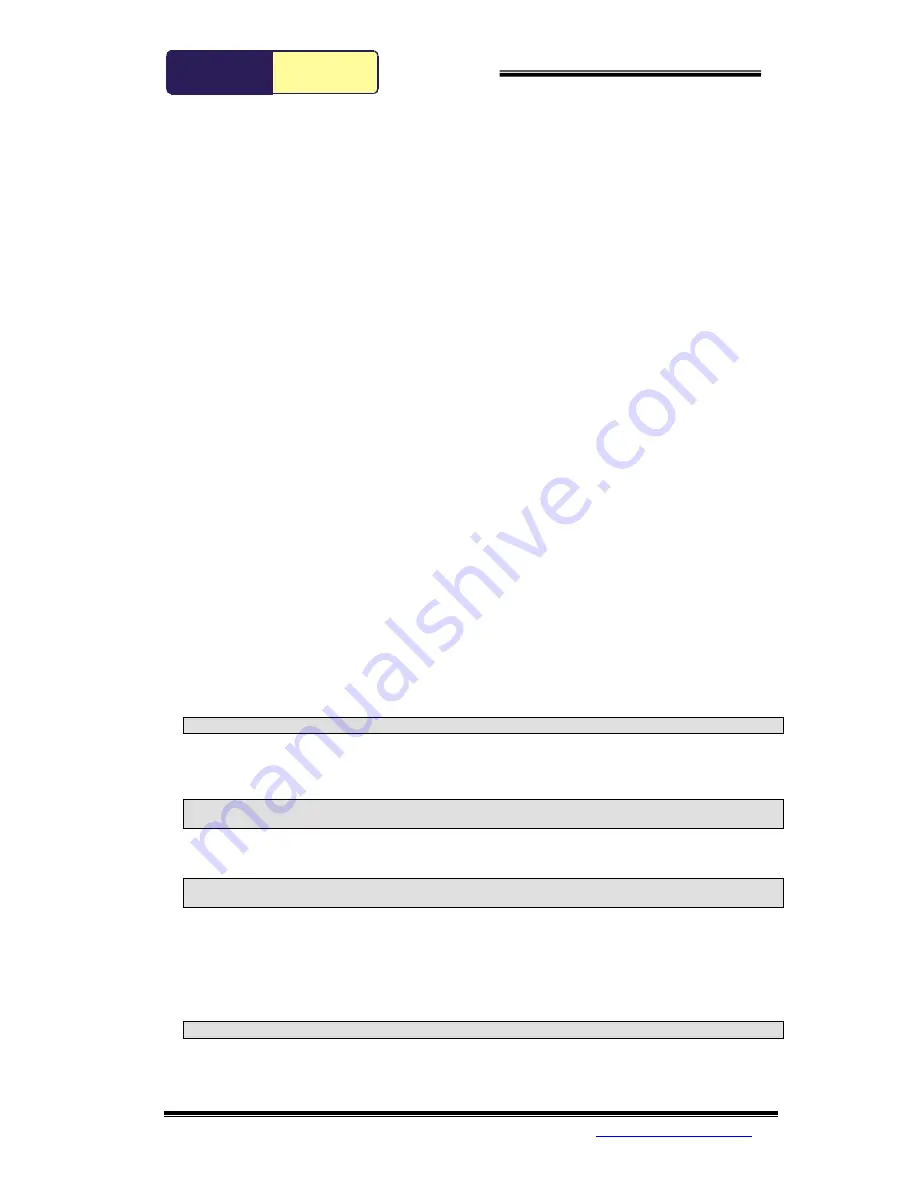
Ether I/O 24 Users Manual V1.3
Elexol Pty Ltd Version 1.3
Page 30
http://www.elexol.com
E L E
X O L
E L E C T R O N I C
S O L U T I O N S
Basic Programming
Please Note: All example code in this section is written in visual basic code using the Winsock control for
opening a UDP socket for communication with the Ether I/O 24 module. As most programming languages
have access to network sockets, please refer to your languages manuals for similar socket functions.
1.
Reading data from the Module
There are two different ways to read data from the socket, polling and interrupt. Polling is best for
simple programs as each part of the program must complete before the next part can begin. However,
if you have set up the AutoScan feature then the interrupt method should be used.
In order to receive data from the module we must bind the port and then check the
Winsock1.BytesReceived value for incoming data. When using the polled mode it is recommended
that each data-waiting loop has a timeout as otherwise a missed packet or unplugged module will cause
your program to stop responding. This is best achieved by using the Timer to exit the data-waiting
loop after a timeout period has elapsed.
The interrupt method is recommended for more advanced programmers as it allows a function to
complete and the program to return to idle while waiting for the data to arrive thereby saving CPU
power, as the program will not have to continually poll the socket for incoming data. This method is
accomplished by using the Winsock1_DataArrival event.
In the following examples we will show both methods where applicable, or just the simple method if
there is no reason to use the interrupt method.
2.
Working with individual bits
As each I/O line is one of a group of 8, we must know how to change these lines one at a time. To
work with these ports as bits we must use the logical OR and logical AND instructions. To make a line
high we take the port’s current value and OR the bit value of the line we wish to change. To make it
low we AND the inverse of the bit value with the port. To generate the inverse value of the bit we use
the NOT function. It is recommended to make a global variable for each of the port values as such.
Global PortAValue, PortBValue, PortCValue As Byte
Thus if we wish to make high the I/O 4 line of port A, we must take the current value of port A and OR
it with 8, which is bit value of I/O 4. The code for this is as follows.
PortAValue = PortAValue Or 8
Winsock1.SendData "A" + Chr$(PortAValue)
To make the same I/O line low again we would use AND with NOT to set the line low.
PortAValue = PortAValue And Not 8
Winsock1.SendData "A" + Chr$(PortAValue)
If the value of the port is not known and you don’t want to change the whole port you must read the
ports value before writing it again.
To convert a line number 1-8 to a bit value 1 – 128 we use twos exponent with the exponent number
being one less than the line number.
BitValue = 2 ^ (Line – 1)