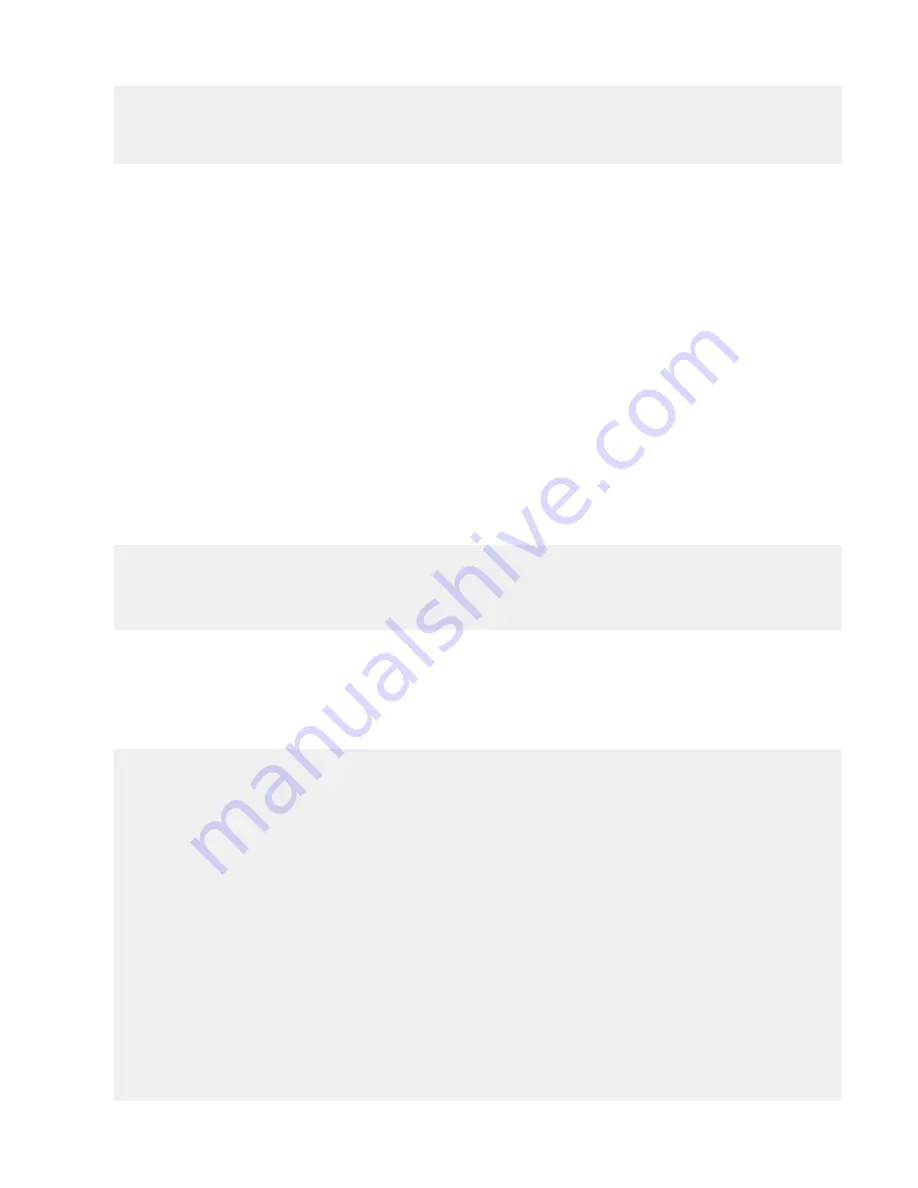
Euresys::EGrabber
Coaxlink Programmer's Guide
showInfo();
}
catch
(
const
std::exception &e) {
// 7
std::cout <<
"error: "
<< e.what() << std::endl;
}
}
1. Include
EGrabber.h
, which defines the
Euresys::EGrabber
class, and includes the other header files we
need (such as
EGenTL.h
and the standard
GenTL header file
).
2. Create a
Euresys::EGenTL
object. This involves the following operations:
• locate and dynamically load the Coaxlink GenTL producer (
coaxlink.cti
);
• retrieve pointers to the functions exported by
coaxlink.cti
, and make them available via
Euresys::EGenTL
methods;
• initialize
coaxlink.cti
(this is done by calling the GenTL initialization function
GCInitLib
).
3. Create a
Euresys::EGrabber
object. The constructor needs the
gentl
object created in step 2. It also takes
as optional arguments the indices of the interface and device to use.
The purpose of the angle brackets (
<>
) that come aer
EGrabber
will become clear later. For now, they can be
safely ignored.
4. Use
GenApi
on page 6 to find out the ID of the Coaxlink card.
Euresys::InterfaceModule
indicates that we
want an answer from the
interface module
.
5. Similarly, find out the ID of the device. This time, we use
Euresys::DeviceModule
to target the
device module
.
6. Finally, read the camera resolution.
Euresys::RemoteModule
indicates that the value must be retrieved from
the camera.
7.
Euresys::EGrabber
uses exceptions to report errors, so we wrap our code inside a
try ... catch
block.
Example of program output:
Interface: PC1633 - Coaxlink Quad G3 (2-camera) - KQG00014
Device: Device0
Resolution: 4096x4096
Acquiring images
This program uses
Euresys::EGrabber
to acquire images from a camera connected to a Coaxlink card:
#include <iostream>
#include <EGrabber.h>
void
grab() {
Euresys::EGenTL gentl;
Euresys::EGrabber<> grabber(gentl);
// 1
grabber.reallocBuffers(3);
// 2
grabber.start(10);
// 3
for
(size_t i = 0; i < 10; ++i) {
Euresys::ScopedBuffer buf(grabber);
// 4
void
*ptr = buf.getInfo<
void
*>(GenTL::BUFFER_INFO_BASE);
// 5
uint64_t ts = buf.getInfo<uint64_t>(GenTL::BUFFER_INFO_TIMESTAMP);
// 6
std::cout <<
"buffer address: "
<< ptr <<
", timestamp: "
<< ts <<
" us"
<< std::endl;
}
// 7
}
int
main() {
try
{
grab();
}
catch
(
const
std::exception &e) {
std::cout <<
"error: "
<< e.what() << std::endl;
}
13