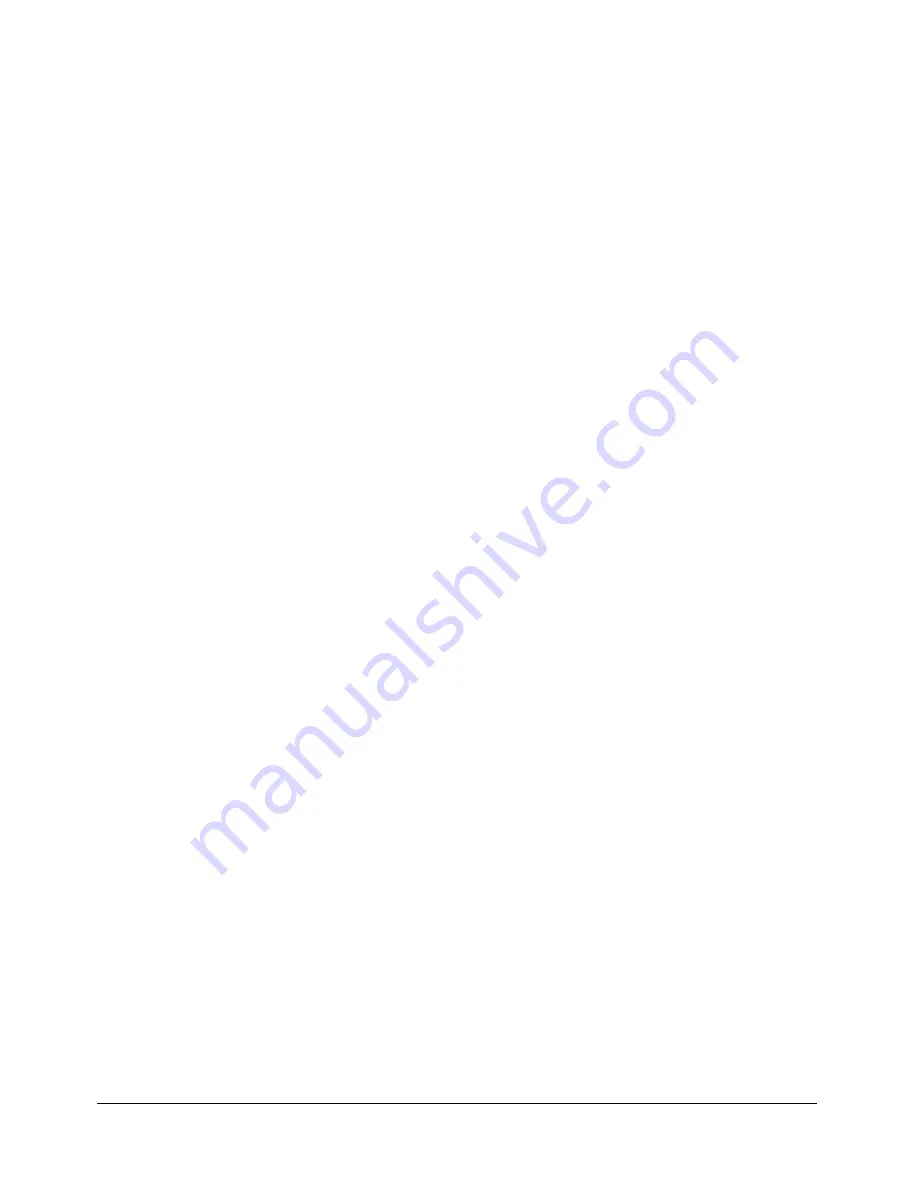
About working with objects
71
When you return an object from the server to Flash, Flash Remoting sends the contents of the
object’s data properties to Flash as a Flash object. In Flash, you can access any of the object’s
properties that are of types that can be converted to Flash data types.
The following sections cover two special cases of objects: ActionScript typed objects and Java
Serializable objects.
Working with ActionScript typed objects
If you use the
Object.RegisterClass
method to register an object in ActionScript, you create a
typed object. Typed objects are useful in Flash applications for creating subclasses of Flash objects.
You can use typed objects in calls to Flash Remoting service functions.
If you use an instance of the object type in a service function call, the
Flashgateway.IO.ASObject
object represents the argument on the server that includes the
object type name.
For example, the following ActionScript creates a typed object and uses it in a service function:
// Make a class (Class constructor)
class myClass extends Object {
myClass() {
super();
value1 = "Test1";
// Register the class definition
Object.registerClass("testClass", myClass);
}
public var value1:String;
}
// Send instance of registered class to a Flash Remoting gateway service
myService.myFunction(new myClass());
When the service function on the application server receives this request, the argument is an
object of type
flashgateway.io.ASObject
in Java and ColdFusion, or
FlashGateway.IO.ASObject
in .NET environments. The service function can access the class
type name, testClass, using the object’s
getType()
method in Java or ColdFusion or the
ASType
property in .NET.
When a service function must create a new typed object to return to Flash Remoting, it creates an
object of type
flashgateway.io.ASObject
in Java or ColdFusion, or of type
FlashGateway.IO.ASObject
in .NET environments. The service function uses the object’s
constructor or
setType()
method in Java,
setType()
method in ColdFusion, or the
ASType
property in .NET to set the class type name to the type specified in the ActionScript
Object.registerClass()
method.
When the Flash client receives the typed object from the service function, Flash runs the
constructor for the type and attaches all the object’s prototype functions.