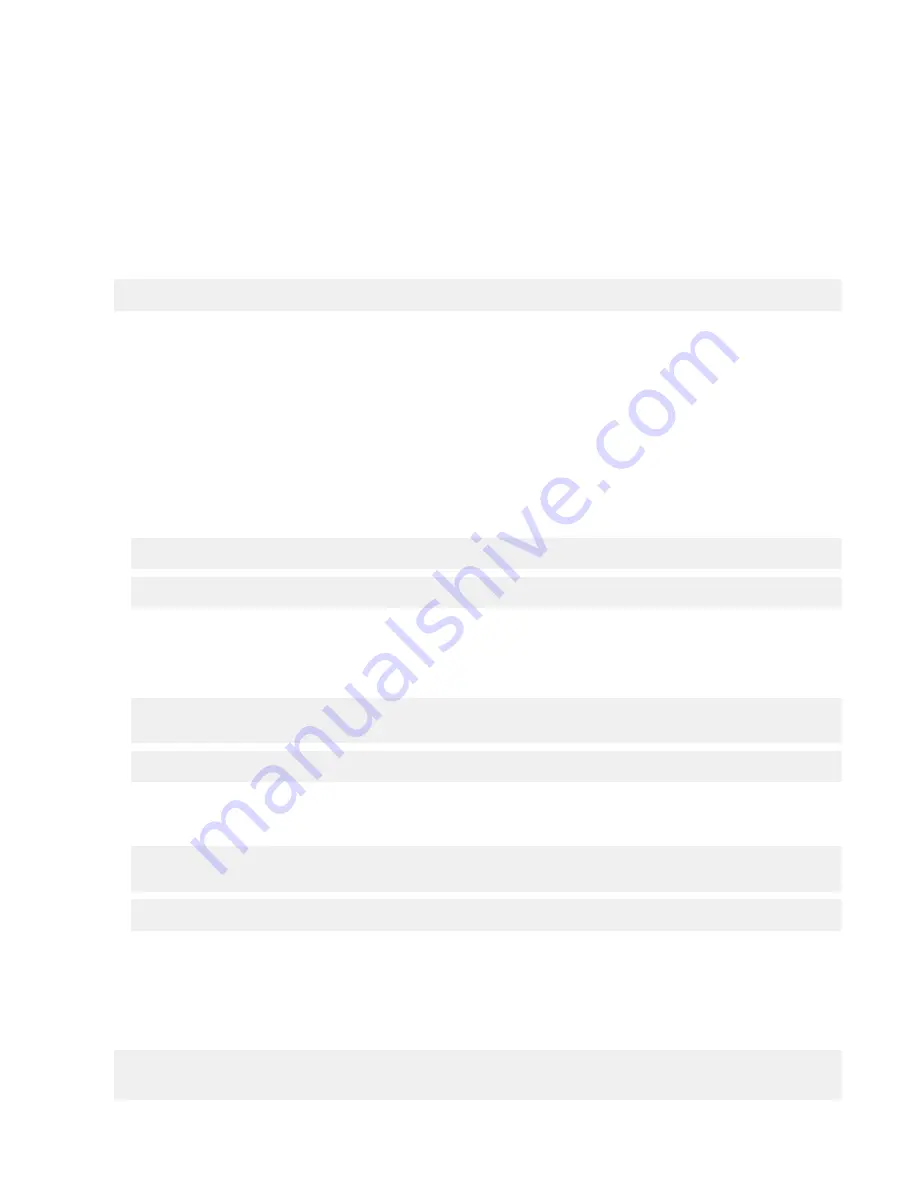
Coaxlink Programmer's Guide
Euresys::EGenTL
Euresys::EGenTL
Euresys::EGenTL
is a library of C++ classes that provide the same functionality as standard GenICam GenTL, but
with a more user-friendly interface. For example, it uses
std::string
instead of raw
char
pointers, and error codes
are transformed into exceptions.
Euresys::EGenTL
also takes care of locating the GenTL producer and loading the
functions it exports.
This library is implemented entirely in C++ header files. As a result, you can simply include the relevant header file:
#include <EGenTL.h>
Instead of the raw, low-level C functions that GenTL defines, we get a
Euresys::EGenTL
object that represents the
GenTL producer:
• Each GenTL function is available as a member method of
Euresys::EGenTL
. GenTL function names start with
an upper-case prefix. In
Euresys::EGenTL
, method names start with the same prefix, but written in lower-case.
For example, the
GCReadPort
function is exposed as the
gcReadPort
method, and the
TLOpenInterface
function as the
tlOpenInterface
method.
• All GenTL functions return a
GC_ERROR
code indicating success or failure. When a function returns a code other
than
GC_ERR_SUCCESS
, an exception is thrown. This removes the burden of manually checking error codes aer
each function call.
• Since GenTL functions return a
GC_ERROR
, output values are returned through pointers passed as arguments.
Euresys::EGenTL
methods offer a more natural interface; they return the output value directly:
GC_API TLGetNumInterfaces(TL_HANDLE hTL, uint32_t *piNumIfaces);
uint32_t tlGetNumInterfaces(TL_HANDLE tlh);
(Note that
GC_API
is defined as
GC_IMPORT_EXPORT GC_ERROR GC_CALLTYPE
. It is simply a
GC_ERROR
decorated with calling convention and DLL import/export attributes.)
• For GenTL functions that deal with text, the corresponding
Euresys::EGenTL
methods convert from
char *
to
std::string
and vice-versa:
GC_API TLGetInterfaceID(TL_HANDLE hTL, uint32_t iIndex,
char
*sID, size_t *piSize);
std::string tlGetInterfaceID(TL_HANDLE tlh, uint32_t index);
• Some GenTL functions retrieve information about the camera or frame grabber. These functions fill a
void *
buffer with a value, and indicate in an
INFO_DATATYPE
the actual type of the value.
Euresys::EGenTL
uses C
++ templates to make these functions easy to use:
GC_API GCGetInfo(TL_INFO_CMD iInfoCmd, INFO_DATATYPE *piType,
void
*pBuffer, size_t *piSize);
template
<
typename
T> T gcGetInfo(TL_INFO_CMD cmd);
A first example
This program uses
Euresys::EGenTL
to iterate over the Coaxlink cards present in the system, and display their id:
#include <iostream>
#include <EGrabber.h> // 1
10