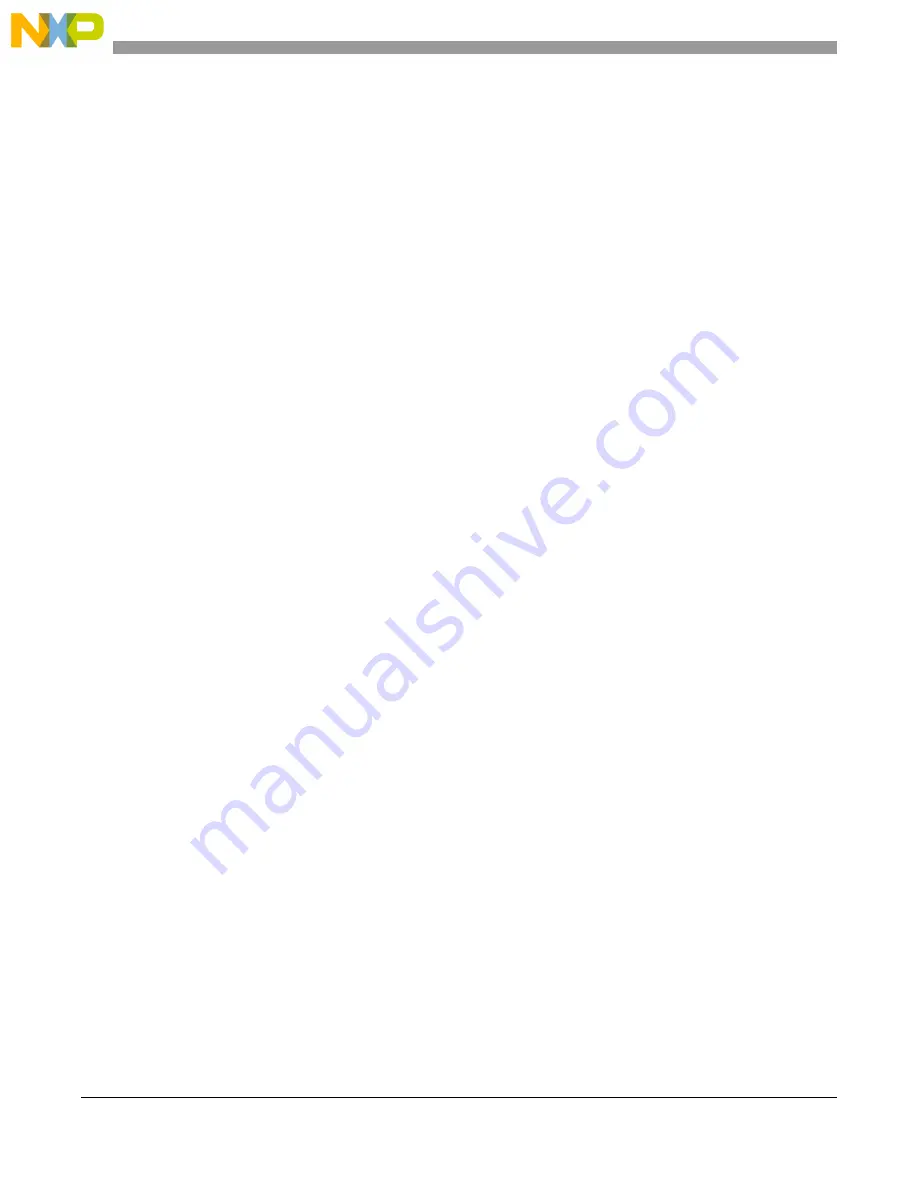
Interfacing an LCD to the MC9S08LC60, Rev. 0
Example Application Using the DEMO9S08LC60
Freescale Semiconductor
22
4.2.3.2.2
Alphanumerics
The example application provides the output_strg_lcd(tU08 *string, tU08 length) function to display alpha
numerics to the LCD display. The complete source code for output_strg_lcd() can be found in lcddrv.c.
The output_strg_lcd() function writes the contents of the string argument to the LCD display. It starts by
writing the rightmost character of the string argument to the rightmost alphanumeric position on the LCD
glass (i.e., the ninth position; see
). The output_strg_lcd() function loops through the string
argument variable characters and indexes to the next LCD glass character position to complete the display.
To display the alphanumerics of the string argument, the software must determine the correct segments of
the 13-segment display to power on while leaving the others off. The software uses a lookup table to
determine the correct segments. The excerpt of the output_strg_lcd() source code shown below illustrates
the usage of the lookup table and the manipulation of the proper LCDRAM registers to display the correct
alphanumeric. When a new value is to be written to the LCDRAM registers, it must be ORed with the
previous value. This is necessary because there are other segments in the LCDRAM register that are not
part of the 13-segment display and their values must be preserved (see
).
c = ((string[i]-0x20)*2); // convert ASCII to table index (in bytes)
if (c < 128) {
*LCD_pos[LCD_position*2-2] = *LCD_pos[LCD_position*2-2] | (tU08)ascii[c];
*LCD_pos[LCD_position*2-1] = *LCD_pos[LCD_position*2-1] |(tU08)ascii[c+1];
}
The look-up table is contained in the ascii[] array, which is shown below. This array determines what
values need to be written to the MCU LCD RAM registers for a given alphanumeric value. The values of
the look-up table were determined using the procedure discussed in
. An Excel worksheet is
provided in AN3280SW1.zip that helps determine the values for the ascii[] array.
const tU08 ascii[] = {
/* */ 0x00,0x00,
/*!*/ 0x00,0x00,
/*"*/ 0x00,0x00,
/*#*/ 0x00,0x00,
/*$*/ 0x00,0x00,
/*%*/ 0x00,0x00,
/*&*/ 0x00,0x00,
/*'*/ 0x0,0x00,
/*(*/ 0x00,0x00,
/*)*/ 0x00,0x00,
/***/ 0xe9,0x3,
/*+*/ 0xe0,0x0,
/*,*/ 0x00,0x00,
/*-*/ 0x40,0x0,
/*.*/ 0x00,0x00,
/* */ 0x00,0x00,
/*0*/ 0x16,0x34,
/*1*/ 0xA0,0x0,
/*2*/ 0x54,0x14,
/*3*/ 0x50,0x34,
/*4*/ 0x42,0x30,
/*5*/ 0x52,0x24,
/*6*/ 0x56,0x24,