
the pin number you would like
to address (0-19 (analog 0-5
are 14-19). the mode can either
be
INPUT
or
OUTPUT
.
int digitalRead(pin);
Once a pin is set as an INPUT
you can use this to return
whether it is HIGH (pulled to
+5 volts) or LOW (pulled to
ground).
Analog
int analogWrite(pin,
value);
Some of the Arduino's pins support
pulse width modulation (3, 5, 6, 9, 10,
11). This turns the pin on and off very
quickly making it act like an analog
output. The value is any number
between 0 (0% duty cycle ~0v) and 255
(100% duty cycle ~5 volts).
The Arduino is a digital
machine but it has the ability to
operate in the analog realm
(through tricks). Here's how to
deal with things that aren't
digital.
int analogRead(pin);
When the analog input pins are set
to input you can read their voltage.
A value between 0 (for 0
volts) and 1024 (for 5
volts) will be
returned
.:For a full programming reference visit:.
http://tinyurl.com/882oxm