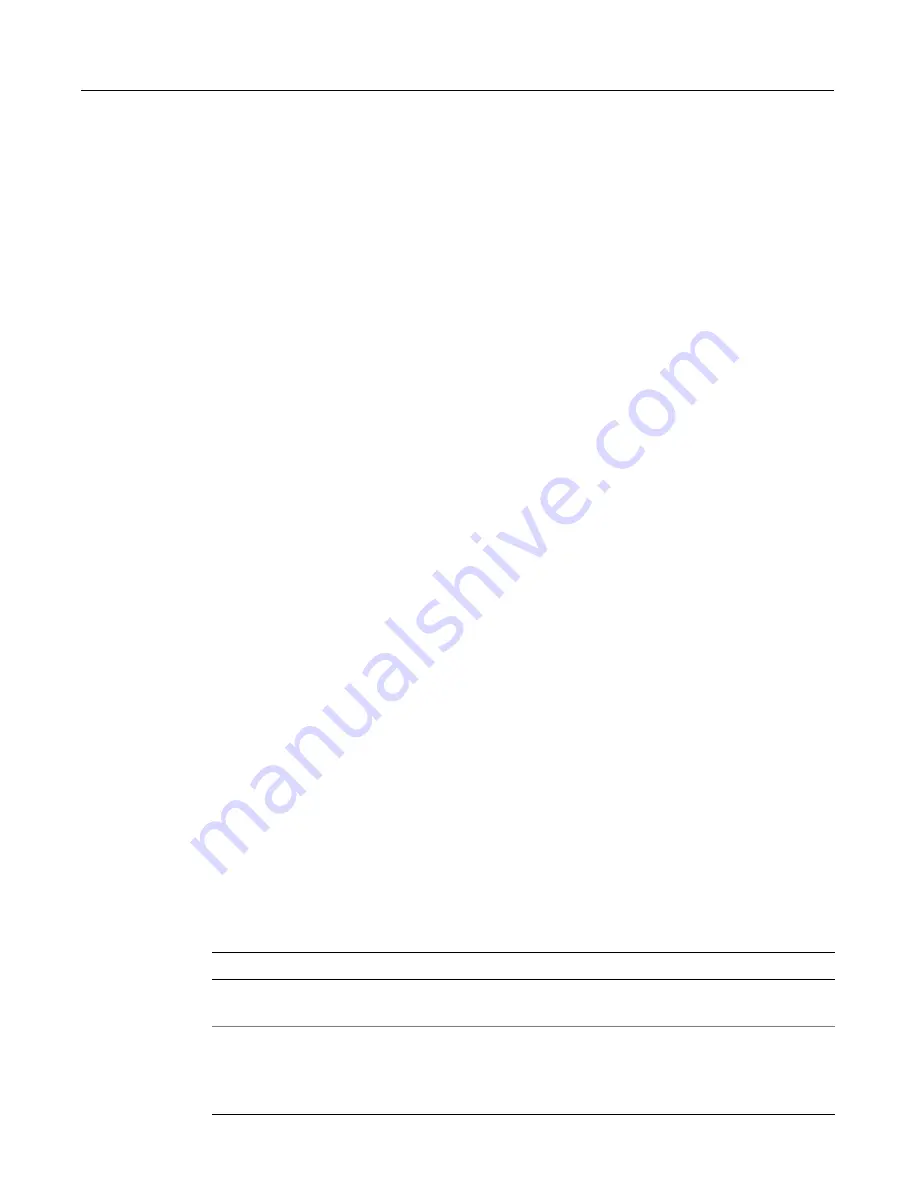
Calling Java Objects
383
Example: exception-throwing class
The following Java code defines the foo class that throws a sample exception. It also
defines a fooException class that extends the Java built-in Exception class and
includes a method for getting an error message.
public class foo {
public foo() {
}
public void doException() throws fooException {
throw new fooException("I am throwing a throw Exception ");
}
}
Class fooException
public class fooException extends Exception {
public String GetErrorMessage() {
return "Error Message from fooException";
}
}
Example: CFML Java exception handling code
The following CFML code calls the foo class doException method. The
cfcatch
block
handles the resulting exception by calling the CFML
GetException
function to
retrieve the Java exception object and then calling the object’s GetErrorMessage
method to get the error information.
<cfobject action=create type=java class=foo name=Obj>
<cftry>
<cfset VOID = Obj.doException() >
<cfcatch type="Any">
<cfset exception=GetException(Obj)>
<cfset message=exception.GetErrorMessage()>
<cfoutput>
<br>The exception message is: #message#<br>
</cfoutput>
</cfcatch>
</cftry>
Reviewing the code
The following table describes the code and its function:
Code
Description
<cfobject action=create type=java
class=foo name=Obj>
Load an instance of the Java foo class
named Obj.
<cftry>
<cfset VOID = Obj.DoException() >
Inside a cftry block. call the doException
method of the Obj object. This method
throws an exception of the fooException
type.
Summary of Contents for COLDFUSION 5-DEVELOPING
Page 1: ...Macromedia Incorporated Developing ColdFusion Applications MacroMedia ColdFusion 5 ...
Page 58: ...38 Chapter 3 Querying a Database ...
Page 134: ...114 Chapter 7 Updating Your Database ...
Page 210: ...190 Chapter 10 Reusing Code ...
Page 232: ...212 Chapter 11 Preventing and Handling Errors ...
Page 238: ...218 Chapter 12 Using the Application Framework ...
Page 262: ...242 Chapter 12 Using the Application Framework ...
Page 278: ...258 Chapter 13 Extending ColdFusion Pages with CFML Scripting ...
Page 320: ...300 Chapter 15 Indexing and Searching Data ...
Page 336: ...316 Chapter 16 Sending and Receiving E mail ...
Page 374: ...354 Chapter 18 Interacting with Remote Servers ...