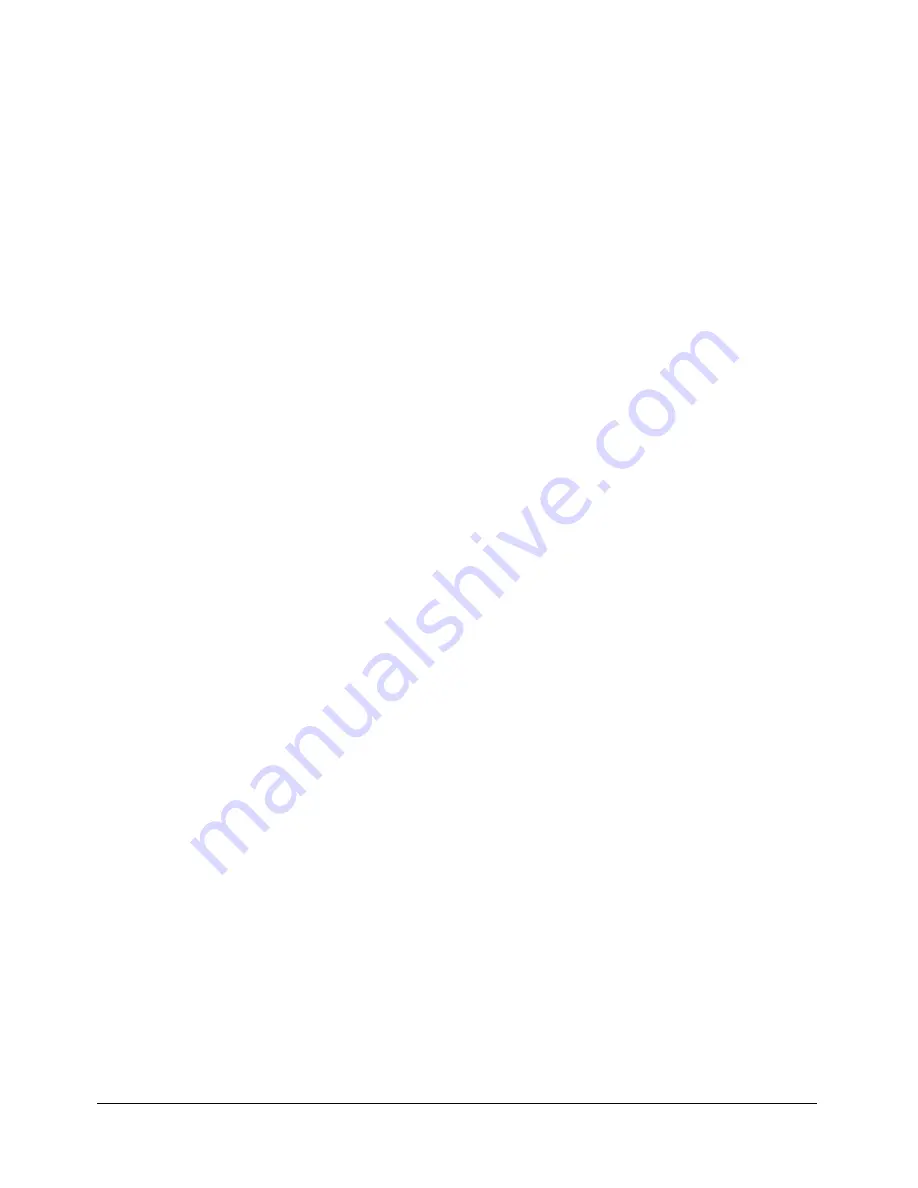
210
Chapter 10: Writing and Calling User-Defined Functions
WriteOutput("MyFunc is not defined");
</cfscript>
You do
not
surround the argument to
IsCustomFunction
in quotation marks, so you can use this
function to determine if function arguments are themselves functions.
Using the Evaluate function
If your user-defined function uses the
Evaluate
function on arguments that contain strings, you
must make sure that all variable names you use as arguments include the scope identifier. Doing
so avoids conflicts with function-only variables.
The following example returns the result of evaluating its argument. It produces the expected
results, the value of the argument, if you pass the argument using its fully scoped name,
Variables.myname. However, the function returns the value of the function local variable if you
pass the argument as myname, without the Variables scope identifier.
<cfscript>
myname = "globalName";
function readname( name ) {
var myname = "localName";
return (Evaluate( name ));
}
</cfscript>
<cfoutput>
<!--- This one collides with local variable name --->
The result of calling readname with myname is:
#readname("myname")# <br>
<!--- This one finds the name passed in --->
The result of calling readname with Variables.myname is:
#readname("Variables.myname")#
</cfoutput>
Passing complex data
Structures, queries, and complex objects such as COM objects are passed to UDFs by reference,
so the function uses the same copy of the data as the caller. Arrays are passed to user-defined
functions by value, so the function gets a new copy of the array data and the array in the calling
page is unchanged by the function. As a result, you must handle arrays differently from all other
complex data types.
Passing structures, queries, and objects
For your function to modify the caller’s copy of a structure, query, or object, you must pass the
variable as an argument. Because the function gets a reference to the caller’s structure, the caller
variable reflects all changes in the function. You do not have to return the structure to the caller.
After the function, returns, the calling page accesses the changed data by using the structure
variable that it passed to the function.
If you do not want a function to modify the caller’s copy of a structure, query, or object, use the
Duplicate
function to make a copy and pass the copy to the function.
Summary of Contents for ColdFusion MX
Page 1: ...Developing ColdFusion MX Applications...
Page 22: ...22 Contents...
Page 38: ......
Page 52: ...52 Chapter 2 Elements of CFML...
Page 162: ......
Page 218: ...218 Chapter 10 Writing and Calling User Defined Functions...
Page 250: ...250 Chapter 11 Building and Using ColdFusion Components...
Page 264: ...264 Chapter 12 Building Custom CFXAPI Tags...
Page 266: ......
Page 314: ...314 Chapter 14 Handling Errors...
Page 344: ...344 Chapter 15 Using Persistent Data and Locking...
Page 349: ...About user security 349...
Page 357: ...Security scenarios 357...
Page 370: ...370 Chapter 16 Securing Applications...
Page 388: ...388 Chapter 17 Developing Globalized Applications...
Page 408: ...408 Chapter 18 Debugging and Troubleshooting Applications...
Page 410: ......
Page 426: ...426 Chapter 19 Introduction to Databases and SQL...
Page 476: ...476 Chapter 22 Using Query of Queries...
Page 534: ...534 Chapter 24 Building a Search Interface...
Page 556: ...556 Chapter 25 Using Verity Search Expressions...
Page 558: ......
Page 582: ...582 Chapter 26 Retrieving and Formatting Data...
Page 668: ......
Page 734: ...734 Chapter 32 Using Web Services...
Page 760: ...760 Chapter 33 Integrating J2EE and Java Elements in CFML Applications...
Page 786: ...786 Chapter 34 Integrating COM and CORBA Objects in CFML Applications...
Page 788: ......