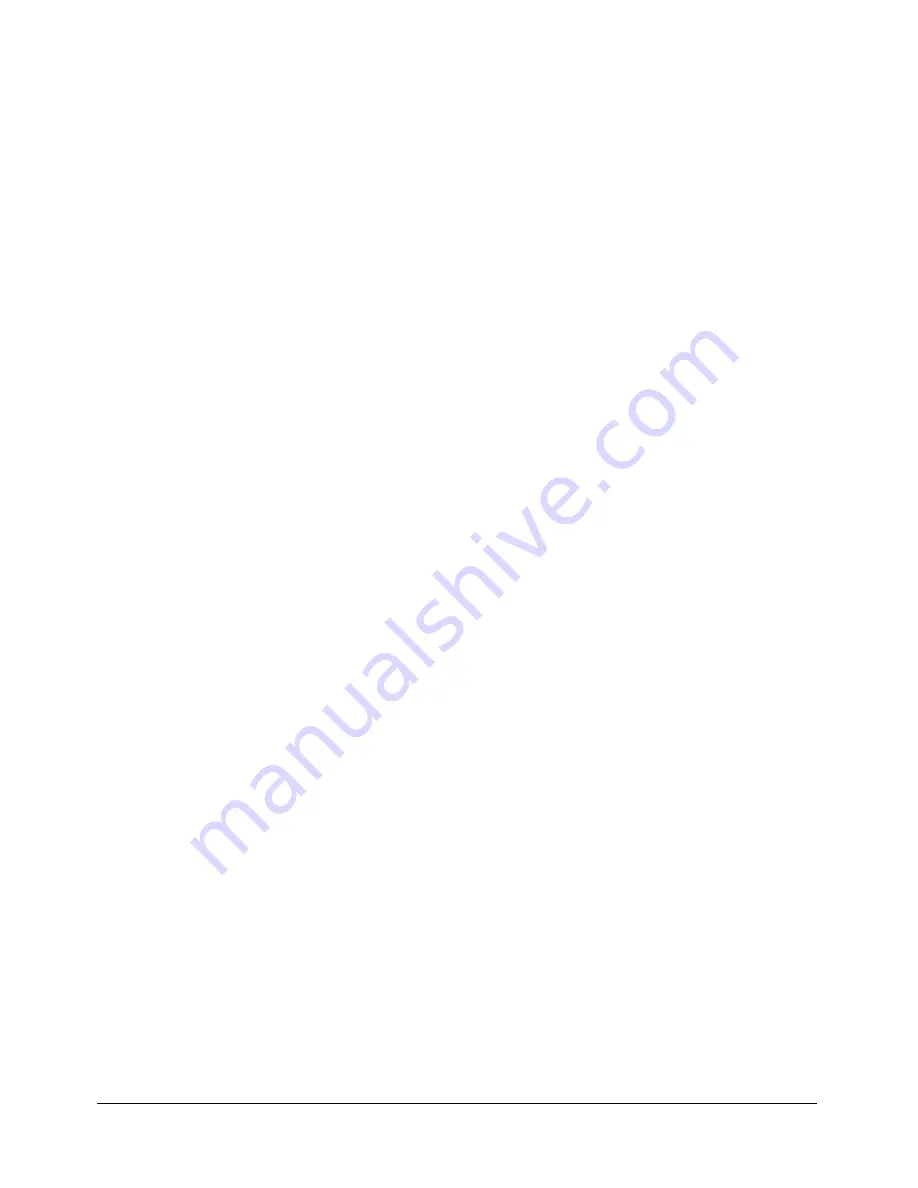
150
Chapter 8: Using Flash Remoting for Microsoft .NET
If more than one parameter is passed from Flash, you access the parameters in your .NET
application in the same order that they were passed from the Flash application. For example, the
following ActionScript function passes two parameters, assuming
firstname
and
lastname
are
input text fields in a Flash application:
ASPXservice.myASPPage(firstname.text, lastname.text);
In an ASPX page, for example, you access the parameters using strict array syntax, as the following
VB.NET code shows:
<%@ Page language="vb" debug="true" CodeBehind="myASPPage.aspx.vb"
AutoEventWireup="false" Inherits="myASPApp.myASPPage" %>
<%@ Register TagPrefix="Macromedia" Namespace="FlashGateway"
Assembly="flashgateway" %>
<Macromedia:Flash ID="Flash" Runat="Server" />
<%
dim message as string
message = "Hi "
if Flash.Params.Count > 0 then
message = message & Flash.Params(0).ToString() & " " &
Flash.Params(1).ToString()
end if
Flash.Result = message
%>
In the code, the
Flash.Params(0)
property represents the
firstname
parameter, and the
Flash.Params(1)
variable represents the
lastname
parameter. The
Page
directive references a
code-behind file, myASPPage.aspx.vb.
Using the Flash Remoting namespace in code-behind files
In ASP.NET applications, you can separate business logic from user interface code using
code-behind files. In the code-behind files, you use the Flash Remoting namespace to access
parameters from and return results to Flash. To use code-behind files, you use the
codebehind
property of the
page
directive in an ASPX page, as the following example shows:
<%@ Page Language="c#" Debug="true" codebehind="myASPPage.aspx.cs"
autoeventwireup="false" Inherits="myASPApp.myASPPage" %>
<%@ Register TagPrefix="Macromedia" Namespace="FlashGateway"
Assembly="flashgateway" %>
<MACROMEDIA:FLASH id="Flash" Runat="Server" />
In the example, the page directive references the code-behind file in the
codebehind
property.
The fully qualified class name is used in the
Inherits
property to inherit the methods of the
code-behind file. You must also use the register directive to register the Flash Remoting custom
server control, and then use the server control in the page.
In the code-behind file itself, you declare the Flash namespace as a protected variable in the class
definition, as the following C# example shows:
namespace myASPApp
{
public class myASPPPage : System.Web.UI.Page
{
protected FlashGateway.Flash Flash;