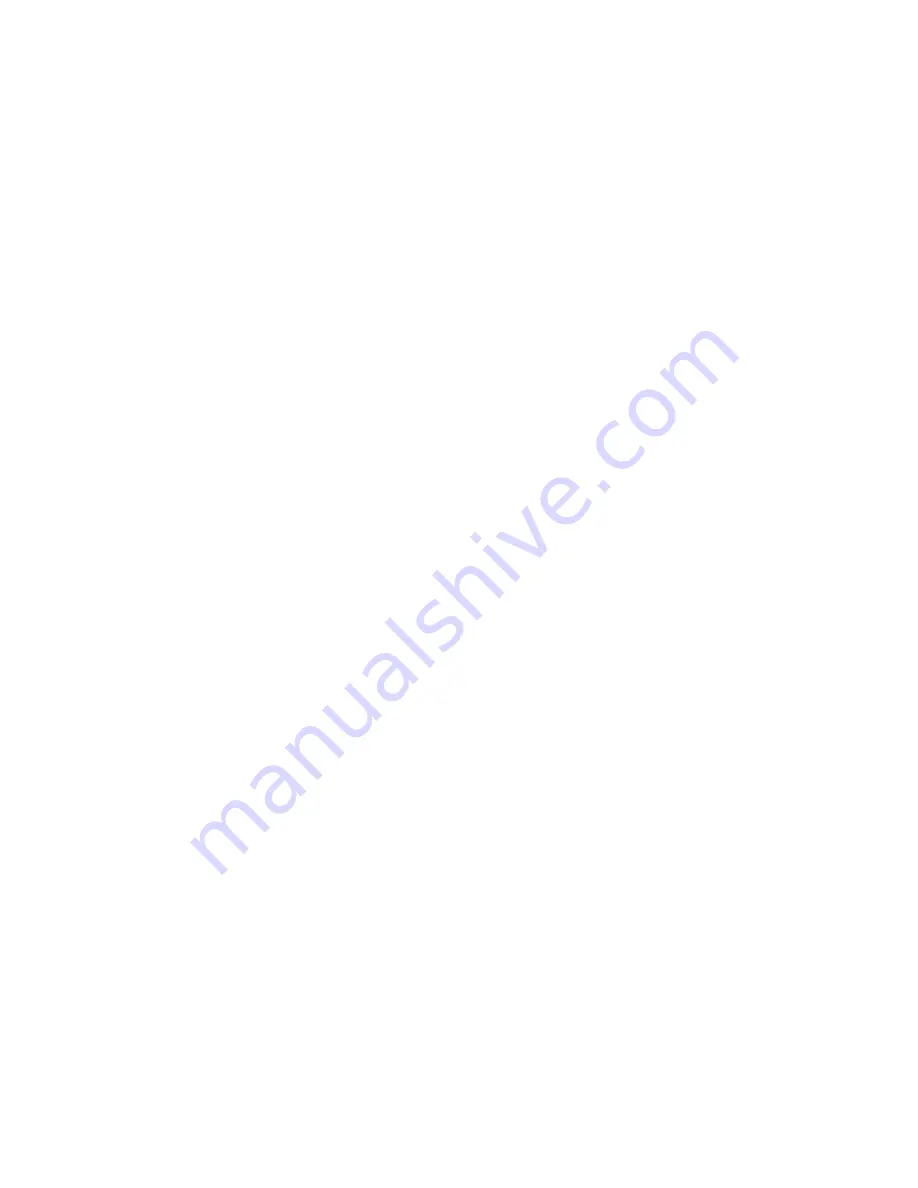
Developing Your Application
Chapter 3
NI-488.2M UM for Windows NT
3-18
© National Instruments Corp.
/* Global variable for the handle to the loaded gpib-32.dll. */
HINSTANCE Gpib32Lib = NULL;
/* Pointers to NI-488.2 global status variables */
int *Pibsta;
int *Piberr;
long *Pibcntl;
#ifdef __cplusplus
}
#endif
In addition to pointers to the status variables and a handle to the loaded
gpib-32.dll
,
you must define the direct entry prototypes for the functions you use in your application.
The prototypes for each function that
gpib-32.dll
exports can be found in the
NI -488.2M Function Reference Manual for Windows NT . The NI-488.2M direct entry
sample programs illustrate how to use direct entry to access
gpib-32.dll
. Use the
LoadLibrary
and
GetProcAddress
functions to load the
gpib-32.dll
and get
pointers to its exported functions. For more information on direct entry, refer to the
Win32 SDK (Software Development Kit) documentation.
In your Win32 application, you first need to load
gpib-32.dll
. The following code
fragment demonstrates how to call the
LoadLibrary
function and check for an error:
Gpib32Lib=LoadLibrary("GPIB-32.DLL");
if (Gpib32Lib == NULL) {
return FALSE;
}
Next, your Win32 application must use
GetProcAddress
. The following code
fragment demonstrates how to retrieve the addresses of the pointers to the status variables
and any functions your application needs:
Pibsta = (int *) GetProcAddress(Gpib32Lib, (LPCSTR)"user_ibsta");
Piberr = (int *) GetProcAddress(Gpib32Lib, (LPCSTR)"user_iberr");
Pibcntl = (long *) GetProcAddress(Gpib32Lib,
(LPCSTR)"user_ibcnt");
Pibdev = (int (__stdcall *)(int, int, int, int, int, int))
GetProcAddress(Gpib32Lib, (LPCSTR)"ibdev");
Pibonl = (int (__stdcall *)(int, int)) GetProcAddress(Gpib32Lib,
(LPCSTR)"ibonl");
If
GetProcAddress
fails, it returns a NULL pointer. The following code fragment
demonstrates how to verify that none of the calls to
GetProcAddress
failed:
if ((Pibsta == NULL) ||
(Piberr == NULL) ||
(Pibcntl == NULL) ||
(Pibdev == NULL) ||
(Pibonl == NULL)) {
// ERROR!