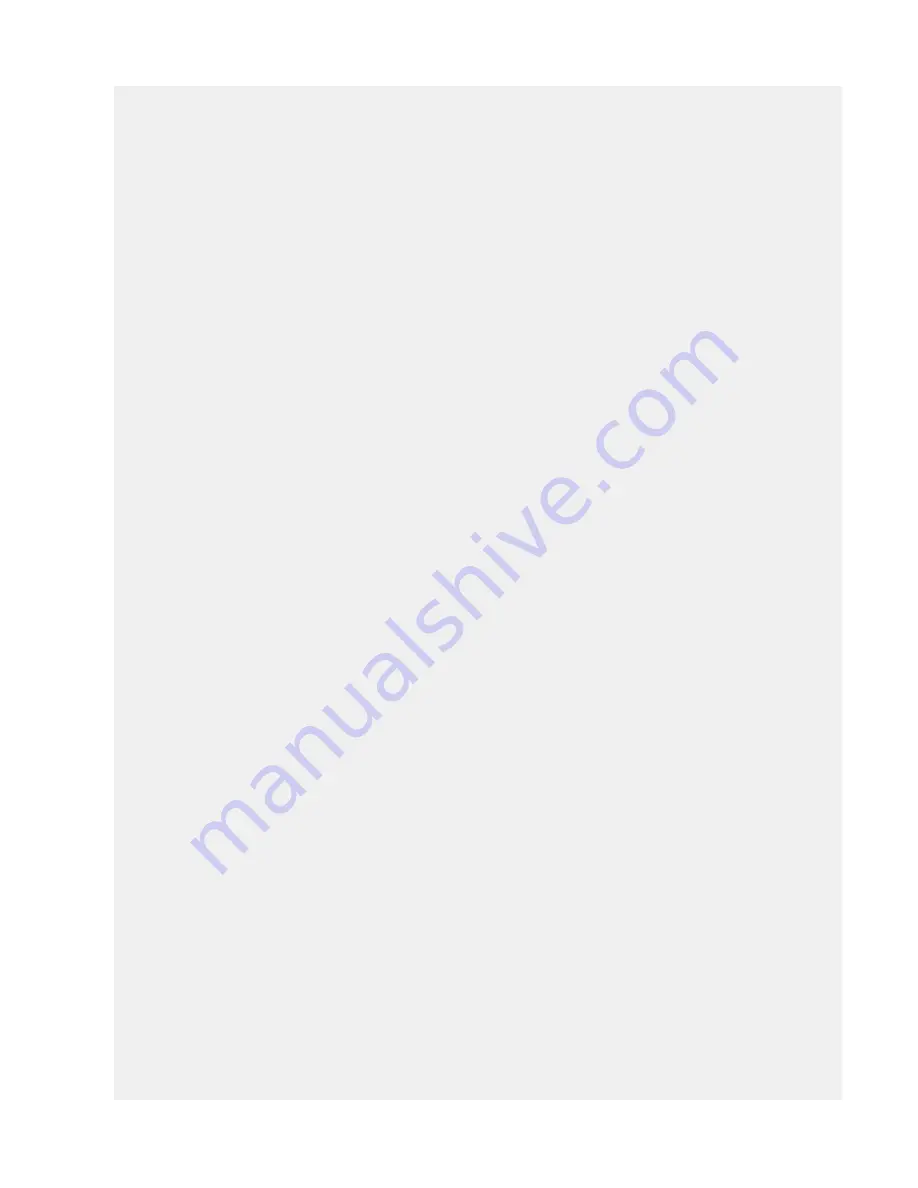
Coaxlink Programmer's Guide
Euresys GenApi scripts
}
function
Undefined() {
// undefined is the type of variables without a value
// undefined is also a special value
var
x;
// undefined
x = 1;
// x has a value
x =
undefined
;
// x is now again undefined
}
// Objects: Object (unordered set of key/value pairs), Array (ordered list
// of values), RegExp (regular expression)
function
Objects() {
// Construction
var
empty = {};
var
x = { a: 1, b: 2, c: 3 };
var
y = { other: x };
// Access to object properties
var
sum1 = x.a + x.b + x.c;
// dot notation
var
sum2 = x[
'a'
] + x[
'b'
] + x[
'c'
];
// bracket notation
// Adding properties
x.d = 4;
// x: { a: 1, b: 2, c: 3, d: 4 }
x[
"e"
] = 5;
// x: { a: 1, b: 2, c: 3, d: 4, e: 5 }
}
function
Arrays() {
// Construction
var
empty = [];
var
x = [3.14159, 2.71828];
var
mix = [1, false,
"abc"
, {}];
// Access to array elements
var
sum = x[0] + x[1];
// bracket notation
// Adding elements
x[2] = 1.61803;
// x: [3.14159, 2.71828, 1.61803]
x[4] = 1.41421;
// x: [3.14159, 2.71828, 1.61803, undefined, 1.41421];
}
function
RegularExpressions() {
var
x = /CXP[36]_X[124]/;
}
}
// Like JavaScript, Euresys GenApi Script is a dynamically typed language. The
// type of a variable is defined by the value it holds, which can change.
function
DynamicVariables() {
var
x = 1;
// Number
x =
"x is now a string"
;
}
// Object types are accessed by reference.
function
References() {
var
x = [3.14159, 2.71828];
// x is a reference to an array
var
y = x;
// y is a reference to the same array
assertEqual(x.length, y.length);
assertEqual(x[0], y[0]);
assertEqual(x[1], y[1]);
y[2] = 1.61803;
// the array can be modified via any reference
assertEqual(x[2], y[2]);
function
update(obj) {
// objects (including arrays) are passed by reference
obj.updated = true;
obj.added = true;
}
var
z = { initialized: true, updated: false };
assertEqual(true, z.initialized);
assertEqual(false, z.updated);
assertEqual(
undefined
, z.added);
update(z);
assertEqual(true, z.initialized);
assertEqual(true, z.updated);
assertEqual(true, z.added);
}
// Supported operators
function
Operators() {
// From lowest to highest precedence:
// Assignment operators: = += -= *= /=
26