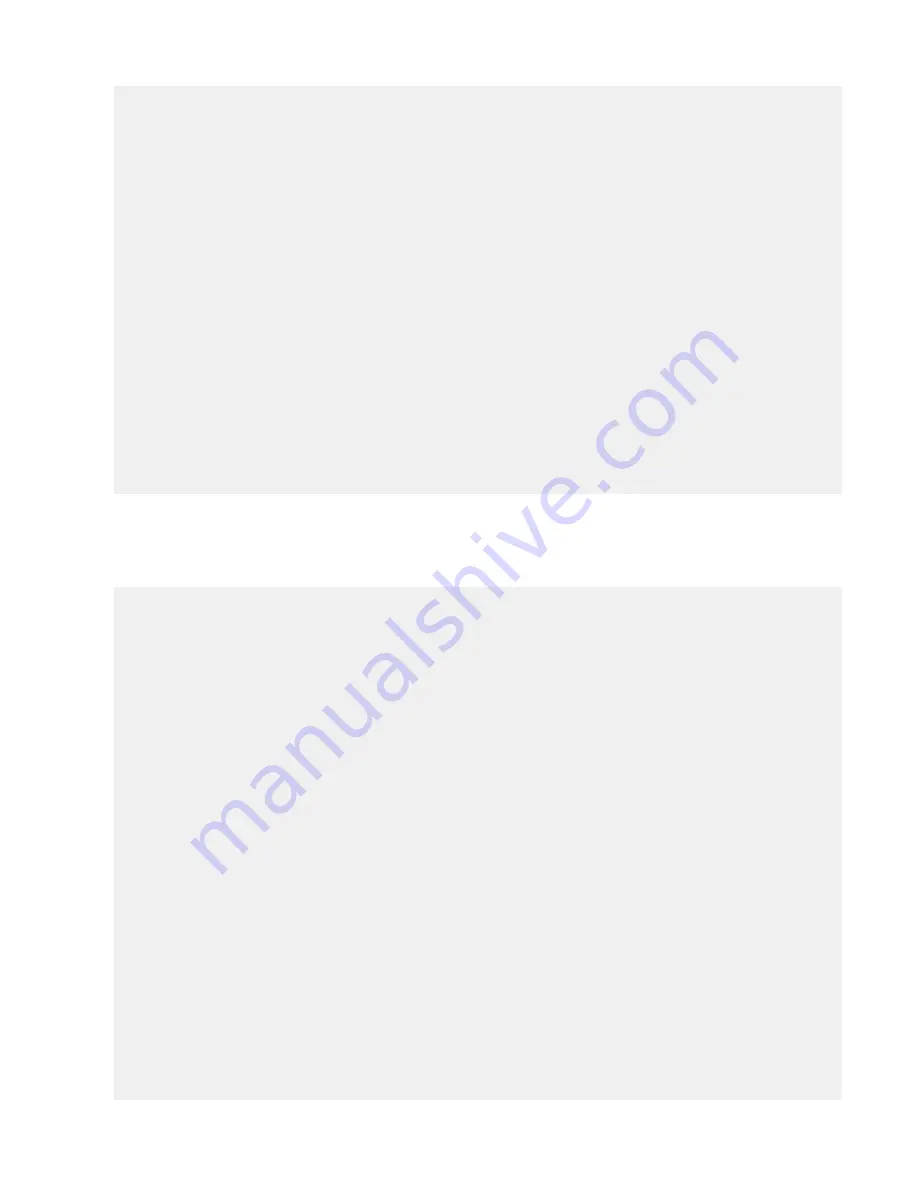
Euresys GenApi scripts
Coaxlink Programmer's Guide
console.log(
'"Coaxlink Quad G3".split(" ") = ['
+
"Coaxlink Quad G3"
.split(
" "
) +
']'
);
// [Coaxlink,Quad,G3]
console.log(
'"Coaxlink Quad G3".split("Quad") = ['
+
"Coaxlink Quad G3"
.split(
"Quad"
) +
']'
);
// [Coaxlink , G3]
console.log(
'["Mono", "Duo", "Quad"].join() = "'
+
[
"Mono"
,
"Duo"
,
"Quad"
].join() +
'"'
);
// "Mono,Duo,Quad"
console.log(
'["Mono", "Duo", "Quad"].join(" & ") = "'
+
[
"Mono"
,
"Duo"
,
"Quad"
].join(
" & "
) +
'"'
);
// "Mono & Duo & Quad"
// Utility functions
console.log(
'random(0,1): '
+ random(0,1));
// random number between 0 and 1
sleep(0.5);
// pause execution of script for 0.5 second
// The builtin function 'require' loads a script, executes it, and returns
// the value of the special 'module.exports' from that module.
var
mod1 = require(
'./module1.js'
);
console.log(
'mod1.description: '
+ mod1.description);
console.log(
'mod1.plus2(3): '
+ mod1.plus2(3));
console.log(
'calling mod1.hello()...'
);
mod1.hello();
// 'require' can deal with:
// - absolute paths
// var mod = require('C:\\absolute\\path\\some-module.js');
// - relative paths (paths relative to the current script)
// var mod = require('./utils/helper.js');
// - coaxlink:// paths (paths relative to the directory where coaxlink scripts
// are installed)
// var mod = require(coaxlink://doc/builtins.js);
doc/grabbers.js
// This file describes the 'grabbers' object of Euresys GenApi Script. It can
// be executed by running 'gentl script coaxlink://doc/grabbers.js'.
// The builtin object 'grabbers' is a list of objects giving access to the
// available GenTL modules/ports.
// In most cases, 'grabbers' contains exactly one element. However, when using
// the 'gentl script' command, 'grabbers' contains the list of all devices.
// This makes it possible to configure several cameras and/or cards.
console.log(
"grabbers.length:"
, grabbers.length);
// Each item in 'grabbers' encapsulates all the ports related to one data
// stream:
// TLPort | GenTL producer
// InterfacePort | Coaxlink card
// DevicePort | local device
// StreamPort | data stream
// RemotePort | camera (if available)
var
PortNames = [
'TLPort'
,
'InterfacePort'
,
'DevicePort'
,
'StreamPort'
,
'RemotePort'
];
// Ports are objects which provide the following textual information:
// name | one of PortNames
// tag | port handle type and value (as shown in memento traces)
for
(
var
i
in
grabbers) {
var
g = grabbers[i];
console.log(
'- grabbers['
+ i +
']'
);
for
(
var
pn of PortNames) {
var
port = g[pn];
console.log(
' - '
+ port.name +
' ('
+ port.tag +
')'
);
}
}
31