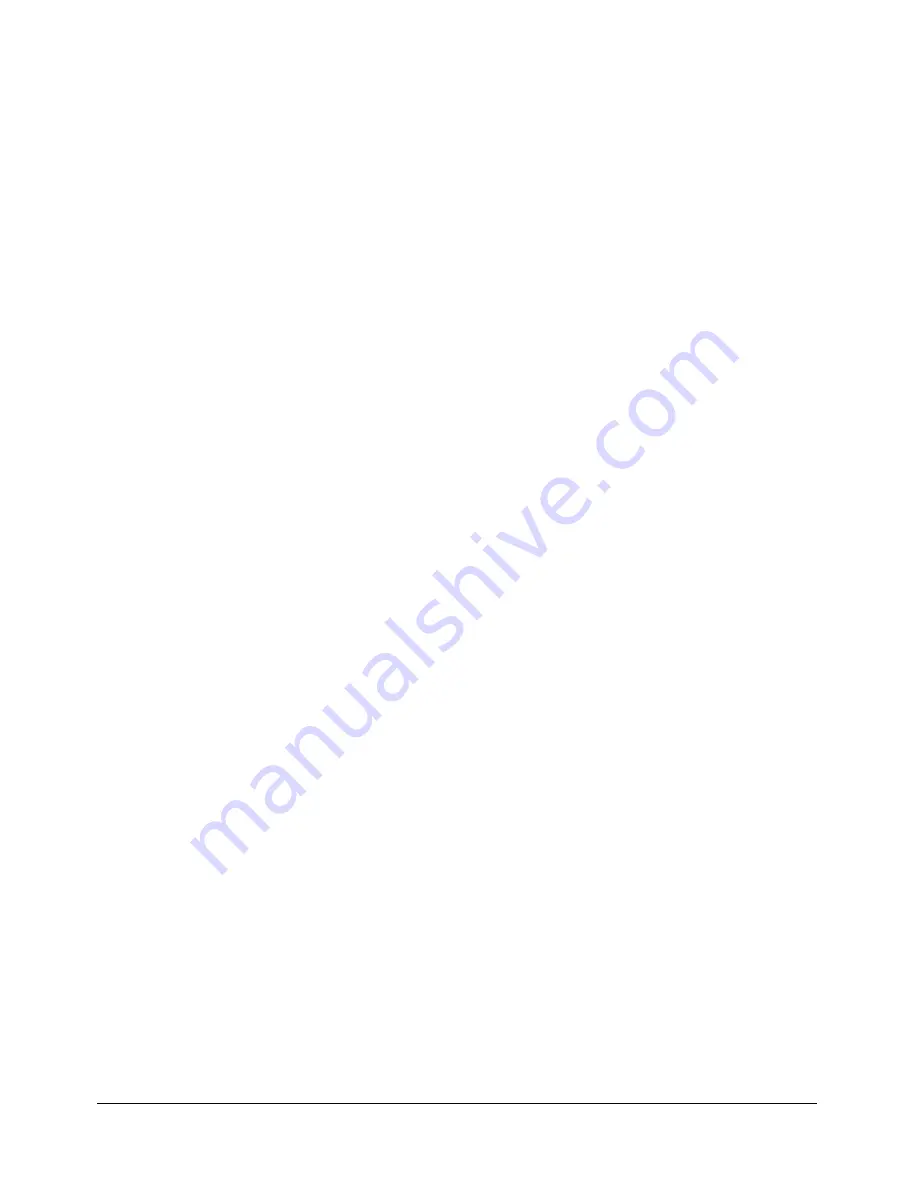
About working with XML
85
For example, the following formatting function takes a record that includes a parkname field. It
converts the parkname text to all lowercase as the label, and uses the field’s length as the data. The
bindFormatFunction()
method calls the formatting function once for each record in the record
set and uses the output of the function to populate a Flash UI component:
function myFormatFunction ( record )
{
// The label is the parkname record field, translated to lowercase
var theLabel:String = record.parkname.toLowerCase();
// The data is the length of the parkname record field
var theData:Number = record.parkname.length;
// Return the label and value to the caller
return {label: theLabel, data: theData};
}
// Call the bindFormatFunction() method
DataGlue.bindFormatFunction(dataView2, result, myFormatFunction);
About working with XML
When you use Flash Remoting you can use either of the following patterns for handling
XML data:
•
You do not use XML in Flash. The Flash application sends information to the server using
simpler data types, including objects if needed. The service functions can generate and
manipulate XML data as necessary. They convert any XML to simpler data types to return to
your Flash application.
•
You use XML directly in Flash. The service functions get XML from, and return XML to, the
Flash application. Your Flash application uses ActionScript XML objects and methods to
generate and manipulate XML as needed.
Your decision as to which method to use should depend on whether it is preferable in your
environment to do more processing in the server or in the client Flash application. For example,
many application servers, such as ColdFusion, provide tools that are specifically optimized for
XML manipulation. Therefore, although Flash includes support for XML objects and provides
methods for manipulating them, you might find it more efficient to process complex XML on
your server and send the processed data to Flash in custom objects, rather than having your
service functions return XML to Flash. However, if you are using Flash Remoting to call a service
that returns XML, you can use the Flash XML methods to access the XML directly.
If you do use XML objects in your Flash application, Flash Remoting converts between the Flash
XML objects used on the client and the standard XML document object type for the application
server: System.Xml.XmlDocument in .NET environments, org.w3c.dom.Document in Java, and
XML document objects in ColdFusion.
The following example sends the contents of two input boxes in an XML object. The first XML
element has the contents of the first text input; this element has a single child that has the
contents of the second text box. The server echoes the XML back to the Flash application, and
the testDocument_Result result handler concatenates the contents of the two nodes to create a
single string for the output box.